How to Check Variable Type in Python
- Variables and Their Types in Python
- Check the Type of a Variable in Python
-
Use the
type()
Function to Check Variable Type in Python -
Use the
isinstance()
Function to Check Variable Type in Python
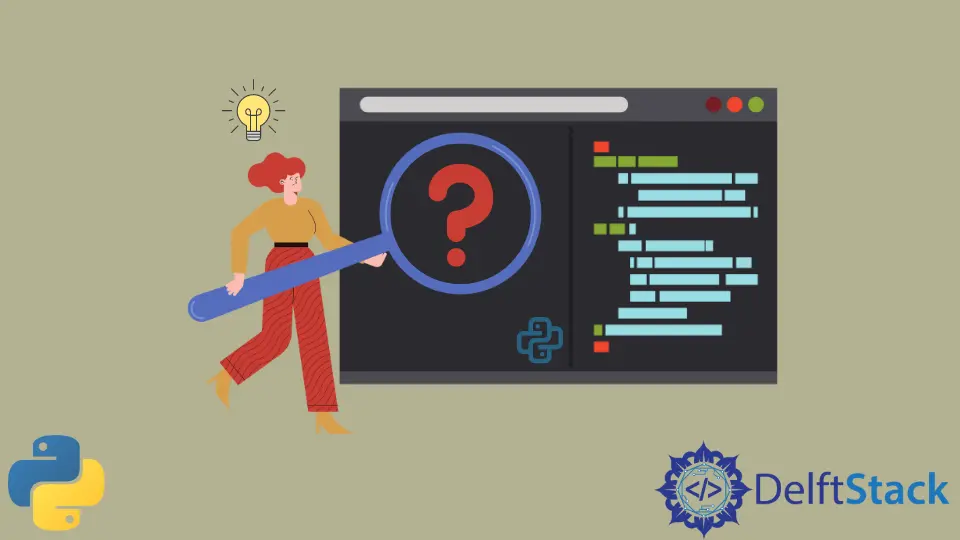
In Python, if you want to store any kind of data, perform some operations on that data or pass the data between functions or objects, you first have to store that data somewhere. It is done with the help of a variable.
Variables and Their Types in Python
A variable is nothing but a box or container inside which our data will be stored. The variable will be allocated to some space in memory (RAM). The data type tells us what type of data a variable holds. And depending on the type of data, the compiler will decide how much space to allocate to that variable inside the memory. And then will assign the memory accordingly.
In Python, you don’t have to explicitly define the type of data you will store inside the variable as you do in C/C++, Java, or any other major programming languages. Here, you can directly assign the value to the variable, and the compiler will identify what type of data the variable holds and in which class it belongs to the integer, string, list, etc.
# Variable of type String
newVariable = "This is a new variable"
print(newVariable)
# Variable of type Boolean
booleanVariable = True
print(booleanVariable)
# Variable of type Float
floatVariable = 20.30
print(floatVariable)
Output:
This is a new variable
True
20.3
Check the Type of a Variable in Python
The variable can be from any of the data types in Python, and all of those are mentioned below.
- Number: This category contains integers, floating-point numbers, and complex numbers.
- String: It is a sequence of Unicode characters. A Unicode is a character set that contains characters and symbols from all languages around the world.
- Boolean: Boolean represents either
True
orFalse
. - List: It is an ordered collection of elements of the different data types. Lists are mutable, which means values inside the list can be changed after it has been created.
- Tuple: It is also an ordered collection of elements of the different data types. The only difference between a list and a tuple is that tuples are immutable, which means that once they are created, they cannot be modified.
- Set: A Set is an unordered collection of unique items.
- Dictionary: It is an unordered collection of key-value pairs. The key and value can be of any type.
There are two ways in which you can check the type of a variable in Python.
Use the type()
Function to Check Variable Type in Python
To check the type of a variable, you can use the type()
function, which takes the variable as an input. Inside this function, you have to pass either the variable name or the value itself. And it will return the variable data type.
myInt = 50
print(type(myInt))
myFloat = 10.50
print(type(myFloat))
myString = "My name is Adam"
print(type(myString))
Output:
<class 'int'>
<class 'float'>
<class 'str'>
Use the isinstance()
Function to Check Variable Type in Python
Another function that can be used to check the type of a variable is called isinstance()
. You need to pass two parameters; the first is the variable (the value whose data type you want to find), and the second parameter is the variable type. If the variable type is identical to the type you have specified in the second parameter, it will return True
and False
otherwise.
# A variable 'myVar' having a value 50 inside
myVar = 50
# Printing the value returned by isinstance() function
print("Does myVar belongs to int: ", isinstance(myVar, int))
# This will return false
# As the value passed is string and you are checking it with int
print("Does string belongs to int: ", isinstance("My String", int))
complexNo = 1 + 2j
print("This this number complex: ", isinstance(complexNo, complex))
Output:
Does myVar belongs to int: True
Does string belongs to int: False
This this number complex: True
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn