在 Python 中定义类全局变量
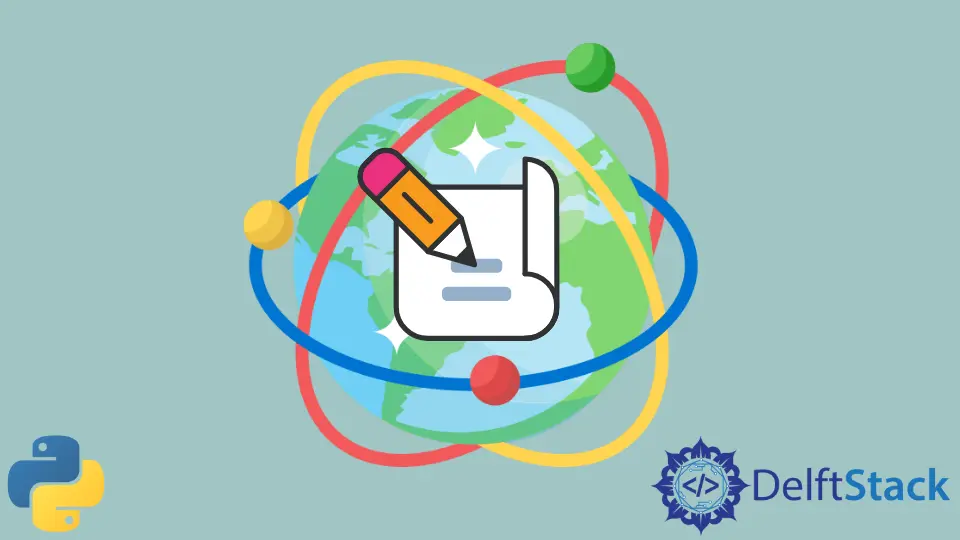
全局变量是一个可见变量,可以在程序的每个部分使用。
全局变量也不在任何函数或方法中定义。另一方面,局部变量是在函数中定义的,并且只能在这些函数中使用。
全局范围或环境由一组在整个程序中可见的变量组成。在 Python 中,作用域可能是 Python 程序中可以访问和使用变量、函数或模块的区域。
通常,在编程中,全局作用域取代块作用域和局部作用域。
在编程中,变量被称为可用于存储值的内存位置。但是,在 Python 中,在类下创建的变量称为类变量,而在特定对象下声明的变量可能称为实例变量。
类变量通常在所有方法和其他变量之外的类定义下声明,而全局类变量则在类之外声明。它们可以被任何方法和类访问。
全局变量定义如下。
model = "SPlaid"
class Cars:
如下所示,任何类或类中的方法都可以通过简单地调用变量名来访问全局变量。多个类和方法也可以同时访问全局变量。
car_type = "SUV"
print("Outside all classes", car_type)
class Tesla:
print("Type of the car within the Tesla class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_tesla(self):
print("Type of car within a Tesla method:", car_type)
# creating object to access method
#
tsl_object = Tesla(car_type)
tsl_object.display_tesla()
class Lucid:
print("Type of the car within the Lucid class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_lucid(self):
print("Type of the car within the Lucid method:", car_type)
# creating an object to access the method within the Lucid Class.
lucid_object = Lucid(car_type)
lucid_object.display_lucid()
输出:
Outside all classes SUV
Type of the car within the Tesla class is: SUV
Type of car within a Tesla method: SUV
Type of the car within the Lucid class is: SUV
Type of the car within the Lucid method: SUV
将类变量定义为全局变量
我们还可以使用 global
关键字修改局部范围变量以使其在全局范围内可用。
在函数中定义的任何变量都被认为是局部变量,因此只能在局部范围内使用。但是,使用 global 关键字,我们可以将局部变量的范围更改为可全局访问。
同样,我们也可以使类变量在使用 global 关键字定义的类之外可访问。类变量可以被该特定类之外的其他类和方法使用。
在下面的代码片段中,我们将尝试在类之外使用类变量。我们肯定会得到一个错误,让我们知道变量没有定义。
class Tesla:
# creating a class variable
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside the Tesla Class", speed)
输出:
print("Accessing the class variable outside the Tesla Class", speed)
NameError: name 'speed' is not defined
如上面的代码示例所示,我们试图访问在类 Tesla 中定义的类变量。但是,这会导致错误,因为在类中声明的变量只能在该类中单独访问。
但是,如前所述,我们可以通过在定义类变量之前使用 global 关键字来避免此错误。只有这样我们才能访问类外的变量。
class Tesla:
# creating a class variable and making it a variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside of the Tesla class", speed)
输出:
Accessing speed variable within the class: 60
Accessing the class variable outside of the Tesla class 60
一旦我们将类变量声明为全局变量,它就可以在它自己的类之外和其他类中访问。
在上面的代码片段中,我们从 Lucid 类访问在 Tesla 类中定义为全局变量的速度变量。与前面出现错误的示例不同,我们可以访问该变量,因为它现在在全局范围内可用。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
# accessing the speed variable from outside the class
print("Accessing the class variable", speed)
class Lucid:
# accessing the speed variables from a different class
print("Accessing the speed variable from a different class:", speed)
输出:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
有趣的是,声明的全局变量,例如速度变量,也可以被另一个类的方法访问和使用。
例如,在下面的代码图中,Lucid 类中定义的方法可以访问速度变量。仅当 global 关键字位于声明之前的类变量之前时,这才是可能的。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable", speed)
class Lucid:
print("Accessing the speed variable from a different class:", speed)
def __init__(self, speed):
self.speed = speed
def display_tesla_speed(self):
print("Accessing the speed variable from a method in another class:", speed)
lucid_object = Lucid(speed)
lucid_object.display_tesla_speed()
输出:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Accessing the speed variable from a method in another class: 60
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn