How to Convert String to Datetime in Python
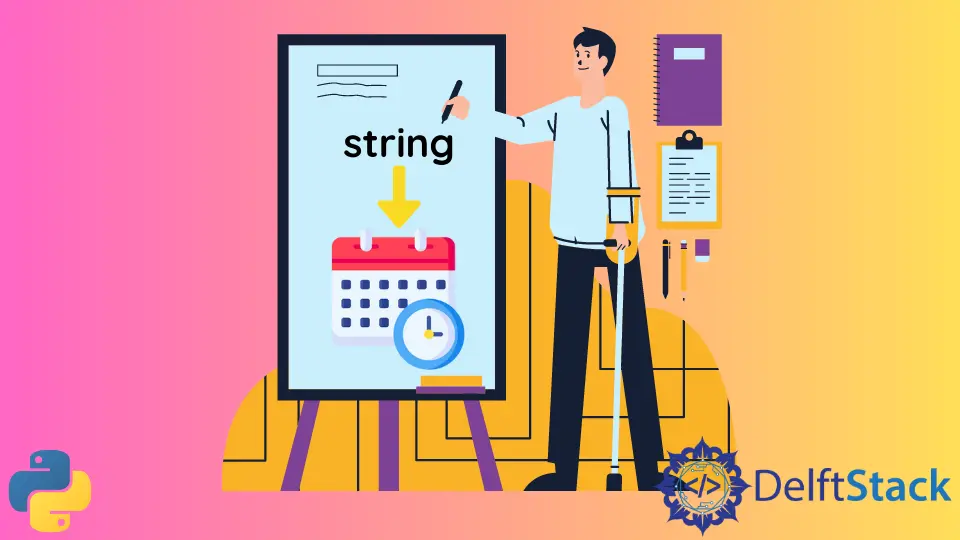
This tutorial introduces how to convert string
to datetime
in Python and also lists some example codes to cover popular datetime
string
formats.
datetime.strptime()
to Convert String to Datetime
In the previous tutorial, we have learned how to get the datetime in string format by using the datetime.strftime()
method. We will use the datetime.strptime()
method to do the revere conversion to get the datetime
object. f
and p
in these two methods mean format
and parse
respectively.
datetime.strptime()
method parses the input string with the given datetime
format and returns the datetime
object.
The basis example to use this strptime()
method is shown below,
from datetime import datetime
datetime.strptime("2018-01-31", "%Y-%m-%d")
The result will be
datetime.datetime(2018, 1, 31, 0, 0)
The directives of the string format codes are listed here for your reference
Directive | Meaning | Example |
---|---|---|
%a |
Weekday as locale’s abbreviated name. | Sun, Mon, …, Sat (en_US);So, Mo, …, Sa (de_DE) |
%A |
Weekday as locale’s full name. | Sunday, Monday, …, Saturday (en_US);Sonntag, Montag, …, Samstag (de_DE) |
%w |
Weekday as a decimal number, where 0 is Sunday and 6 is Saturday. | 0, 1, …, 6 |
%d |
Day of the month as a zero-padded decimal number. | 01, 02, …, 31 |
%b |
Month as locale’s abbreviated name. | Jan, Feb, …, Dec (en_US);Jan, Feb, …, Dez (de_DE) |
%B |
Month as locale’s full name. | January, February, …, December (en_US);Januar, Februar, …, Dezember (de_DE) |
%m |
Month as a zero-padded decimal number. | 01, 02, …, 12 |
%y |
Year without century as a zero-padded decimal number. | 00, 01, …, 99 |
%Y |
Year with century as a decimal number. | 0001, 0002, …, 2013, 2014, …, 9998, 9999 |
%H |
Hour (24-hour clock) as a zero-padded decimal number. | 00, 01, …, 23 |
%I |
Hour (12-hour clock) as a zero-padded decimal number. | 01, 02, …, 12 |
%p |
Locale’s equivalent of either AM or PM. | AM, PM (en_US);am, pm (de_DE) |
%M |
Minute as a zero-padded decimal number. | 00, 01, …, 59 |
%S |
Second as a zero-padded decimal number. | 00, 01, …, 59 |
%f |
Microsecond as a decimal number, zero-padded on the left. | 000000, 000001, …, 999999 |
%z |
UTC offset in the form ±HHMM[SS](empty string if the object is naive). | (empty), +0000, -0400, +1030 |
%Z |
Time zone name (empty string if the object is naive). | (empty), UTC, EST, CST |
%j |
Day of the year as a zero-padded decimal number. | 001, 002, …, 366 |
%U |
Week number of the year (Sunday as the first day of the week) as a zero padded decimal number. All days in a new year preceding the first Sunday are considered to be in week 0. | 00, 01, …, 53 |
%W |
Week number of the year (Monday as the first day of the week) as a decimal number. All days in a new year preceding the first Monday are considered to be in week 0. | 00, 01, …, 53 |
%c |
Locale’s appropriate date and time representation. | Tue Aug 16 21:30:00 1988 (en_US);Di 16 Aug 21:30:00 1988 (de_DE) |
%x |
Locale’s appropriate date representation. | 08/16/88 (None);08/16/1988 (en_US);16.08.1988 (de_DE) |
%X |
Locale’s appropriate time representation. | 21:30:00 (en_US);21:30:00 (de_DE) |
%% |
A literal '%' character. |
% |
Python datetime.strptime()
Examples
7-May-2013
datetime
Format
from datetime import datetime
dateString = "7-May-2018"
dateFormatter = "%u-%b-%Y"
datetime.strptime(dateString, dateFormatter)
Output:
datetime.datetime(2018, 5, 1, 0, 0)
31/12/2018
datetime
Format
from datetime import datetime
dateString = "31/12/2013"
dateFormatter = "%d/%m/%Y"
datetime.strptime(dateString, dateFormatter)
Output:
datetime.datetime(2013, 12, 31, 0, 0)
Mon, July 16 2018
datetime
Format
from datetime import datetime
dateString = "31/12/2013"
dateFormatter = "%d/%m/%Y"
datetime.strptime(dateString, dateFormatter)
Output:
datetime.datetime(2013, 12, 31, 0, 0)
Monday, July 16, 2018 20:01:56
datetime
Format
from datetime import datetime
dateString = "Monday, July 16, 2018 20:01:56"
dateFormatter = "%A, %B %d, %Y %H:%M:%S"
datetime.strptime(dateString, dateFormatter)
Output:
datetime.datetime(2018, 7, 16, 20, 1, 56)
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookRelated Article - Python String
- How to Remove Commas From String in Python
- How to Check a String Is Empty in a Pythonic Way
- How to Convert a String to Variable Name in Python
- How to Remove Whitespace From a String in Python
- How to Extract Numbers From a String in Python