How to Implement Private Variables in Python
- What Is a Private Variable in Python?
- How to Implement a Private Variable in Python?
- Access Private Variables in Python
- Conclusion of Python Private Variables
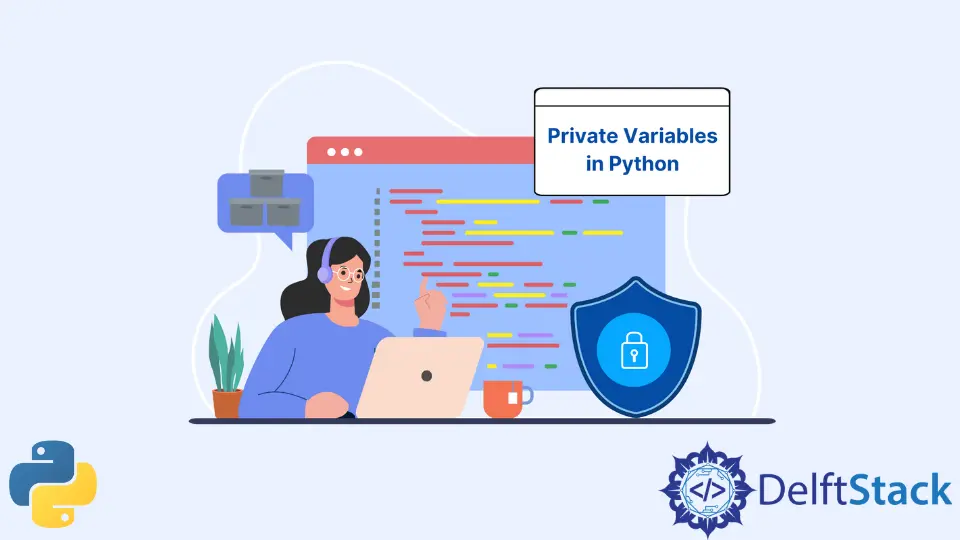
Private variables are used in programs for access control. Programming languages like Java and C++ have specified keywords to define a private variable.
In Python, we don’t have a keyword to define private variables. This article will discuss how to implement private variables and access them using the name of a class.
What Is a Private Variable in Python?
We cannot create a private variable in Python, but we can simulate the implementation of private variables using special naming conventions.
We can access any attribute of an object using the syntax objectName.attributeName
. Look at the example.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.SSN)
Output:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
We have created a class Person
with attributes name
, age
, and SSN
. After creating an instance person1
of the Person
class, we accessed attributes name
, age
, and SSN
outside the class using the syntax person1.name
, person1.age
, and person1.SSN
.
We don’t want the SSN
to be accessed outside the class definition. We don’t have any keywords to create a private variable in Python. So how should we simulate the implementation of a private variable?
How to Implement a Private Variable in Python?
To implement a private variable in Python, we will use double underscore (__)
before the variable name. Let’s define the attribute SSN
of the Person
class using double underscore.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
If we create an instance person1
of the Person
class and access the attribute __SSN
using the syntax person1.__SSN
, the program shows an error and raises the AttributeError
exception below.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.__SSN)
Output:
Name of the person is: Alex
Age of the person is: 15
/usr/lib/Python3/dist-packages/requests/__init__.py:89: RequestsDependencyWarning: urllib3 (1.26.7) or chardet (3.0.4) doesn't match a supported version!
warnings.warn("urllib3 ({}) or chardet ({}) doesn't match a supported "
Traceback (most recent call last):
File "/home/aditya1117/PycharmProjects/PythonProject/string1.py", line 11, in <module>
print("SSN of the person is:", person1.__SSN)
AttributeError: 'Person' object has no attribute '__SSN'
We can access all other attributes but __SSN
. The program says there’s no attribute named __SSN
. How can we access the value in the attribute __SSN
then?
Access Private Variables in Python
We can’t access a private variable outside the class definition, but we can access it inside. So, we can create a method inside the class to access the private variable value.
We can create a method named getSSN()
that returns the value of the attribute __SSN
as shown below.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1.getSSN())
Output:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
Instead of defining a method, we can directly access the private variable. Let’s see how the private variables are stored in the memory. When we define a variable name with the double underscore (__
), the Python interpreter says that there’s no such attribute.
To understand how the variable is stored, we will check all the attributes of the given object. We will use the dir()
function, which takes an object or input arguments and returns a list of all the object attributes. Let’s check the attributes of the person1
object using the dir()
function.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Attributes of the Person are:")
print(dir(person1))
Output:
Attributes of the Person are:
['_Person__SSN', '__doc__', '__init__', '__module__', 'age', 'getSSN', 'name']
Observe that the name
and age
attributes are present, but there’s no __SSN
attribute. However, there’s an attribute named _Person__SSN
. Let’s print the value of this attribute for the object person1
.
class Person:
def __init__(self, name, age, SSN):
self.name = name
self.age = age
self.__SSN = SSN
def getSSN(self):
return self.__SSN
person1 = Person(name="Alex", age=15, SSN=1234567890)
print("Name of the person is:", person1.name)
print("Age of the person is:", person1.age)
print("SSN of the person is:", person1._Person__SSN)
Output:
Name of the person is: Alex
Age of the person is: 15
SSN of the person is: 1234567890
Observe that the attribute _Person__SSN
has the same value as __SSN
. The attribute __SSN
is stored as _Person__SSN
in the program. Whenever we access the attribute __SSN
, the program runs into an AttributeError
exception.
In Python, when we define an attribute using double underscore (__
), the program adds _className
before the attribute name. This process is called mangling.
Python perceives that the attributes named using double underscores are private variables in mangling. However, this isn’t true as we can access the variables using the syntax _className__attributeName
.
Conclusion of Python Private Variables
In this article, we discussed private variables in Python. We have seen that we don’t have any specific keyword to declare a private variable in Python, unlike other programming languages. However, we can simulate the implementation of private variables by naming the variables using a double underscore character.
We also discussed two ways to access private variables in Python. We would suggest using methods to access private variables. Using the approach with the class name will destroy the essence of implementing private variables as we will be accessing the variable directly. The program gives you the warning to access the private variables using the _className
before the attribute name.
Aditya Raj is a highly skilled technical professional with a background in IT and business, holding an Integrated B.Tech (IT) and MBA (IT) from the Indian Institute of Information Technology Allahabad. With a solid foundation in data analytics, programming languages (C, Java, Python), and software environments, Aditya has excelled in various roles. He has significant experience as a Technical Content Writer for Python on multiple platforms and has interned in data analytics at Apollo Clinics. His projects demonstrate a keen interest in cutting-edge technology and problem-solving, showcasing his proficiency in areas like data mining and software development. Aditya's achievements include securing a top position in a project demonstration competition and gaining certifications in Python, SQL, and digital marketing fundamentals.
GitHub