Python Datetime.fromtimestamp() Method
-
Syntax of Python
datetime.fromtimestamp()
Method -
Example Codes: Working With the
datetime.fromtimestamp()
Method -
Example Codes: Enter Different Timestamps in the
datetime.fromtimestamp()
Method -
Example Codes: Represent a Timestamp in Different Formats Using the
datetime.fromtimestamp()
Method
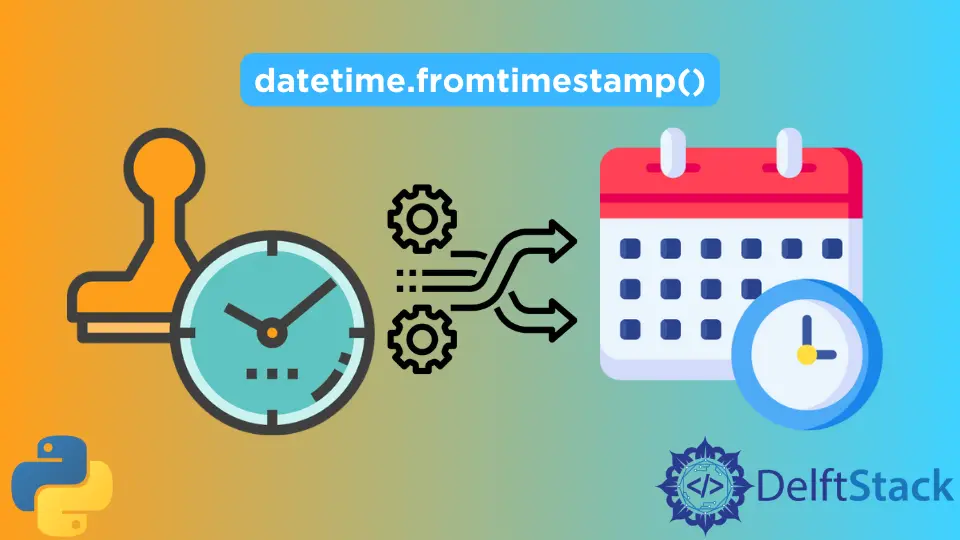
Python datetime.fromtimestamp()
method is an efficient way of converting a timestamp into a DateTime object. A timestamp is encoded information representing the date and time at which a particular event occurred.
Syntax of Python datetime.fromtimestamp()
Method
datetime.fromtimestamp(datetime_object, tz)
Parameters
datetime_object |
A date and time object that needs to be converted into a timestamp instance. |
tz |
An optional parameter with a default value of None. It represents the timezone in which we want to convert our DateTime object. |
Return
The return type of this method is an object called dt_object.
It returns the local date and time (DateTime object).
Example Codes: Working With the datetime.fromtimestamp()
Method
from datetime import datetime
timestamp = 1545830077
datetime_object = datetime.fromtimestamp(timestamp)
print("The date and time are ", datetime_object)
print("type(dt_object) =", type(datetime_object))
Output:
The date and time are 2018-12-26 13:14:37
type(dt_object) = <class 'datetime.datetime'>
Note that the above code can be used to find any date and time using just a timestamp.
Example Codes: Enter Different Timestamps in the datetime.fromtimestamp()
Method
from datetime import datetime
timestamp = -67130984480.0
datetime_object = datetime.fromtimestamp(timestamp)
print("The date and time are ", datetime_object)
timestamp = 303059844320.0
datetime_object = datetime.fromtimestamp(timestamp)
print("The date and time are ", datetime_object)
Output:
Traceback (most recent call last):
File "main.py", line 5, in <module>
datetime_object = datetime.fromtimestamp(timestamp)
ValueError: year -158 is out of range
Traceback (most recent call last):
File "main.py", line 5, in <module>
datetime_object = datetime.fromtimestamp(timestamp)
ValueError: year 11573 is out of range
The above code raises an OverflowError
or OSError
exception for times far in the past or far in the future. The above code is tested on a UNIX system.
Example Codes: Represent a Timestamp in Different Formats Using the datetime.fromtimestamp()
Method
from datetime import datetime
timestamp = 1725309972.357546
datetime_object = datetime.fromtimestamp(timestamp)
string_datetime = datetime_object.strftime("%d-%m-%Y, %H:%M:%S")
print("Format 1:", string_datetime)
string_date = datetime_object.strftime("%d %B, %Y")
print("Format 2:", string_date)
string_time = datetime_object.strftime("%I%p %M:%S")
print("Format 3:", string_time)
Output:
Format 1: 02-09-2024, 20:46:12
Format 2: 02 September, 2024
Format 3: 08PM 46:12
The above code can easily convert the timestamp to any format.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn