Python でクラスグローバル変数を定義する
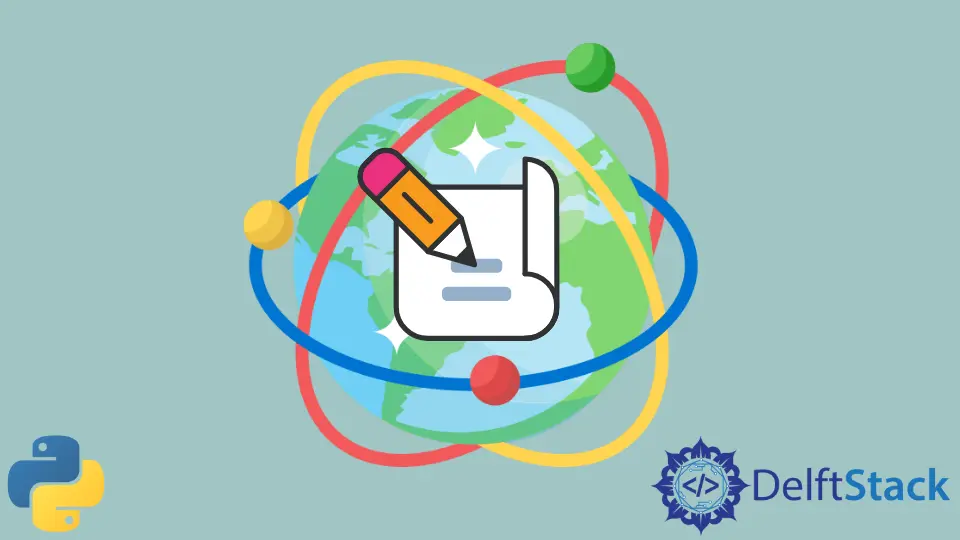
グローバル変数は可視変数であり、プログラムのすべての部分で使用できます。
グローバル変数も、関数またはメソッド内で定義されていません。一方、ローカル変数は関数内で定義され、それらの関数内でのみ使用できます。
グローバルスコープまたは環境は、プログラム全体で表示される一連の変数で構成されます。Python では、スコープは、変数、関数、またはモジュールにアクセスして使用できる Python プログラムの領域である場合があります。
一般に、プログラミングでは、グローバルスコープがブロックスコープとローカルスコープの両方に優先します。
プログラミングでは、変数は値を格納するために使用できるメモリ位置と呼ばれます。ただし、Python では、クラスの下で作成された変数はクラス変数と呼ばれ、特定のオブジェクトの下で宣言された変数はインスタンス変数と呼ばれる場合があります。
クラス変数は通常、すべてのメソッドおよびその他の変数の外部でクラス定義の下で宣言されますが、グローバルクラス変数はクラスの外部で宣言されます。これらは、任意のメソッドとクラスからアクセスできます。
グローバル変数は次のように定義されます。
model = "SPlaid"
class Cars:
以下に示すように、グローバル変数には、変数の名前を呼び出すだけで、クラス内の任意のクラスまたはメソッドからアクセスできます。グローバル変数には、複数のクラスとメソッドから同時にアクセスすることもできます。
car_type = "SUV"
print("Outside all classes", car_type)
class Tesla:
print("Type of the car within the Tesla class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_tesla(self):
print("Type of car within a Tesla method:", car_type)
# creating object to access method
#
tsl_object = Tesla(car_type)
tsl_object.display_tesla()
class Lucid:
print("Type of the car within the Lucid class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_lucid(self):
print("Type of the car within the Lucid method:", car_type)
# creating an object to access the method within the Lucid Class.
lucid_object = Lucid(car_type)
lucid_object.display_lucid()
出力:
Outside all classes SUV
Type of the car within the Tesla class is: SUV
Type of car within a Tesla method: SUV
Type of the car within the Lucid class is: SUV
Type of the car within the Lucid method: SUV
クラス変数をグローバル変数として定義する
global
キーワードを使用して、ローカルスコープ変数を変更してグローバルスコープで使用できるようにすることもできます。
関数内で定義された変数はすべてローカル変数と見なされるため、ローカルスコープ内でのみ使用できます。ただし、global キーワードを使用すると、ローカル変数のスコープを変更して、グローバルにアクセスできるようにすることができます。
同様に、global キーワードを使用して定義されたクラスの外部でクラス変数にアクセスできるようにすることもできます。クラス変数は、その特定のクラス外の他のクラスおよびメソッドで使用できます。
以下のスニペットでは、クラス外のクラス変数を使用してみます。変数が定義されていないことを知らせるエラーが確実に発生します。
class Tesla:
# creating a class variable
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside the Tesla Class", speed)
出力:
print("Accessing the class variable outside the Tesla Class", speed)
NameError: name 'speed' is not defined
上記のコード例に見られるように、クラス Tesla 内で定義されたクラス変数にアクセスしようとしています。ただし、クラス内で宣言された変数はそのクラス内でのみアクセス可能であるため、これによりエラーが発生します。
ただし、前述のように、クラス変数を定義する前に global キーワードを使用することで、このエラーを回避できます。そうして初めて、クラス外の変数にアクセスできます。
class Tesla:
# creating a class variable and making it a variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside of the Tesla class", speed)
出力:
Accessing speed variable within the class: 60
Accessing the class variable outside of the Tesla class 60
クラス変数をグローバル変数として宣言すると、それ自体のクラスの外部および他のクラス内でアクセスできるようになります。
上記のコードスニペットでは、Lucid クラスの Tesla クラスでグローバル変数として定義されている速度変数にアクセスします。エラーが発生した前の例とは異なり、変数はグローバルスコープで使用できるようになったため、変数にアクセスできます。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
# accessing the speed variable from outside the class
print("Accessing the class variable", speed)
class Lucid:
# accessing the speed variables from a different class
print("Accessing the speed variable from a different class:", speed)
出力:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
興味深いことに、速度変数などの宣言されたグローバル変数は、別のクラスのメソッドからもアクセスして使用できます。
たとえば、次のコード図では、Lucid クラスで定義されたメソッドが速度変数にアクセスできます。これは、グローバルキーワードが宣言の前にクラス変数の前にある場合にのみ可能です。
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable", speed)
class Lucid:
print("Accessing the speed variable from a different class:", speed)
def __init__(self, speed):
self.speed = speed
def display_tesla_speed(self):
print("Accessing the speed variable from a method in another class:", speed)
lucid_object = Lucid(speed)
lucid_object.display_tesla_speed()
出力:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Accessing the speed variable from a method in another class: 60
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn