在 Python 中检查变量类型
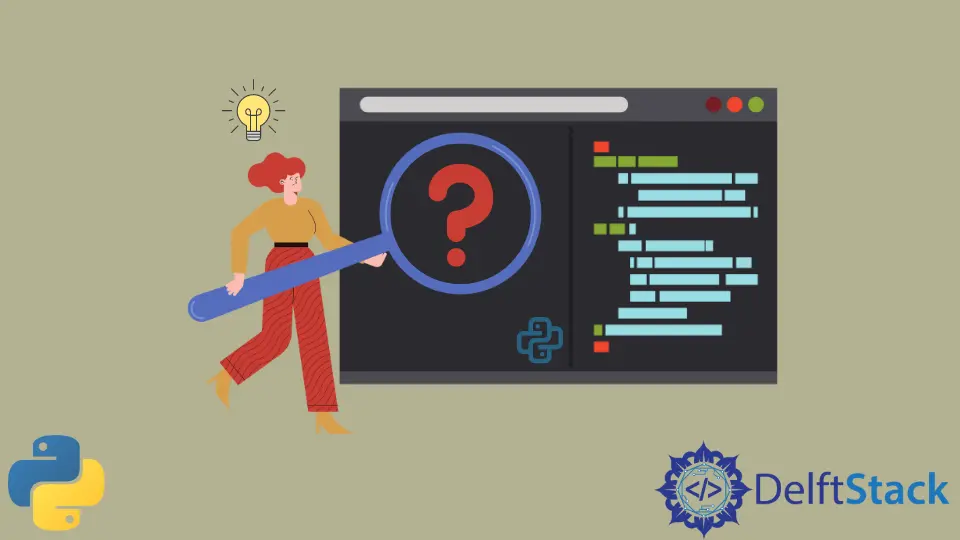
在 Python 中,如果你想存储任何一种数据,对这些数据进行一些操作,或者在函数或对象之间传递数据,你首先要把这些数据存储在某个地方。这是在变量的帮助下完成的。
Python 中的变量及其类型
变量不过是一个盒子或容器,我们的数据将被存储在里面。变量将被分配到内存(RAM)的某个空间。数据类型告诉我们一个变量存放的数据类型。而根据数据的类型,编译器会决定在内存里面给这个变量分配多少空间。然后会相应的分配内存。
在 Python 中,你不必像在 C/C++、Java 或其他主要编程语言中那样,明确定义你将存储在变量里面的数据类型。在这里,你可以直接给变量赋值,编译器会识别出变量存放的数据是什么类型,属于整数、字符串、列表等哪个类。
# Variable of type String
newVariable = "This is a new variable"
print(newVariable)
# Variable of type Boolean
booleanVariable = True
print(booleanVariable)
# Variable of type Float
floatVariable = 20.30
print(floatVariable)
输出:
This is a new variable
True
20.3
在 Python 中检查变量的类型
变量可以来自 Python 中的任何一种数据类型,下面都会提到。
- 数字:这一类包含整数、浮点数和复数。
- 字符串:它是 Unicode 字符的序列。Unicode 是一个字符集,包含了全世界所有语言的字符和符号。
- 布尔型:布尔型代表
True
或False
。 - 列表:它是不同数据类型元素的有序集合。列表是可以突变的,也就是说在创建了列表之后,列表里面的值是可以改变的。
- 元组:它也是不同数据类型元素的有序集合。它也是不同数据类型元素的有序集合。列表和元组的唯一区别是,元组是不可变的,也就是说一旦创建了元组,就不能修改。
- 集合:集合是一个无序的不含重复元素的集合。
- 字典:它是键值对的无序集合。键和值可以是任何类型。
在 Python 中,有两种方法可以检查一个变量的类型。
使用 type()
函数来检查 Python 中的变量类型
要检查一个变量的类型,你可以使用 type()
函数,它将变量作为输入。在这个函数中,你必须传递变量名或变量值本身,它将返回变量的数据类型。它将返回变量的数据类型。
myInt = 50
print(type(myInt))
myFloat = 10.50
print(type(myFloat))
myString = "My name is Adam"
print(type(myString))
输出:
<class 'int'>
<class 'float'>
<class 'str'>
在 Python 中使用 isinstance()
函数检查变量类型
另一个可以用来检查变量类型的函数是 isinstance()
。你需要传递两个参数,第一个是变量(你想找到其数据类型的值),第二个参数是变量类型。如果变量类型与你在第二个参数中指定的类型相同,它将返回 True
,否则返回 False
。
# A variable 'myVar' having a value 50 inside
myVar = 50
# Printing the value returned by isinstance() function
print("Does myVar belongs to int: ", isinstance(myVar, int))
# This will return false
# As the value passed is string and you are checking it with int
print("Does string belongs to int: ", isinstance("My String", int))
complexNo = 1 + 2j
print("This this number complex: ", isinstance(complexNo, complex))
输出:
Does myVar belongs to int: True
Does string belongs to int: False
This this number complex: True
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn