Python datetime.timezone Class
- Syntax
-
Methods of Python
datetime.timezone
Class -
Example 1: Timezone-Aware
datetime
Objects -
Example 2: Timezone-Aware
time
Objects
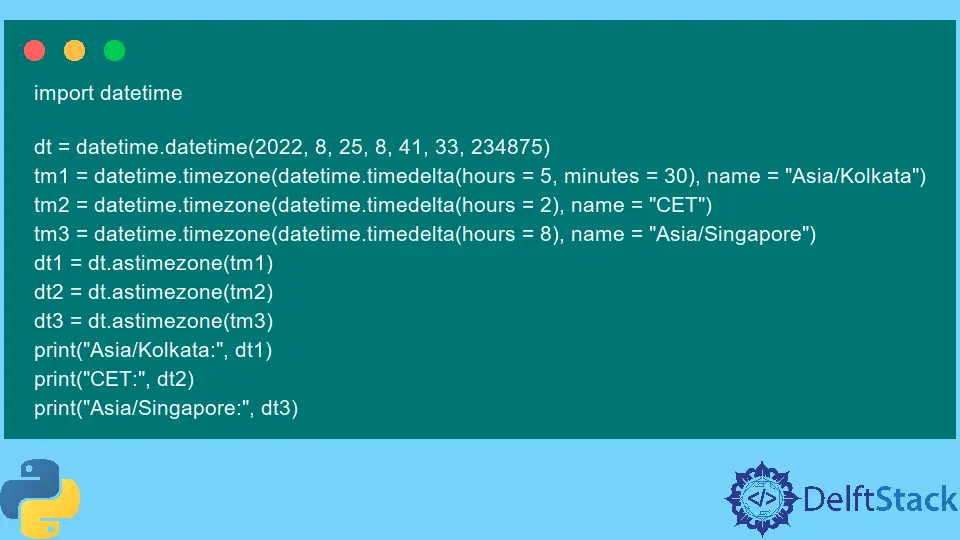
The datetime
module is a built-in module available in Python programming language. This library offers several classes to represent quantities related to date and time, such as date
to represent a date, datetime
to represent date and time combined, timedelta
to represent a time difference, and timezone
to describe a timezone.
The timezone
class is a subclass of the tzinfo
class, which is an abstract class and can only be inherited. To learn about the tzinfo
class, refer to the official documentation here.
The tzinfo
class fails at representing timezones of landmarks where different time offsets are used for different days of a year. Moreover, it cannot represent timezones of locations where historical changes have been made to civil time.
The timezone
class implements 5
important methods and 1
attributes, which are as follows.
Syntax
datetime.timezone()
Parameters
Type | Details | |
---|---|---|
offset |
timedelta |
The UTC offset for the timezone is expressed as a timedelta object. |
name |
String | A name for the timezone. |
Returns
Since it is a class, it doesn’t return anything, but its constructor creates a timezone
object.
Methods of Python datetime.timezone
Class
The abstract class tzinfo
contains five methods the inheriting class has to implement. Luckily, the timezone
class implements them, and they are as follows.
timezone(offset, name=None)
The timezone
is a constructor used to create class timezone
instances. This method accepts two parameters.
offset
: Time offset for a timezone. It should be of typetimedelta
. To read more abouttimedelta
, refer to the official documentation here.name
: A string name for the timezone. By default, it is set toNone
.
Note that the timedelta
should be between -timedelta(hours = 24)
and timedelta(hours = 24)
; otherwise, a ValueError
is raised.
Since timezone
is a subclass of the abstract class tzinfo
, we can use its objects to create timezone-aware datetime
objects. Refer to the following Python code.
import datetime
dt = datetime.datetime(2022, 8, 25, 8, 41, 33, 234875)
tm1 = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="Asia/Kolkata")
tm2 = datetime.timezone(datetime.timedelta(hours=2), name="CET")
tm3 = datetime.timezone(datetime.timedelta(hours=8), name="Asia/Singapore")
dt1 = dt.astimezone(tm1)
dt2 = dt.astimezone(tm2)
dt3 = dt.astimezone(tm3)
print("Asia/Kolkata:", dt1)
print("CET:", dt2)
print("Asia/Singapore:", dt3)
Output:
Asia/Kolkata: 2022-08-25 14:11:33.234875+05:30
CET: 2022-08-25 10:41:33.234875+02:00
Asia/Singapore: 2022-08-25 16:41:33.234875+08:00
timezone.utcoffset(dt)
This method returns the timezone offset or the UTC offset of a timezone
object. Note that the dt
argument is mandatory and ignored internally.
So, we can pass a None
value for it. This method returns a timedelta
object whose value equals the difference between local time and UTC.
Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 8, 25, 8, 41, 33, 234875)
tm1 = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="Asia/Kolkata")
tm2 = datetime.timezone(datetime.timedelta(hours=2), name="CET")
tm3 = datetime.timezone(datetime.timedelta(hours=8), name="Asia/Singapore")
dt1 = dt.astimezone(tm1)
dt2 = dt.astimezone(tm2)
dt3 = dt.astimezone(tm3)
print("Asia/Kolkata:", dt1)
print("CET:", dt2)
print("Asia/Singapore:", dt3)
print("\nUTC Offsets\n")
print(tm1.utcoffset(None)) # here
print(tm2.utcoffset(None)) # here
print(tm3.utcoffset(None)) # here
Output:
Asia/Kolkata: 2022-08-25 14:11:33.234875+05:30
CET: 2022-08-25 10:41:33.234875+02:00
Asia/Singapore: 2022-08-25 16:41:33.234875+08:00
UTC Offsets
5:30:00
2:00:00
8:00:00
timezone.tzname(dt)
This method returns the name of the timezone set in the timezone
object. If no value is assigned to the constructor, it is set to None
.
For a None
value, the name of the timezone is resolved by tzname(dt)
using the offset
. If the offset is timedelta(0)
, the name returned is "UTC"
.
For other cases, the following format is followed.
UTC±HH:MM
Where ±
is the sign of the offset, and HH
and MM
are two digits of offset.hours
and offset.minutes
, respectively.
Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 8, 25, 8, 41, 33, 234875)
tm1 = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="Asia/Kolkata")
tm2 = datetime.timezone(datetime.timedelta(hours=2), name="CET")
tm3 = datetime.timezone(datetime.timedelta(hours=8), name="Asia/Singapore")
dt1 = dt.astimezone(tm1)
dt2 = dt.astimezone(tm2)
dt3 = dt.astimezone(tm3)
print("Asia/Kolkata:", dt1)
print("CET:", dt2)
print("Asia/Singapore:", dt3)
print("\nTimezone Names\n")
print(tm1.tzname(None))
print(tm2.tzname(None))
print(tm3.tzname(None))
Output:
Asia/Kolkata: 2022-08-25 14:11:33.234875+05:30
CET: 2022-08-25 10:41:33.234875+02:00
Asia/Singapore: 2022-08-25 16:41:33.234875+08:00
Timezone Names
Asia/Kolkata
CET
Asia/Singapore
timezone.dst(dt)
This method does not do anything; it always returns None
. Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 8, 25, 8, 41, 33, 234875)
tm1 = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="Asia/Kolkata")
tm2 = datetime.timezone(datetime.timedelta(hours=2), name="CET")
tm3 = datetime.timezone(datetime.timedelta(hours=8), name="Asia/Singapore")
dt1 = dt.astimezone(tm1)
dt2 = dt.astimezone(tm2)
dt3 = dt.astimezone(tm3)
print("Asia/Kolkata:", dt1)
print("CET:", dt2)
print("Asia/Singapore:", dt3)
print("\nDST")
print(tm1.dst(None))
print(tm2.dst(None))
print(tm3.dst(None))
Output:
Asia/Kolkata: 2022-08-25 14:11:33.234875+05:30
CET: 2022-08-25 10:41:33.234875+02:00
Asia/Singapore: 2022-08-25 16:41:33.234875+08:00
DST
None
None
None
timezone.fromutc(dt)
This method returns dt + offset
. Note that the dt
must be of a datetime
object, and its tzinfo
should be set to self
.
Refer to the following Python code for an example.
import datetime
dt = datetime.datetime(2022, 8, 25, 8, 41, 33, 234875)
tm1 = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="Asia/Kolkata")
tm2 = datetime.timezone(datetime.timedelta(hours=2), name="CET")
tm3 = datetime.timezone(datetime.timedelta(hours=8), name="Asia/Singapore")
dt1 = dt.astimezone(tm1)
dt2 = dt.astimezone(tm2)
dt3 = dt.astimezone(tm3)
print("Asia/Kolkata:", dt1)
print("CET:", dt2)
print("Asia/Singapore:", dt3)
print("\nFrom UTC\n")
print(tm1.fromutc(dt1))
print(tm2.fromutc(dt2))
print(tm3.fromutc(dt3))
Output:
Asia/Kolkata: 2022-08-25 14:11:33.234875+05:30
CET: 2022-08-25 10:41:33.234875+02:00
Asia/Singapore: 2022-08-25 16:41:33.234875+08:00
From UTC
2022-08-25 19:41:33.234875+05:30
2022-08-25 12:41:33.234875+02:00
2022-08-26 00:41:33.234875+08:00
timezone.utc
This attribute stores the UTC or Coordinated Universal Time. More formally, it stores the value of timezone(timedelta(0))
.
Refer to the following Python code for an example.
import datetime
print(datetime.timezone.utc)
Output:
UTC
Example 1: Timezone-Aware datetime
Objects
import datetime
dt = datetime.datetime(2022, 9, 11, 12, 45, 59, 993842)
tm = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="IST")
dtAware = dt.astimezone(tm)
print("Naive DateTime Object:", dt)
print("Timezone-Aware DateTime Object:", dtAware)
Output:
Naive DateTime Object: 2022-09-11 12:45:59.993842
Timezone-Aware DateTime Object: 2022-09-11 18:15:59.993842+05:30
The Python code above first creates a naive datetime
object. Next, with the help of the timezone
class, it creates the IST timezone, which is UTC+05:30
.
Next, using the astimezone()
method available on a datetime
object, it creates a new datetime
object which is timezone-aware. Note that the date and time values are adjusted according to the timezone in the new object.
Since IST is 5
hours and 30
minutes ahead of UTC, the time is incremented by 5
hours and 30
minutes.
Example 2: Timezone-Aware time
Objects
import datetime
tm = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="IST")
t = datetime.time(12, 45, 59, 993842, tm)
print("Timezone-Aware Time Object:", t)
Output:
Timezone-Aware DateTime Object: 12:45:59.993842+05:30
The Python code above first creates the IST timezone using the timezone
class. Next, it creates a timezone-aware time
object using the time
class.