Python datetime.datetime.replace() Method
- Syntax
- Example 1: Modify Date, Time, and Timezone Details
-
Example 2: Convert a Timezone-Aware
datetime
to a Naive Instance
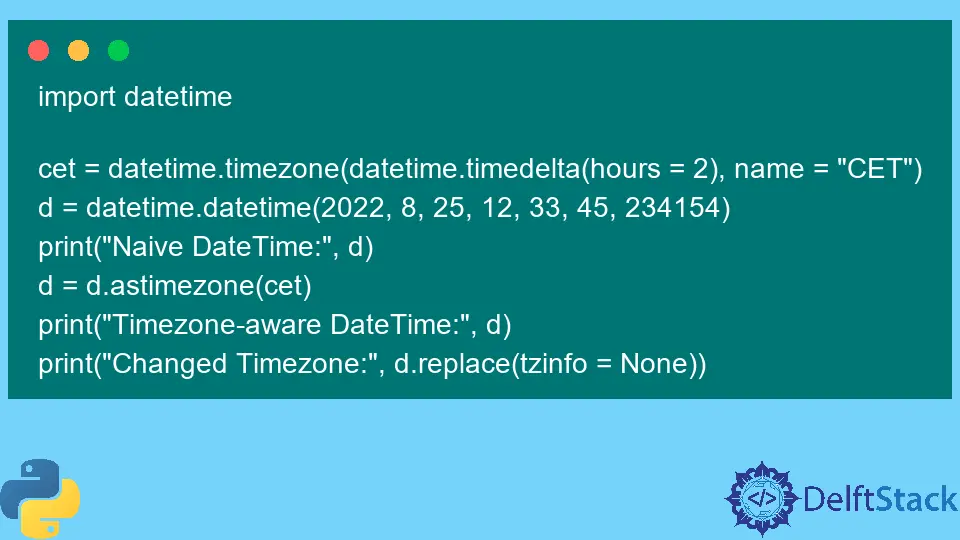
Python is a dynamic and famed general-purpose language. It is enriched with various use cases and support libraries and has a ton of support from its developers.
It offers a datetime
module, which is built-in, that makes working with date, time, and timezones a piece of cake. This module provides methods and classes such as date
, datetime
, tzinfo
, timezone
, and timedelta
to work with date and time.
These classes are flooded with numerous functions and attributes and offer robust features.
A date, along with time, is known as DateTime
. Creating datetime
objects can sometimes be tedious because they accept many values such as hour
, day
, minutes
, timezone
, seconds
, etc.
Instead of manually creating a new datetime
object, we can use an existing object, tweak its values according to our needs and generate a new datetime
object.
Every datetime
object owns a replace()
that allows us to specify new values for its attributes and generate a new datetime
object with those values. In this article, we will dive deep into the replace()
method and thoroughly understand it with the help of some relevant examples.
Syntax
datetime.replace(
year=self.year,
month=self.month,
day=self.day,
hour=self.hour,
minute=self.minute,
second=self.second,
microsecond=self.microsecond,
tzinfo=self.tzinfo,
fold=0,
)
Parameters
Type | Explanation | |
---|---|---|
year |
Integer | A year value. |
month |
Integer | A month value between 1 and 12, inclusive. |
day |
Integer | A day value between 1 and 31, inclusive. |
hour |
Integer | An hour value between 0 and 23, inclusive. |
minute |
Integer | A minute value between 0 and 59, inclusive. |
second |
Integer | A second value between 0 and 59, inclusive. |
microsecond |
Integer | A microsecond value between 0 and 999999, inclusive. |
tzinfo |
A subclass of datetime.tzinfo |
A concrete class subclassing the abstract datetime.tzinfo class. |
fold |
Integer | A binary field representing an invalid date. Here, 1 means an invalid date. |
Returns
The replace()
method returns a new datetime
object with all the same attributes as the original object, except for those attributes for which new values were provided.
Note that if tzinfo
is set to None
, a timezone-unaware datetime
object is returned for which no conversions are performed for date and time.
By default, for naive or timezone-unaware objects, date and time are stored according to UTC or Coordinated Universal Time.
Example 1: Modify Date, Time, and Timezone Details
import datetime
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
ist = datetime.timezone(datetime.timedelta(hours=5, minutes=30), name="India")
d = datetime.datetime(2022, 8, 25, 12, 33, 45, 234154)
print("Naive DateTime:", d)
d = d.astimezone(cet)
print("Timezone-aware DateTime:", d)
print("Changed Date:", d.replace(year=2001, month=5, day=20))
print("Changed Time:", d.replace(hour=10, minute=11, second=30, microsecond=0))
print("Changed Timezone:", d.replace(tzinfo=ist))
Output:
Naive DateTime: 2022-08-25 12:33:45.234154
Timezone-aware DateTime: 2022-08-25 14:33:45.234154+02:00
Changed Date: 2001-05-20 14:33:45.234154+02:00
Changed Time: 2022-08-25 10:11:30+02:00
Changed Timezone: 2022-08-25 14:33:45.234154+05:30
The Python code above first creates a datetime
object. It also creates two timezone objects, the CET timezone (+02:00
) and the IST timezone (+05:30
).
The naive datetime
is then converted to a timezone-aware object using the astimezone()
method. The timezone is set as CET, and the date and time values are adjusted accordingly.
Next, using the replace()
method, the date, time, and timezone are updated. Note that when the timezone is updated to the IST timezone, the date and time values are not adjusted according to it, unlike the astimezone()
method; they remain the same as before.
Example 2: Convert a Timezone-Aware datetime
to a Naive Instance
import datetime
cet = datetime.timezone(datetime.timedelta(hours=2), name="CET")
d = datetime.datetime(2022, 8, 25, 12, 33, 45, 234154)
print("Naive DateTime:", d)
d = d.astimezone(cet)
print("Timezone-aware DateTime:", d)
print("Changed Timezone:", d.replace(tzinfo=None))
Output:
Naive DateTime: 2022-08-25 12:33:45.234154
Timezone-aware DateTime: 2022-08-25 14:33:45.234154+02:00
Changed Timezone: 2022-08-25 14:33:45.234154
The Python code above first creates a datetime
object. This object is naive (it doesn’t know anything about the timezone; there is no ±HH:MM
value).
Next, we create a new timezone-aware object using the astimezone()
method of the object. This method accepts an object of a class subclassing the abstract datetime.tzinfo
class and returns a new datetime
object.
We set the timezone to CET or Central European Time. If we carefully observe the output, we will find that the time has changed by 2
hours; from 12:33:45.234154
to 14:33:45.234154+02:00
, and it has a timezone as well.
The CET timezone is 2
hours ahead of the UTC. Next, using the replace()
method, we set the timezone to None
.
Note that now the datetime
object is once again naive, but the output tells a different story. The date and time values were not reverted to UTC-based values; the timezone changed (14:33:45.234154+02:00
to 14:33:45.234154
).