Python 中的私有变量
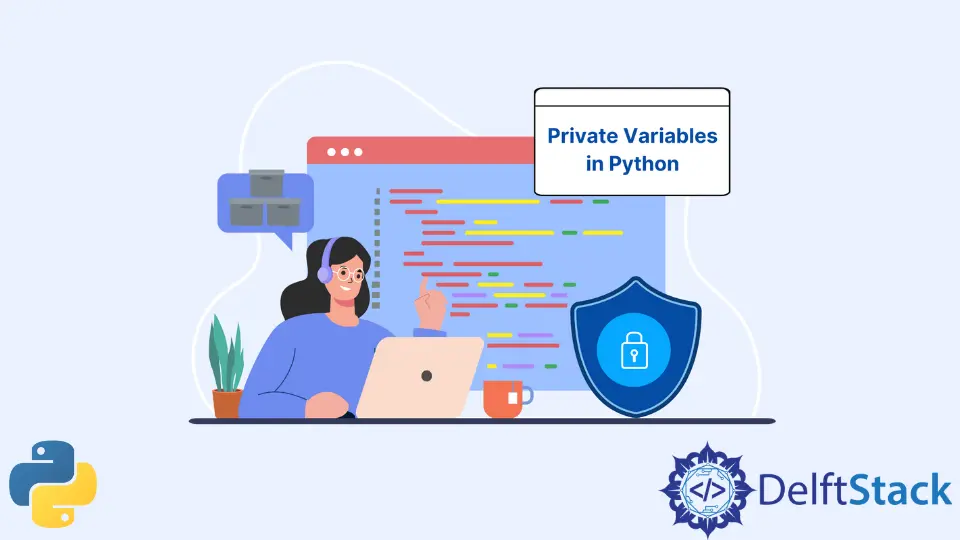
私有变量在程序中用于访问控制。Java 和 C++ 等编程语言已指定关键字来定义私有变量。
在 Python 中,我们没有定义私有变量的关键字。本文将讨论如何实现私有变量并使用类名访问它们。
什么是 Python 中的私有变量
我们不能在 Python 中创建私有变量,但我们可以使用特殊的命名约定来模拟私有变量的实现。
我们可以使用语法 objectName.attributeName
访问对象的任何属性。看例子。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.SSN)
输出:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
我们创建了一个类 Person
,其属性为 name
、age
和 SSN
。在创建 Person
类的实例 person1
后,我们使用语法 person1.name
、person1.age
和 person1
在类外部访问属性 name
、age
和 SSN
.SSN`。
我们不希望在类定义之外访问 SSN
。我们没有任何关键字可以在 Python 中创建私有变量。那么我们应该如何模拟私有变量的实现呢?
在 Python 中如何实现私有变量
为了在 Python 中实现私有变量,我们将在变量名前使用双下划线 (__)
。让我们使用双下划线定义 Person
类的属性 SSN
。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
如果我们创建 Person
类的实例 person1
并使用语法 person1.__SSN
访问属性 __SSN
,程序会显示错误并引发下面的 AttributeError
异常。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.__SSN)
输出:
Name of the person is: Alex
Age of the person is: 15
/usr/lib/Python3/dist-packages/requests/__init__.py:89: RequestsDependencyWarning: urllib3 (1.26.7) or chardet (3.0.4) doesn't match a supported version!
warnings.warn("urllib3 ({}) or chardet ({}) doesn't match a supported "
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/PythonProject/string1.py", line 11, in <module>
print("SSN of the person is:", person1.__SSN)
AttributeError: 'Person' object has no attribute '__SSN'
我们可以访问除 __SSN
之外的所有其他属性。该程序说没有名为 __SSN
的属性。那么我们如何访问属性 __SSN
中的值呢?
在 Python 中访问私有变量
我们不能在类定义之外访问私有变量,但我们可以在内部访问它。因此,我们可以在类内部创建一个方法来访问私有变量值。
我们可以创建一个名为 getSSN()
的方法,它返回属性 __SSN
的值,如下所示。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.getSSN())
输出:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
我们可以直接访问私有变量,而不是定义方法。让我们看看私有变量是如何存储在内存中的。当我们用双下划线 (__
) 定义变量名时,Python 解释器会说没有这样的属性。
要了解变量的存储方式,我们将检查给定对象的所有属性。我们将使用 dir()
函数,它接受一个对象或输入参数并返回所有对象属性的列表。让我们使用 dir()
函数检查 person1
对象的属性。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Attributes of the Person are:")
print(dir(person1))
输出:
Attributes of the Person are:
['_Person__SSN', '__doc__', '__init__', '__module__', 'age', 'getSSN', 'name']
观察 name
和 age
属性是否存在,但没有 __SSN
属性。但是,有一个名为 _Person__SSN
的属性。让我们为对象 person1
打印此属性的值。
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1._Person__SSN)
输出:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
注意属性 _Person__SSN
与 __SSN
具有相同的值。属性 __SSN
在程序中存储为 _Person__SSN
。每当我们访问属性 __SSN
时,程序都会遇到 AttributeError
异常。
在 Python 中,当我们使用双下划线 (__
) 定义属性时,程序会在属性名称前添加 _className
。这个过程称为重整。
Python 认为使用双下划线命名的属性是修改中的私有变量。然而,这不是真的,因为我们可以使用语法 _className__attributeName
访问变量。
Python 私有变量的结论
在本文中,我们讨论了 Python 中的私有变量。我们已经看到,与其他编程语言不同,我们没有任何特定的关键字来在 Python 中声明私有变量。但是,我们可以通过使用双下划线字符命名变量来模拟私有变量的实现。
我们还讨论了在 Python 中访问私有变量的两种方法。我们建议使用方法来访问私有变量。使用带有类名的方法将破坏实现私有变量的本质,因为我们将直接访问该变量。该程序会警告你在属性名称之前使用 _className
访问私有变量。
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub