How to Check if String Matches Regex in Python
- Understanding Regular Expressions
- Using re.match() to Check String Matches
- Using re.search() for Pattern Matching
- Using re.fullmatch() for Complete Matches
- Conclusion
- FAQ
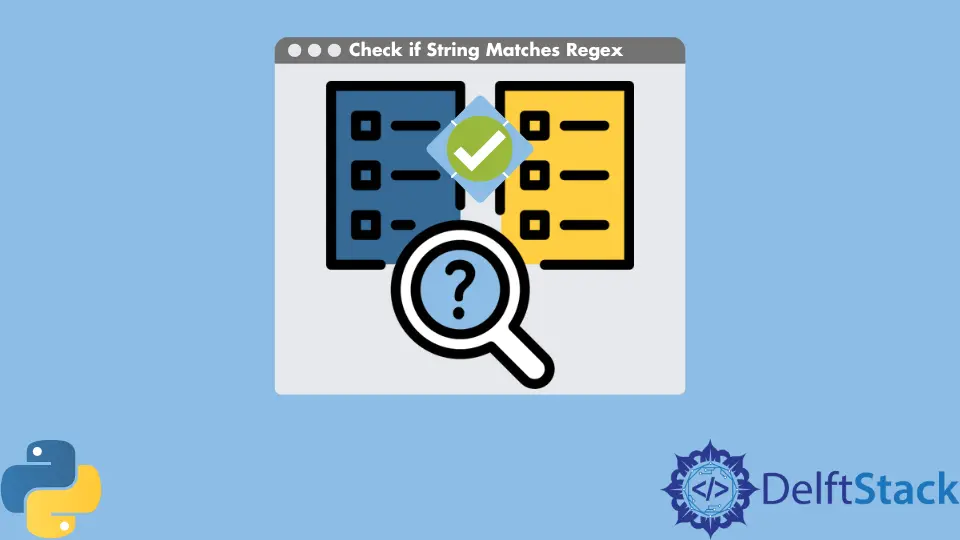
When working with data in Python, you often need to validate or manipulate strings. One powerful tool for this task is regular expressions (regex), which allow you to define complex search patterns.
In this tutorial, we’ll explore how to check if a string matches a regex pattern in Python. Whether you’re validating user input, searching through text, or performing data cleaning, understanding regex can significantly enhance your string manipulation skills. We will cover various methods for checking string matches, complete with clear examples and explanations. By the end of this guide, you’ll be equipped with the knowledge to effectively use regex in your Python projects.
Understanding Regular Expressions
Before diving into the methods for checking if a string matches a regex in Python, it’s essential to grasp what regular expressions are. Regular expressions are sequences of characters that form a search pattern. They can be used for string searching and manipulation, allowing you to find specific patterns within text. Python provides a built-in module called re
that enables you to work with regex easily.
The re
module includes several functions for matching strings against regex patterns. The most common functions are match()
, search()
, and fullmatch()
. Each of these functions serves a unique purpose in string validation. Let’s explore how to use these functions effectively.
Using re.match() to Check String Matches
The re.match()
function checks if the beginning of a string matches a specified regex pattern. If the pattern is found at the start of the string, it returns a match object; otherwise, it returns None
. This method is useful when you want to ensure that a string starts with a specific pattern.
Here’s a simple example:
import re
pattern = r'^[A-Z]' # Pattern to check if the string starts with an uppercase letter
string = "Hello, World!"
match = re.match(pattern, string)
if match:
result = "Match found"
else:
result = "No match"
Output:
Match found
In this example, we define a regex pattern that checks if the string starts with an uppercase letter. The re.match()
function evaluates the string “Hello, World!” against this pattern. Since the string begins with “H,” which is uppercase, the function returns a match object, indicating a successful match.
Using re.search() for Pattern Matching
While re.match()
only checks for a match at the start of the string, re.search()
scans through the entire string for a match. This function is particularly useful when you want to find a pattern anywhere in the string, not just at the beginning.
Let’s take a look at how to use re.search()
:
import re
pattern = r'\d+' # Pattern to find one or more digits
string = "There are 42 apples."
search = re.search(pattern, string)
if search:
result = "Match found"
else:
result = "No match"
Output:
Match found
In this code, we use the regex pattern \d+
, which looks for one or more digits in the string. The re.search()
function scans through “There are 42 apples.” and successfully finds “42.” This demonstrates how re.search()
can locate a pattern anywhere within a string, making it a versatile option for string validation.
Using re.fullmatch() for Complete Matches
If you want to check whether an entire string matches a regex pattern, re.fullmatch()
is the function to use. This method ensures that the entire string conforms to the specified pattern, which is particularly useful for validating formats like email addresses or phone numbers.
Here’s an example of how to use re.fullmatch()
:
import re
pattern = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$' # Pattern for email validation
string = "example@example.com"
full_match = re.fullmatch(pattern, string)
if full_match:
result = "Valid email"
else:
result = "Invalid email"
Output:
Valid email
In this example, we define a regex pattern that matches a valid email format. The re.fullmatch()
function checks if the entire string “example@example.com” fits this pattern. Since it does, the function returns a match object, confirming that the email is valid. This method is particularly valuable for scenarios where strict adherence to a format is necessary.
Conclusion
Regular expressions are a powerful tool in Python for string validation and manipulation. By using functions like re.match()
, re.search()
, and re.fullmatch()
, you can effectively check if a string matches a desired pattern. Whether you’re validating user input, searching through text, or cleaning data, mastering regex will significantly enhance your programming skills. With the examples provided in this tutorial, you now have a solid foundation to start using regex in your Python projects.
FAQ
-
What is a regular expression?
A regular expression is a sequence of characters that defines a search pattern, commonly used for string searching and manipulation. -
How do I import the regex module in Python?
You can import the regex module in Python using the statementimport re
. -
What is the difference between re.match() and re.search()?
re.match()
checks for a match only at the beginning of the string, whilere.search()
scans through the entire string for a match. -
Can I use regex for email validation in Python?
Yes, you can use regex patterns to validate email formats using functions likere.fullmatch()
. -
How do I test if a string contains digits using regex?
You can use the pattern\d+
withre.search()
to check if a string contains one or more digits.
Related Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python