在 Python 中檢查變數型別
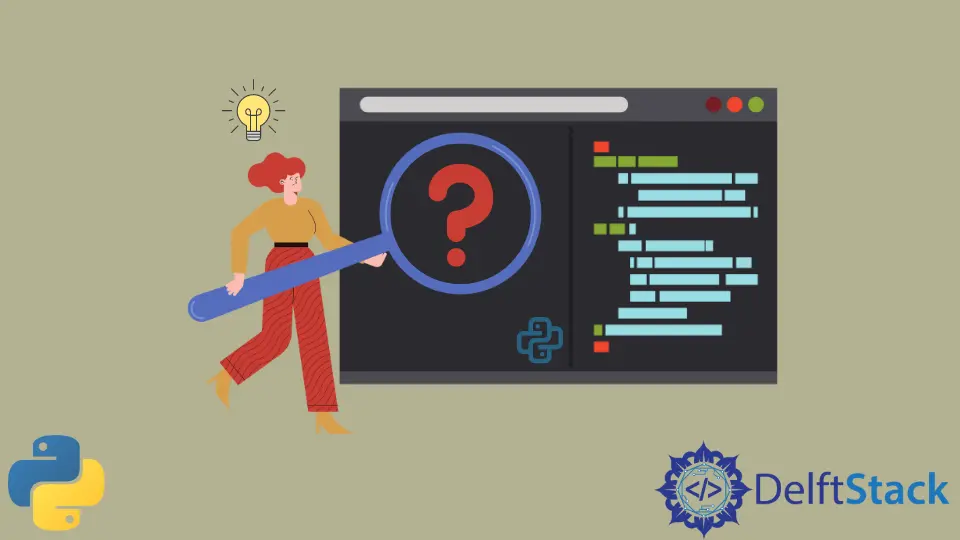
在 Python 中,如果你想儲存任何一種資料,對這些資料進行一些操作,或者在函式或物件之間傳遞資料,你首先要把這些資料儲存在某個地方。這是在變數的幫助下完成的。
Python 中的變數及其型別
變數不過是一個盒子或容器,我們的資料將被儲存在裡面。變數將被分配到記憶體(RAM)的某個空間。資料型別告訴我們一個變數存放的資料型別。而根據資料的型別,編譯器會決定在記憶體裡面給這個變數分配多少空間。然後會相應的分配記憶體。
在 Python 中,你不必像在 C/C++、Java 或其他主要程式語言中那樣,明確定義你將儲存在變數裡面的資料型別。在這裡,你可以直接給變數賦值,編譯器會識別出變數存放的資料是什麼型別,屬於整數、字串、列表等哪個類。
# Variable of type String
newVariable = "This is a new variable"
print(newVariable)
# Variable of type Boolean
booleanVariable = True
print(booleanVariable)
# Variable of type Float
floatVariable = 20.30
print(floatVariable)
輸出:
This is a new variable
True
20.3
在 Python 中檢查變數的型別
變數可以來自 Python 中的任何一種資料型別,下面都會提到。
- 數字:這一類包含整數、浮點數和複數。
- 字串:它是 Unicode 字元的序列。Unicode 是一個字符集,包含了全世界所有語言的字元和符號。
- 布林型:布林型代表
True
或False
。 - 列表:它是不同資料型別元素的有序集合。列表是可以突變的,也就是說在建立了列表之後,列表裡面的值是可以改變的。
- 元組:它也是不同資料型別元素的有序集合。它也是不同資料型別元素的有序集合。列表和元組的唯一區別是,元組是不可變的,也就是說一旦建立了元組,就不能修改。
- 集合:集合是一個無序的不含重複元素的集合。
- 字典:它是鍵值對的無序集合。鍵和值可以是任何型別。
在 Python 中,有兩種方法可以檢查一個變數的型別。
使用 type()
函式來檢查 Python 中的變數型別
要檢查一個變數的型別,你可以使用 type()
函式,它將變數作為輸入。在這個函式中,你必須傳遞變數名或變數值本身,它將返回變數的資料型別。它將返回變數的資料型別。
myInt = 50
print(type(myInt))
myFloat = 10.50
print(type(myFloat))
myString = "My name is Adam"
print(type(myString))
輸出:
<class 'int'>
<class 'float'>
<class 'str'>
在 Python 中使用 isinstance()
函式檢查變數型別
另一個可以用來檢查變數型別的函式是 isinstance()
。你需要傳遞兩個引數,第一個是變數(你想找到其資料型別的值),第二個引數是變數型別。如果變數型別與你在第二個引數中指定的型別相同,它將返回 True
,否則返回 False
。
# A variable 'myVar' having a value 50 inside
myVar = 50
# Printing the value returned by isinstance() function
print("Does myVar belongs to int: ", isinstance(myVar, int))
# This will return false
# As the value passed is string and you are checking it with int
print("Does string belongs to int: ", isinstance("My String", int))
complexNo = 1 + 2j
print("This this number complex: ", isinstance(complexNo, complex))
輸出:
Does myVar belongs to int: True
Does string belongs to int: False
This this number complex: True
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn