How to Convert a String to Variable Name in Python
-
Convert String to Variable Name Using
globals()
andlocals()
in Python -
Convert String to Variable Name Using
exec()
in Python - Convert String to Variable Name Using Dictionary in Python
- Conclusion
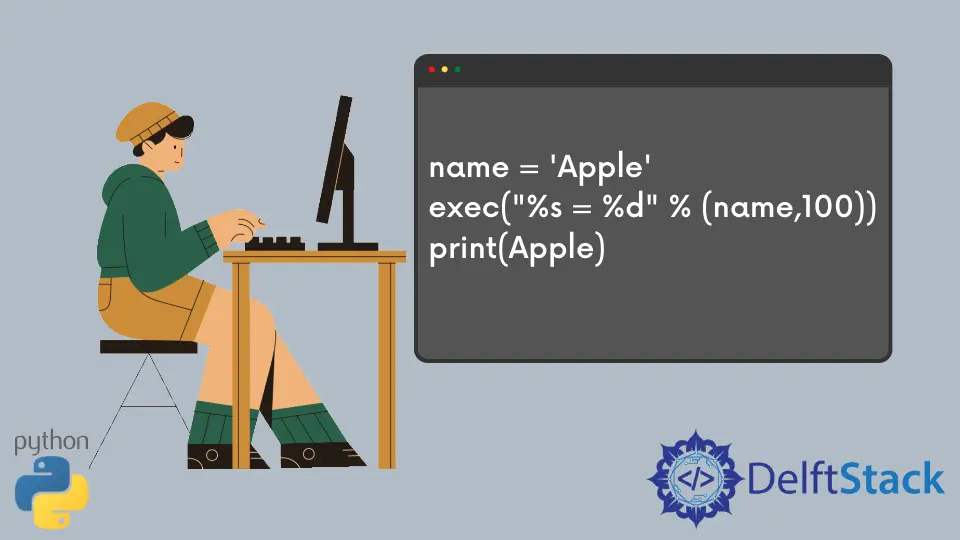
In Python, there are various ways in which we can convert a string value into a variable name. In this article, we will discuss various ways in which we can achieve this.
Some people might want to do this to define a variable name dynamically while the Python program is under execution. Converting a string to a variable name using the ways shown below is not recommended while developing production-ready software because this can cause some issues.
Note: It is possible to convert a string value into a variable, but please be cautious while doing this.
Convert String to Variable Name Using globals()
and locals()
in Python
Using globals()
to Convert String Into a Variable
In Python, dynamic programming can extend to the creation of variable names from strings. This is where the built-in globals()
function becomes particularly useful.
It allows for the dynamic creation and manipulation of variables within the global scope based on string values.
The globals()
function in Python returns the dictionary of the current global symbol table. A global symbol table stores all the information related to the program’s global scope, which can be accessed using the globals()
function.
def create_global_variable(name, value):
globals()[name] = value
# Define variable names and values
variable_name = "dynamicGlobalVar"
variable_value = 10
# Create a global variable with the specified name and value
create_global_variable(variable_name, variable_value)
# Access and print the newly created global variable
print(dynamicGlobalVar)
In our code, we first define a function create_global_variable
that takes two parameters: name
and value
. Inside this function, we access the global symbol table using globals()
and create a new entry where the key is name
and the value is value
.
This operation dynamically creates a global variable.
After defining this function, we specify the name ("dynamicGlobalVar"
) and value (10
) of our new variable. By calling create_global_variable(variable_name, variable_value)
, we dynamically create a global variable named dynamicGlobalVar
.
Lastly, we use a print statement to output the value of this newly created variable.
Output:
Using locals()
to Convert String Into a Variable
The locals()
function in Python returns the dictionary of the current local symbol table. A local symbol table can be accessed with the help of the locals()
function.
The locals()
function almost works the same way as the globals()
function. The only difference is that locals()
can access the local symbol table, and globals()
can access the global symbol table and return a dictionary.
def demonstrate_locals():
locals()["temp_var"] = "Dynamic Content"
return locals().get("temp_var")
# Call the function and print the result
print(demonstrate_locals())
In our example, the function demonstrate_locals
is designed to showcase the use of locals()
. We use locals()
to access the local symbol table and add a new entry with the key 'temp_var'
and the value 'Dynamic Content'
.
This line effectively creates a new local variable named temp_var
.
After creating this variable, we use locals().get('temp_var')
to retrieve the value of temp_var
. This is a safe way to access the variable, especially considering that direct modification of the local symbol table can be unpredictable in some scenarios.
Finally, we call demonstrate_locals
and print its return value, which is the value of the newly created temp_var
.
Output:
Convert String to Variable Name Using exec()
in Python
In Python, one of the more advanced and powerful capabilities is the execution of Python code contained in a string using the exec()
function. This can include dynamically creating variables from strings, a technique that, while useful in certain scenarios, requires careful handling to avoid security risks and maintain code clarity.
The exec()
function is used to execute dynamically generated Python code, which can include variable assignments, function definitions, or even class definitions. Its primary use is in scenarios where the code to be executed is not known until runtime.
# Variable name and value as strings
variable_name = "dynamicVar"
value = 42
# Constructing and executing the string as Python code
exec(f"{variable_name} = {value}")
# Access and print the newly created variable
print(dynamicVar)
In our code, we start by defining two strings: variable_name
, which is intended to be the name of our new variable, and value
, which represents the value we want to assign to it. We then use an f-string to construct a string that represents a Python assignment statement: dynamicVar = 42
.
The exec()
function is used to execute this string as Python code. This effectively creates a variable named dynamicVar
with the value 42
in the current namespace.
Finally, we demonstrate that this variable has been created and contains the expected value by printing it.
Output:
Convert String to Variable Name Using Dictionary in Python
In Python, managing dynamic variable names necessitates a flexible and secure approach. One effective method is using dictionaries to map strings to variable names.
This technique sidesteps the complexities and risks associated with functions like exec()
and globals()
, offering a safer and more manageable alternative.
Using dictionaries as a substitute for dynamic variables is a common practice in Python, especially in scenarios where the creation and management of a large number of variables are required.
# Creating a dictionary to hold dynamic variables
dynamic_vars = {}
# Defining variable names and values
var_name = "temperature"
var_value = 23
# Assigning the variable name and value to the dictionary
dynamic_vars[var_name] = var_value
# Accessing and printing the value using the variable name
print(dynamic_vars["temperature"])
In this example, we start by creating an empty dictionary named dynamic_vars
. This dictionary will serve as a container for our dynamic variables.
We then define two variables, var_name
and var_value
, which hold the string that represents our variable’s name and its corresponding value, respectively.
Next, we use the string stored in var_name
as a key in our dynamic_vars
dictionary and assign var_value
to this key. This action mimics the creation of a variable named temperature
with the value 23
.
Finally, we access and print the value associated with the key "temperature"
in the dictionary.
Output:
Conclusion
This article explored various methods in Python for converting strings into variable names, highlighting their unique applications and cautionary notes. The globals()
and locals()
functions allow dynamic variable creation in global and local scopes, respectively, offering flexibility but requiring careful use to avoid complexity and risks in production environments.
The exec()
method, capable of executing Python code dynamically, provides considerable flexibility but comes with significant security concerns and can affect code clarity. Finally, the dictionary-based approach presents a safer, structured alternative, avoiding the risks associated with the other methods and maintaining a clean namespace.
Each method has its advantages and trade-offs, with the choice dependent on project requirements and a balance between safety, simplicity, and clarity. This exploration aids programmers in selecting the most suitable method for their specific needs, ensuring efficient and secure code implementation.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedInRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python
- How to Convert String to Datetime in Python