Python의 개인 변수
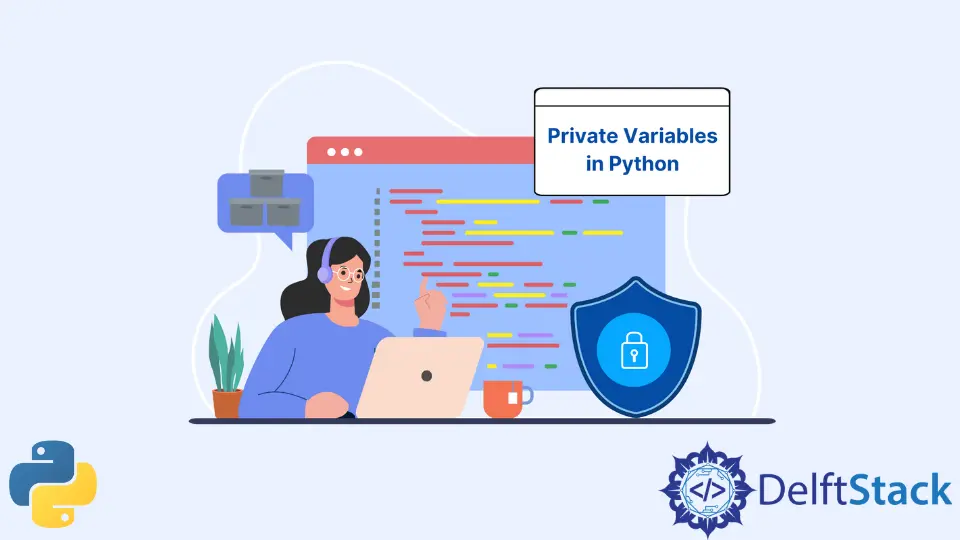
개인 변수는 액세스 제어를 위해 프로그램에서 사용됩니다. Java 및 C++와 같은 프로그래밍 언어에는 개인 변수를 정의하는 키워드가 지정되어 있습니다.
Python에는 개인 변수를 정의하는 키워드가 없습니다. 이 기사에서는 개인 변수를 구현하고 클래스 이름을 사용하여 액세스하는 방법에 대해 설명합니다.
파이썬에서 개인 변수란 무엇입니까?
Python에서 개인 변수를 만들 수는 없지만 특별한 명명 규칙을 사용하여 개인 변수 구현을 시뮬레이션할 수 있습니다.
objectName.attributeName
구문을 사용하여 객체의 모든 속성에 액세스할 수 있습니다. 예를 보십시오.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.SSN)
출력:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
name
, age
및 SSN
속성을 가진 Person
클래스를 만들었습니다. Person
클래스의 인스턴스 person1
을 생성한 후 person1.name
, person1.age
및 person1 구문을 사용하여 클래스 외부의
name,
age및
SSN 속성에 액세스했습니다. .SSN
.
SSN
이 클래스 정의 외부에서 액세스되는 것을 원하지 않습니다. Python에서 개인 변수를 생성하기 위한 키워드가 없습니다. 그렇다면 개인 변수의 구현을 어떻게 시뮬레이트해야 할까요?
Python에서 개인 변수를 구현하는 방법은 무엇입니까?
파이썬에서 개인 변수를 구현하기 위해 변수 이름 앞에 이중 밑줄 (__)
을 사용합니다. 이중 밑줄을 사용하여 Person
클래스의 SSN
속성을 정의해 보겠습니다.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
Person
클래스의 인스턴스 person1
을 만들고 person1.__SSN
구문을 사용하여 속성 __SSN
에 액세스하면 프로그램에서 오류를 표시하고 아래 AttributeError
예외를 발생시킵니다.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.__SSN)
출력:
Name of the person is: Alex
Age of the person is: 15
/usr/lib/Python3/dist-packages/requests/__init__.py:89: RequestsDependencyWarning: urllib3 (1.26.7) or chardet (3.0.4) doesn't match a supported version!
warnings.warn("urllib3 ({}) or chardet ({}) doesn't match a supported "
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/PythonProject/string1.py", line 11, in <module>
print("SSN of the person is:", person1.__SSN)
AttributeError: 'Person' object has no attribute '__SSN'
__SSN
을 제외한 다른 모든 속성에 액세스할 수 있습니다. 프로그램에 __SSN
이라는 속성이 없다고 표시됩니다. 그렇다면 __SSN
속성의 값에 어떻게 액세스할 수 있습니까?
Python에서 개인 변수에 액세스
클래스 정의 외부에서는 private 변수에 액세스할 수 없지만 내부에서는 액세스할 수 있습니다. 따라서 클래스 내부에 개인 변수 값에 액세스하는 메서드를 만들 수 있습니다.
아래와 같이 __SSN
속성의 값을 반환하는 getSSN()
이라는 메서드를 만들 수 있습니다.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.getSSN())
출력:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
메서드를 정의하는 대신 개인 변수에 직접 액세스할 수 있습니다. 개인 변수가 메모리에 어떻게 저장되는지 봅시다. 이중 밑줄(__
)로 변수 이름을 정의할 때 Python 인터프리터는 그러한 속성이 없다고 말합니다.
변수가 어떻게 저장되는지 이해하기 위해 주어진 객체의 모든 속성을 확인할 것입니다. 객체 또는 입력 인수를 취하고 모든 객체 속성의 목록을 반환하는 dir()
함수를 사용할 것입니다. dir()
함수를 사용하여 person1
객체의 속성을 확인합시다.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Attributes of the Person are:")
print(dir(person1))
출력:
Attributes of the Person are:
['_Person__SSN', '__doc__', '__init__', '__module__', 'age', 'getSSN', 'name']
name
및 age
속성은 있지만 __SSN
속성은 없는지 확인합니다. 그러나 _Person__SSN
이라는 속성이 있습니다. 객체 person1
에 대한 이 속성의 값을 인쇄해 보겠습니다.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1._Person__SSN)
출력:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
속성 _Person__SSN
이 __SSN
과 동일한 값을 갖는지 확인하십시오. 속성 __SSN
은 프로그램에서 _Person__SSN
으로 저장됩니다. __SSN
속성에 액세스할 때마다 프로그램에서 AttributeError
예외가 실행됩니다.
Python에서 이중 밑줄(__
)을 사용하여 속성을 정의할 때 프로그램은 속성 이름 앞에 _className
을 추가합니다. 이 과정을 맹글링이라고 합니다.
파이썬은 이중 밑줄을 사용하여 명명된 속성이 맹글링에서 개인 변수라고 인식합니다. 그러나 _className__attributeName
구문을 사용하여 변수에 액세스할 수 있으므로 이는 사실이 아닙니다.
Python 개인 변수의 결론
이 기사에서 우리는 Python의 개인 변수에 대해 논의했습니다. 우리는 다른 프로그래밍 언어와 달리 Python에서 개인 변수를 선언하는 특정 키워드가 없음을 확인했습니다. 그러나 이중 밑줄 문자를 사용하여 변수 이름을 지정하여 개인 변수 구현을 시뮬레이션할 수 있습니다.
또한 Python에서 개인 변수에 액세스하는 두 가지 방법에 대해 논의했습니다. 개인 변수에 액세스하는 방법을 사용하는 것이 좋습니다. 클래스 이름과 함께 접근 방식을 사용하면 변수에 직접 액세스하므로 개인 변수 구현의 본질이 파괴됩니다. 프로그램은 속성 이름 앞에 _className
을 사용하여 개인 변수에 액세스하라는 경고를 표시합니다.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub