How to Define a Class Global Variable in Python
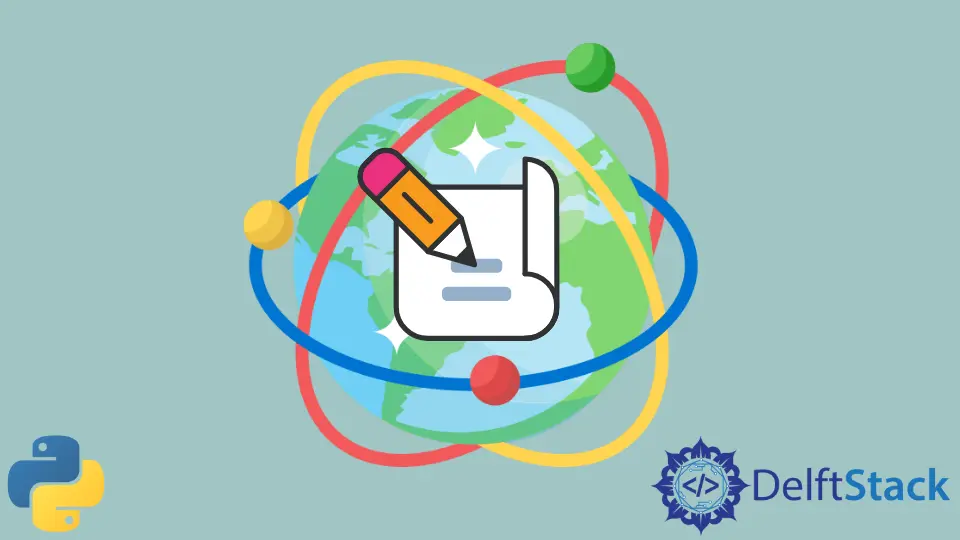
A global variable is a visible variable and can be used in every part of a program.
Global variables are also not defined within any function or method. On the other hand, local variables are defined within functions and can only be used within those function(s).
A global scope or environment is composed of a set of variables visible throughout the program. In Python, the scope may be an area in a Python program where a variable, function, or module can be accessed and used.
Generally, in programming, the global scope supersedes both block and local scope.
In Programming, variables are referred to as memory locations that can be used to store values. However, in Python, variables created under a class are called Class variables, while those declared under a particular object may be referred to as instance variables.
While Class variables are normally declared under the class definition outside all methods and other variables, Global Class variables are declared outside a class. They can be accessed by any method and class.
Global variables are defined as follows.
model = "SPlaid"
class Cars:
As shown below, global variables can be accessed by any class or method within a class by simply calling the variable’s name. Global variables can also be accessed by multiple classes and methods at the same time.
car_type = "SUV"
print("Outside all classes", car_type)
class Tesla:
print("Type of the car within the Tesla class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_tesla(self):
print("Type of car within a Tesla method:", car_type)
# creating object to access method
#
tsl_object = Tesla(car_type)
tsl_object.display_tesla()
class Lucid:
print("Type of the car within the Lucid class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_lucid(self):
print("Type of the car within the Lucid method:", car_type)
# creating an object to access the method within the Lucid Class.
lucid_object = Lucid(car_type)
lucid_object.display_lucid()
Output:
Outside all classes SUV
Type of the car within the Tesla class is: SUV
Type of car within a Tesla method: SUV
Type of the car within the Lucid class is: SUV
Type of the car within the Lucid method: SUV
Define Class Variables as Global Variables
We can also modify a local scope variable to become available in the global scope using the global
keyword.
Any variable defined within a function is considered a local variable and thus can only be used within the local scope. However, using the global keyword, we can change the scope of a local variable to be accessible globally.
Similarly, we can also make a class variable accessible outside the class that has been defined using the global keyword. The class variable can be used by other classes and methods outside that particular class.
In the snippet below, we are going to try and use a class variable outside the class. We will certainly get an error letting us know that the variable is not defined.
class Tesla:
# creating a class variable
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside the Tesla Class", speed)
Output:
print("Accessing the class variable outside the Tesla Class", speed)
NameError: name 'speed' is not defined
As seen in the code example above, we are trying to access the class variable defined within the class Tesla. However, this results in an error since a variable declared within a class is only accessible within that class alone.
But, as mentioned before, we can avert this error by using the global keyword before defining the class variable. Only then can we access the variable outside the class.
class Tesla:
# creating a class variable and making it a variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside of the Tesla class", speed)
Output:
Accessing speed variable within the class: 60
Accessing the class variable outside of the Tesla class 60
Once we have declared the class variable as a global variable, it is accessible outside its own class and within other classes.
In the code snippet above, we access the speed variable defined as a global variable in the class Tesla from the class Lucid. Unlike in the earlier example where we got an error, we can access the variable since it is now available in the global scope.
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
# accessing the speed variable from outside the class
print("Accessing the class variable", speed)
class Lucid:
# accessing the speed variables from a different class
print("Accessing the speed variable from a different class:", speed)
Output:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Interestingly, global variables declared, such as speed variables, can also be accessed and used by methods of another class.
For instance, in the code illustration below, a method defined in the Lucid class has access to the speed variable. This is only possible when the global keyword precedes a class variable before a declaration.
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable", speed)
class Lucid:
print("Accessing the speed variable from a different class:", speed)
def __init__(self, speed):
self.speed = speed
def display_tesla_speed(self):
print("Accessing the speed variable from a method in another class:", speed)
lucid_object = Lucid(speed)
lucid_object.display_tesla_speed()
Output:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Accessing the speed variable from a method in another class: 60
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn