Python datetime.strftime() Method
Musfirah Waseem
Jan 30, 2023
-
Syntax of Python
datetime.strftime()
Method -
Example 1: Use
datetime.strftime()
Method in Python -
Example 2: Convert to String Using
datetime.strftime()
Method -
Example 3: Represent Time in Different Formats Using the
datetime.strftime()
Method
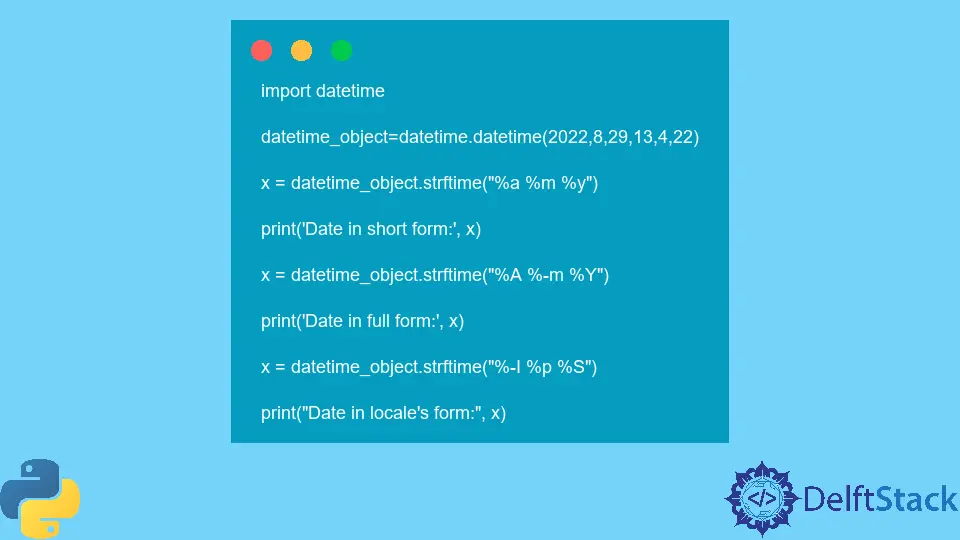
Python datetime.strftime()
method is an efficient way of converting any stated datetime
object to different string formats.
Syntax of Python datetime.strftime()
Method
datetime.strftime(format)
Parameters
Description | Example | |
---|---|---|
format |
It is the data in datetime format, which is then converted using the following codes: |
|
%a |
Abbreviated weekday name | Sun, Mon, Tue, Wed |
%A |
Full weekday name | Sunday, Monday |
%w |
Weekday as decimal number | 0,1,2,3,4,5,6 |
%d |
Day as a zero-padded decimal | 01,02,03,04,05,06… |
%-d |
Day as a decimal number | 1,2,3,4,5,6… |
%b |
Abbreviated month name | Jan, Feb, Mar |
%m |
Months as zero-padded decimal | 01,02,03,04,05,06… |
%-m |
Month number as a decimal | 1,2,3,4,5,6… |
%B |
Full month name | January, February.. |
%y |
Year without century, zero-padded decimal | 99,00,01.. |
%-y |
Year without century as a decimal | 99,0,1.. |
%Y |
Year with century | 1999,2000,2001.. |
%H |
24-hour clock, zero-padded decimal | 01,02,….23 |
%-H |
24-hour clock as a decimal | 1,2,3,….23 |
%I |
12-hour clock, zero-padded decimal | 01,02,….12 |
%-I |
12-hour clock as a decimal | 1,2,3,….12 |
%M |
Minutes as a zero-padded decimal | 01,02,….59 |
%-M |
Minutes as a decimal | 1,2,3,….59 |
%S |
Seconds as a zero-padded decimal | 01,02,….59 |
%-S |
Seconds as a decimal | 1,2,3,….59 |
%p |
locale’s AM or PM | AM, PM |
%Z |
Timezone name | est,utc,gmt… |
%z |
UTC offset in the form +HHMM or -HHMM |
+0112,-0112…. |
%j |
Day number of a year, zero-padded decimal | 001,002,….365 |
%-j |
Day number in a year as a decimal | 1,2,3,….365 |
%c |
locale’s date and time representation | Mon Aug 29 07:06:05 2022 |
%x |
locale’s date representation | 29/08/22 |
%X |
locale’s time representation | 10:03:43 |
%f |
microsecond, zero-padded on the left side | 000000…. 999999 |
%U |
Week number of a year (Sunday as first) | 0,1,2…6 |
%W |
Week number of a year | 00,01,…53 |
Return
This method’s return type is a string representing date and time. It uses date
, time
or datetime
objects.
Example 1: Use datetime.strftime()
Method in Python
import datetime
datetime_object = datetime.datetime(2022, 8, 29, 12, 30, 5)
year = datetime_object.strftime("%Y")
print("year:", year)
month = datetime_object.strftime("%m")
print("month:", month)
day = datetime_object.strftime("%d")
print("day:", day)
time = datetime_object.strftime("%H:%M:%S")
print("time:", time)
date_time = datetime_object.strftime("%m/%d/%Y, %H:%M:%S")
print("date and time:", date_time)
Output:
year: 2022
month: 08
day: 29
time: 12:30:05
date and time: 08/29/2022, 12:30:05
The above code shows only the attributes which we have specified.
Example 2: Convert to String Using datetime.strftime()
Method
import datetime
timestamp = 1528797322
datetime_object = datetime.datetime.fromtimestamp(timestamp)
x = datetime_object.strftime("%c")
print("Date and time: ", x)
x = datetime_object.strftime("%x")
print("Date:", x)
x = datetime_object.strftime("%X")
print("Time:", x)
x = datetime_object.strftime("%I%p")
print("Locale's AM or PM:", x)
Output:
Date and time: Tue Jun 12 09:55:22 2018
Date: 06/12/18
Time: 09:55:22
Locale's AM or PM: 09AM
Based on the string and the format code used, such as %d
, %B
, the method returns its equivalent datetime
object.
Example 3: Represent Time in Different Formats Using the datetime.strftime()
Method
import datetime
datetime_object = datetime.datetime(2022, 8, 29, 13, 4, 22)
x = datetime_object.strftime("%a %m %y")
print("Date in short form:", x)
x = datetime_object.strftime("%A %-m %Y")
print("Date in full form:", x)
x = datetime_object.strftime("%-I %p %S")
print("Date in locale's form:", x)
Output:
Date in short form: Mon 08 22
Date in full form: Monday 8 2022
Date in locale's form: 1 PM 22
In the above code, we got the time following a format we specified using format codes.
Author: Musfirah Waseem
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn