Python에서 클래스 전역 변수 정의
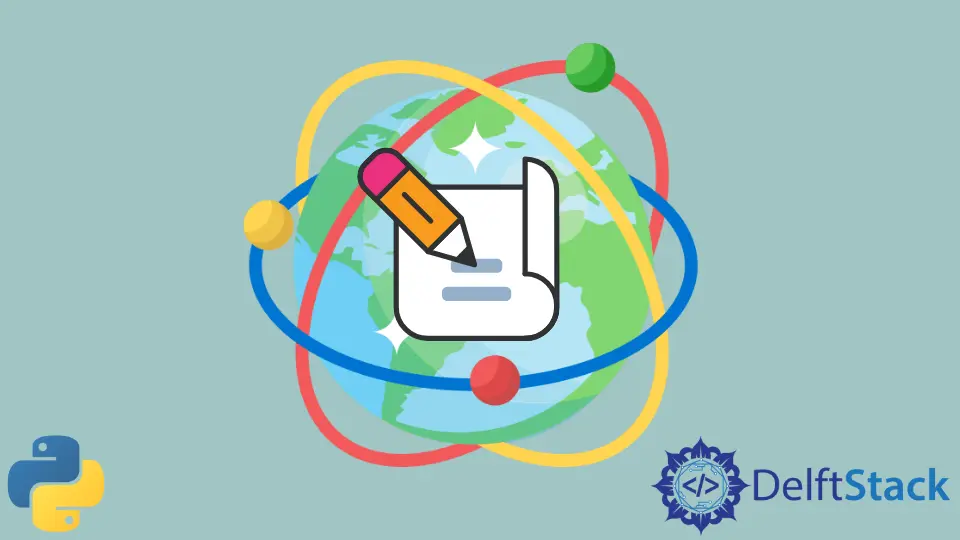
전역 변수는 가시적인 변수이며 프로그램의 모든 부분에서 사용할 수 있습니다.
전역 변수는 함수나 메서드 내에서도 정의되지 않습니다. 반면에 지역 변수는 함수 내에서 정의되며 해당 함수 내에서만 사용할 수 있습니다.
전역 범위 또는 환경은 프로그램 전체에서 볼 수 있는 변수 집합으로 구성됩니다. Python에서 범위는 변수, 함수 또는 모듈에 액세스하고 사용할 수 있는 Python 프로그램의 영역일 수 있습니다.
일반적으로 프로그래밍에서 전역 범위는 블록 및 로컬 범위를 모두 대체합니다.
프로그래밍에서 변수는 값을 저장하는 데 사용할 수 있는 메모리 위치라고 합니다. 그러나 Python에서는 클래스 아래에 생성된 변수를 클래스 변수라고 하고 특정 객체 아래에 선언된 변수를 인스턴스 변수라고 합니다.
클래스 변수는 일반적으로 모든 메서드 및 기타 변수 외부의 클래스 정의에서 선언되지만 전역 클래스 변수는 클래스 외부에서 선언됩니다. 모든 메서드와 클래스에서 액세스할 수 있습니다.
전역 변수는 다음과 같이 정의됩니다.
model = "SPlaid"
class Cars:
아래에서 볼 수 있듯이 전역 변수는 단순히 변수 이름을 호출하여 클래스 또는 클래스 내의 메서드에서 액세스할 수 있습니다. 전역 변수는 동시에 여러 클래스와 메서드에서 액세스할 수도 있습니다.
car_type = "SUV"
print("Outside all classes", car_type)
class Tesla:
print("Type of the car within the Tesla class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_tesla(self):
print("Type of car within a Tesla method:", car_type)
# creating object to access method
#
tsl_object = Tesla(car_type)
tsl_object.display_tesla()
class Lucid:
print("Type of the car within the Lucid class is:", car_type)
def __init__(self, car_type):
self.car_type = car_type
def display_lucid(self):
print("Type of the car within the Lucid method:", car_type)
# creating an object to access the method within the Lucid Class.
lucid_object = Lucid(car_type)
lucid_object.display_lucid()
출력:
Outside all classes SUV
Type of the car within the Tesla class is: SUV
Type of car within a Tesla method: SUV
Type of the car within the Lucid class is: SUV
Type of the car within the Lucid method: SUV
클래스 변수를 전역 변수로 정의
global
키워드를 사용하여 전역 범위에서 사용 가능하도록 지역 범위 변수를 수정할 수도 있습니다.
함수 내에 정의된 모든 변수는 지역 변수로 간주되므로 지역 범위 내에서만 사용할 수 있습니다. 그러나 global 키워드를 사용하여 전역적으로 액세스할 수 있도록 지역 변수의 범위를 변경할 수 있습니다.
마찬가지로 global 키워드를 사용하여 정의된 클래스 외부에서 클래스 변수에 액세스할 수 있도록 만들 수도 있습니다. 클래스 변수는 특정 클래스 외부의 다른 클래스 및 메소드에서 사용할 수 있습니다.
아래 스니펫에서는 클래스 외부의 클래스 변수를 사용하려고 합니다. 변수가 정의되지 않았음을 알리는 오류가 발생합니다.
class Tesla:
# creating a class variable
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside the Tesla Class", speed)
출력:
print("Accessing the class variable outside the Tesla Class", speed)
NameError: name 'speed' is not defined
위의 코드 예제에서 볼 수 있듯이 Tesla 클래스 내에 정의된 클래스 변수에 액세스하려고 합니다. 그러나 클래스 내에서 선언된 변수는 해당 클래스 내에서만 액세스할 수 있으므로 오류가 발생합니다.
그러나 앞에서 언급했듯이 클래스 변수를 정의하기 전에 global 키워드를 사용하여 이 오류를 방지할 수 있습니다. 그래야만 클래스 외부의 변수에 액세스할 수 있습니다.
class Tesla:
# creating a class variable and making it a variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable outside of the Tesla class", speed)
출력:
Accessing speed variable within the class: 60
Accessing the class variable outside of the Tesla class 60
클래스 변수를 전역 변수로 선언하면 자체 클래스 외부와 다른 클래스 내에서 액세스할 수 있습니다.
위의 코드 조각에서 Lucid 클래스에서 Tesla 클래스의 전역 변수로 정의된 속도 변수에 액세스합니다. 오류가 발생한 이전 예제와 달리 이제 전역 범위에서 사용할 수 있으므로 변수에 액세스할 수 있습니다.
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Acessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
# accessing the speed variable from outside the class
print("Accessing the class variable", speed)
class Lucid:
# accessing the speed variables from a different class
print("Accessing the speed variable from a different class:", speed)
출력:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
흥미롭게도 속도 변수와 같이 선언된 전역 변수는 다른 클래스의 메서드에서도 액세스 및 사용할 수 있습니다.
예를 들어 아래 코드 그림에서 Lucid 클래스에 정의된 메서드는 속도 변수에 액세스할 수 있습니다. 이는 선언 전에 클래스 변수 앞에 global 키워드가 있는 경우에만 가능합니다.
class Tesla:
# creating a class variable and making it a global variable
global speed
speed = 60
print("Accessing speed variable within the class:", speed)
def __init__(self, speed):
self.speed = speed
def display_speed():
print("Speed of the Tesla is:", speed)
print("Accessing the class variable", speed)
class Lucid:
print("Accessing the speed variable from a different class:", speed)
def __init__(self, speed):
self.speed = speed
def display_tesla_speed(self):
print("Accessing the speed variable from a method in another class:", speed)
lucid_object = Lucid(speed)
lucid_object.display_tesla_speed()
출력:
Accessing speed variable within the class: 60
Accessing the class variable 60
Accessing the speed variable from a different class: 60
Accessing the speed variable from a method in another class: 60
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn