How to Count Characters in a String in Java
-
Count Characters in a String in Java Using the
String.length()
Method -
Count Characters in a String in Java Using Java 8 Streams
chars()
Method -
Count Characters in a String in Java Using the
codePoints()
Method -
Count Characters in a String in Java Using a Loop and
charAt()
-
Count Characters in a String in Java Using
StringUtils
From Apache Commons Lang Library - Count Characters in a String in Java Using Guava Library
- Conclusion
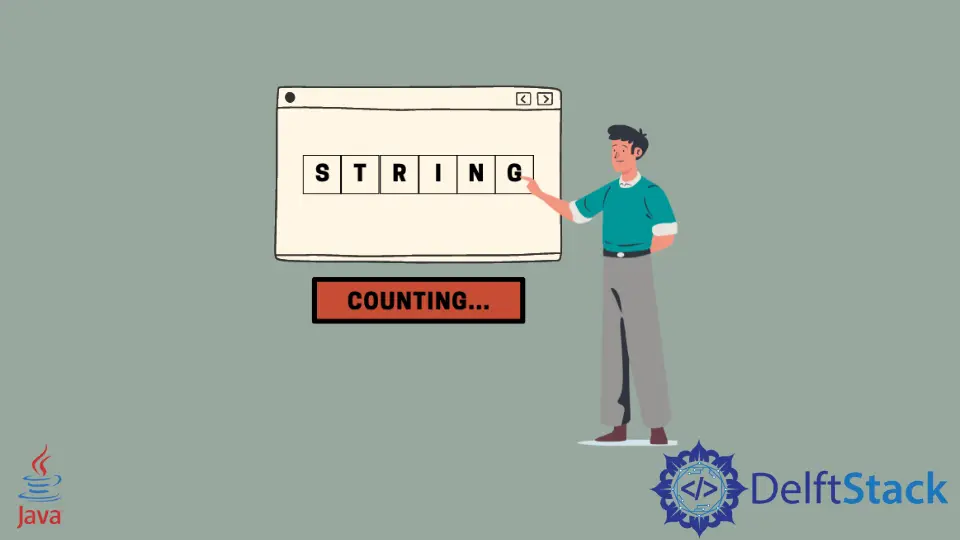
String manipulation is a fundamental aspect of Java programming, and understanding how to work with strings efficiently is crucial for developers. Counting the number of string characters is a common operation, whether you’re validating input lengths, analyzing text data, or performing various string-related tasks.
In this article, we will delve into the different methods and techniques employed to determine the count of characters in a Java string.
Count Characters in a String in Java Using the String.length()
Method
One simple and commonly used method to count all the characters within a given string is by employing the length()
method. This method belongs to the String
class and returns the number of characters in the string.
Syntax of the length()
method:
int length = str.length();
Here, str
is the string for which you want to count the characters, and the result is stored in the variable length
.
Java Count Characters in a String Using String.length()
Example
Code Input:
public class CharacterCountExample {
public static void main(String[] args) {
String str = "Hello, World!";
int length = str.length();
System.out.println("Length of the string: " + length);
}
}
In the above example, an input string Hello, World!
is assigned to the variable str
. The length()
method is then applied to the string, and the result is stored in the variable length
.
The str.length()
call returns the number of characters in the string str
. This method is efficient and convenient for obtaining the length of a string without the need for loops or additional processing.
Code Output:
In this case, the output reflects the total number of characters present in the string Hello, World!
which is 13
.
Count Characters in a String in Java Using Java 8 Streams chars()
Method
Java 8 introduced the Streams API, providing a more functional approach to processing collections, including strings. When it comes to counting characters in a string, the chars()
method is a valuable addition.
This method, part of the String
class, returns an IntStream of the characters in the string, allowing for concise and expressive code.
Syntax of the chars()
method:
long count = str.chars().count();
Here, str
is the string for which you want to count the characters, and the result is stored in the variable count
. The count()
method is then called on the resulting IntStream to obtain the total count.
Java Count Characters in a String Using chars()
Example
Code Input:
import java.util.stream.IntStream;
public class CharacterCountExample {
public static void main(String[] args) {
String str = "Hello, World!";
long count = str.chars().count();
System.out.println("Length of the string: " + count);
}
}
In this Java program, the same given input string is assigned to the variable str
. The chars()
method is then applied to the string, returning an IntStream
of the characters’ Unicode code points.
The count()
method is subsequently called on this stream, obtaining the total count of characters in the string.
Code Output:
This output corresponds to the total number of characters in the string Hello, World!
—13 characters.
Count Characters in a String in Java Using the codePoints()
Method
Java provides various methods to count characters in a string, and the codePoints()
method is another powerful option. This method returns an IntStream
of Unicode code points from the given string, allowing for a flexible and comprehensive approach to character counting.
Syntax of the codePoints()
method:
long count = str.codePoints().count();
Here, str
is the string for which we want to count the characters, and the result is stored in the variable count
. The count()
method is then called on the resulting IntStream
to obtain the total count.
Java Count Characters in a String Using codePoints()
Example
Code Input:
public class CharacterCountExample {
public static void main(String[] args) {
String str = "Hello, DelftStack!";
long count = str.codePoints().count();
System.out.println("Length of the string: " + count);
}
}
In this example, the string Hello, DelftStack!
is assigned to the variable str
. The codePoints()
method is then applied to the string, returning an IntStream
of Unicode code points.
The count()
method is then called on this stream, obtaining the total count of characters in the string.
Code Output:
This output corresponds to the total number of characters present in the string Hello, DelftStack!
—18 characters. The codePoints()
method is particularly useful when dealing with Unicode characters and extended character sets.
Count Characters in a String in Java Using a Loop and charAt()
In some cases, it may be necessary to determine the occurrences of a specific character within a given string. One traditional and effective approach to achieve this is by utilizing a loop in conjunction with the charAt()
method.
The charAt()
method, belonging to the String
class, allows us to access individual characters at specified positions within the string.
The syntax involves iterating through each character in the string using a loop and checking each character using charAt()
:
int count = 0;
for (int i = 0; i < str.length(); i++) {
if (str.charAt(i) == targetChar) {
count++;
}
}
Here, str
is the string in which we want to count the occurrences of a specific character (targetChar
), and the result is stored in the variable count
.
Java Count Characters in a String Using a Loop and charAt()
Example
Code Input:
public class SpecificCharacterCountExample {
public static void main(String[] args) {
String str = "Hello, World!";
char targetChar = 'l';
int count = 0; // Initialize count array index
for (int i = 0; i < str.length(); i++) { // If any matches found
if (str.charAt(i) == targetChar) {
count++;
}
}
System.out.println("Occurrences of '" + targetChar + "': " + count);
}
}
In this example, we specify the target character we want to count, in this case, the character l
. The program then iterates through each character in the string using a loop.
The charAt(i)
method is used to retrieve the character at the current position (i
), and we check if it matches the target character. If there’s a match, the count
variable is incremented.
Code Output:
In this Java program, the output indicates that the character l
appears three times in the string Hello, World!
.
Count Characters in a String in Java Using StringUtils
From Apache Commons Lang Library
For a more convenient and feature-rich approach to counting characters in a string, the Apache Commons Lang library provides the StringUtils
class, which offers a variety of string manipulation utilities. One of these utilities is the StringUtils.countMatches()
method, which allows us to count the occurrences of a specific character or substring within a given string.
Syntax of the StringUtils.countMatches()
method:
int count = StringUtils.countMatches(str, target);
Here, str
is the string for which we want to count the characters, and target
is the specific character or substring we want to count.
When using the StringUtils
class from Apache Commons Lang, it’s essential to include the Apache Commons Lang library as a dependency in your project. If you are using Maven as your build tool, you can add the following dependency to your project’s pom.xml
file:
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.13.0</version> <!-- Replace with the latest version -->
</dependency>
</dependencies>
Make sure to check the Maven Central Repository for the latest version of Apache Commons Lang and update the version number accordingly.
Java Count Characters in a String Using StringUtils
Example
Code Input:
import org.apache.commons.lang3.StringUtils;
public class CharacterCountExample {
public static void main(String[] args) {
String str = "Hello, DelftStack!";
String target = "l";
int count = StringUtils.countMatches(str, target);
System.out.println("Occurrences of '" + target + "': " + count);
}
}
In the above example, the target character to count is also set to l
. The StringUtils.countMatches()
method is then called with the string and target character as arguments. The result is the count of occurrences of the target character in the string.
The use of StringUtils.countMatches()
simplifies the code significantly, providing a concise way to count occurrences of a specific character or substring without the need for explicit loops or manual character comparisons.
Code Output:
In this example, the output indicates that the character l
appears three times in the string Hello, DelftStack!
.
Count Characters in a String in Java Using Guava Library
Guava, a popular open-source Java library developed by Google, provides a set of utility classes and methods to simplify common programming tasks. One such utility is the countIn()
method of the CharMatcher
class, which can be used to count occurrences of a specific character or a set of characters within a string.
Syntax of the CharMatcher.countIn()
method:
int count = CharMatcher.is(targetChar).countIn(str);
Here, str
is the string for which we want to count the characters, and targetChar
is the specific character we want to count.
If you are using Maven as your build tool, add the following dependency to your project’s pom.xml
file:
<dependencies>
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version> <!-- Replace with the latest version -->
</dependency>
</dependencies>
Java Count Characters in a String Using Guava Library Example
Code Input:
import com.google.common.base.CharMatcher;
public class CharacterCountExample {
public static void main(String[] args) {
String str = "Hello, World!";
char targetChar = 'l';
int count = CharMatcher.is(targetChar).countIn(str);
System.out.println("Occurrences of '" + targetChar + "': " + count);
}
}
In this example, we use the same target character to count. The CharMatcher.is(targetChar)
creates a matcher that matches only the specified character.
The countIn(str)
method is then called on this matcher, counting the occurrences of the target character in the string.
Code Output:
In this example, the output indicates that the character l
appears three times in the given string.
Conclusion
In this article, we covered several approaches that cater to different preferences and scenarios: using loops and the charAt()
method, Java 8 Streams, Apache Commons Lang, and Guava. These are the diverse set of tools to choose from based on your project requirements and coding style.
As you progress in your Java journey, consider exploring more advanced topics related to string manipulation, such as pattern matching and regular expressions.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Convert Java String Into Byte
- How to Generate Random String in Java
- The Swap Method in Java