在 Python 中定義類全域性變數
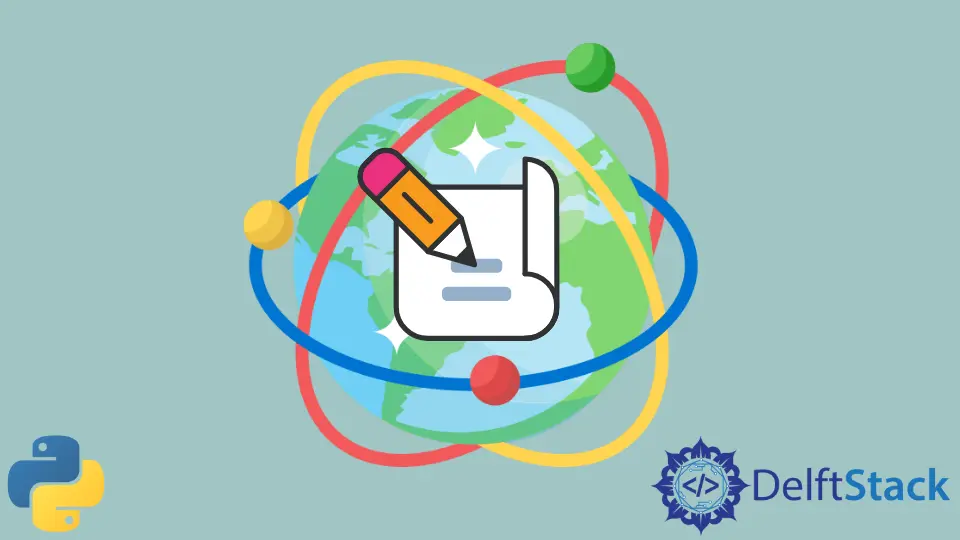
全域性變數是一個可見變數,可以在程式的每個部分使用。
全域性變數也不在任何函式或方法中定義。另一方面,區域性變數是在函式中定義的,並且只能在這些函式中使用。
全域性範圍或環境由一組在整個程式中可見的變數組成。在 Python 中,作用域可能是 Python 程式中可以訪問和使用變數、函式或模組的區域。
通常,在程式設計中,全域性作用域取代塊作用域和區域性作用域。
在程式設計中,變數被稱為可用於儲存值的記憶體位置。但是,在 Python 中,在類下建立的變數稱為類變數,而在特定物件下宣告的變數可能稱為例項變數。
類變數通常在所有方法和其他變數之外的類定義下宣告,而全域性類變數則在類之外宣告。它們可以被任何方法和類訪問。
全域性變數定義如下。
model = "SPlaid"
class Cars:
如下所示,任何類或類中的方法都可以通過簡單地呼叫變數名來訪問全域性變數。多個類和方法也可以同時訪問全域性變數。
car_type = "SUV"
print("Outside all classes", car_type)
class Tesla:
print("Type of the car within the Tesla class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_tesla(self):
print("Type of car within a Tesla method:", car_type)
# creating object to access method
#
tsl_object = Tesla(car_type)
tsl_object.display_tesla()
class Lucid:
print("Type of the car within the Lucid class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_lucid(self):
print("Type of the car within the Lucid method:", car_type)
# creating an object to access the method within the Lucid Class.
lucid_object = Lucid(car_type)
lucid_object.display_lucid()
輸出:
Outside all classes SUV
Type of the car within the Tesla class is: SUV
Type of car within a Tesla method: SUV
Type of the car within the Lucid class is: SUV
Type of the car within the Lucid method: SUV
將類變數定義為全域性變數
我們還可以使用 global
關鍵字修改區域性範圍變數以使其在全域性範圍內可用。
在函式中定義的任何變數都被認為是區域性變數,因此只能在區域性範圍內使用。但是,使用 global 關鍵字,我們可以將區域性變數的範圍更改為可全域性訪問。
同樣,我們也可以使類變數在使用 global 關鍵字定義的類之外可訪問。類變數可以被該特定類之外的其他類和方法使用。
在下面的程式碼片段中,我們將嘗試在類之外使用類變數。我們肯定會得到一個錯誤,讓我們知道變數沒有定義。
class Tesla:
# creating a class variable
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside the Tesla Class", speed)
輸出:
print("Accessing the class variable outside the Tesla Class", speed)
NameError: name 'speed' is not defined
如上面的程式碼示例所示,我們試圖訪問在類 Tesla 中定義的類變數。但是,這會導致錯誤,因為在類中宣告的變數只能在該類中單獨訪問。
但是,如前所述,我們可以通過在定義類變數之前使用 global 關鍵字來避免此錯誤。只有這樣我們才能訪問類外的變數。
class Tesla:
# creating a class variable and making it a variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside of the Tesla class", speed)
輸出:
Accessing speed variable within the class: 60
Accessing the class variable outside of the Tesla class 60
一旦我們將類變數宣告為全域性變數,它就可以在它自己的類之外和其他類中訪問。
在上面的程式碼片段中,我們從 Lucid 類訪問在 Tesla 類中定義為全域性變數的速度變數。與前面出現錯誤的示例不同,我們可以訪問該變數,因為它現在在全域性範圍內可用。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
# accessing the speed variable from outside the class
print("Accessing the class variable", speed)
class Lucid:
# accessing the speed variables from a different class
print("Accessing the speed variable from a different class:", speed)
輸出:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
有趣的是,宣告的全域性變數,例如速度變數,也可以被另一個類的方法訪問和使用。
例如,在下面的程式碼圖中,Lucid 類中定義的方法可以訪問速度變數。僅當 global 關鍵字位於宣告之前的類變數之前時,這才是可能的。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable", speed)
class Lucid:
print("Accessing the speed variable from a different class:", speed)
def __init__(self, speed):
self.speed = speed
def display_tesla_speed(self):
print("Accessing the speed variable from a method in another class:", speed)
lucid_object = Lucid(speed)
lucid_object.display_tesla_speed()
輸出:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Accessing the speed variable from a method in another class: 60
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn