How to Get the Day of the Week in Python
-
Use the
weekday()
Method to Get the Name of the Day in Python -
Use the
isoweekday()
Method to Get the Name of the Day in Python -
Use the
calendar
Module to Get the Name of the Day in Python -
Use the
strftime()
Method to Get the Name of the Day in Python -
Use Pandas
Timestamp
Method to Get the Name of the Day in Python - Conclusion
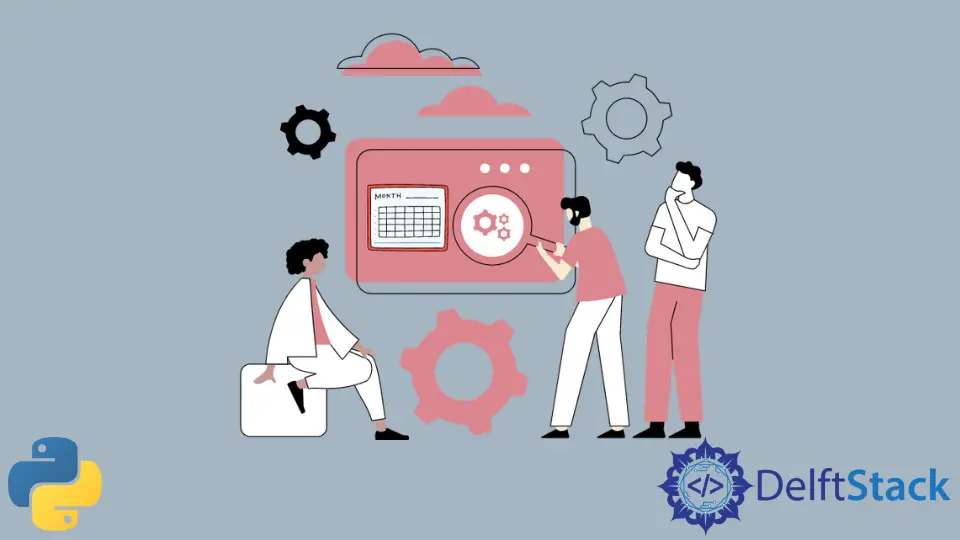
In the world of Python programming, understanding and manipulating dates and times is a fundamental skill. This article explores different methods to obtain the name of the day of the week in Python.
Whether you’re working with Python’s built-in datetime
module, the calendar
module, or the versatile pandas
library, you’ll learn how to extract this essential piece of temporal information. These methods offer flexibility and can be applied in various scenarios, from scheduling tasks to date-related calculations.
Use the weekday()
Method to Get the Name of the Day in Python
Before we can start working with dates, we need to import the datetime
module. This module provides classes and functions for manipulating dates and times.
Here’s how to import it:
from datetime import datetime
By importing the datetime
class from the datetime
module, we can create datetime
objects, which represent specific points in time.
To get the day of the week from a given date, we first need to create a datetime
object that represents that date. You can do this by using the datetime
constructor and specifying the year, month, and day as arguments.
For example, to create a datetime
object for October 27, 2023
, you would use the following code:
date = datetime(2023, 10, 27)
Now, you have a datetime
object named date
that represents the specified date.
The datetime
module provides the weekday()
method, which can be called on a datetime
object to obtain the day of the week. The weekday()
method returns an integer, where Monday is 0
and Sunday is 6
.
Here’s how you can use it:
day_of_week = date.weekday()
Now, the variable day_of_week
contains an integer representing the day of the week for the given date. You can use this integer to perform various tasks or simply display the day of the week.
Here’s a complete example of how to get the day of the week from a given date in Python using the datetime
module:
from datetime import datetime
date = datetime(2023, 10, 27)
day_of_week = date.weekday()
days = ["Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday"]
day_name = days[day_of_week]
print(f"The day of the week for {date.date()} is {day_name}.")
When you run this code, it will output:
The day of the week for 2023-10-27 is Friday.
Here’s another example of how to use the weekday()
method to obtain the day of the week for the current date:
from datetime import datetime
current_datetime = datetime.today()
day_of_week = current_datetime.weekday()
print(day_of_week)
Output:
1
When you run this code, you will receive an output that corresponds to the current day of the week. For instance, if you execute the code and the result is 1
, it indicates that the current day is Tuesday, as Tuesday is the second day of the week (0-based indexing).
This method is particularly useful when you need to perform date-related calculations, schedule tasks based on the day of the week, or format date information. It provides a straightforward way to work with date and time data in Python.
Keep in mind that the weekday()
method is just one of the many date and time functions available in Python’s datetime
module, which allows you to perform a wide range of operations and manipulations on dates and times, making it a valuable tool for handling temporal data in your Python applications.
Use the isoweekday()
Method to Get the Name of the Day in Python
This method works similarly to the weekday()
method. However, this is used to obtain the day of the week in the ISO week numbering system.
To begin, you also need to import the datetime
module in Python:
from datetime import datetime
Before you can use isoweekday()
, you must create a datetime
object representing the date for which you want to determine the day of the week. This can be achieved by using the datetime
constructor and providing the year, month, and day as arguments.
For example, to create a datetime
object for November 10, 2023
, you would use the following code:
date = datetime(2023, 11, 10)
Now, you have a datetime
object named date
that represents the specified date.
The datetime
module’s isoweekday()
method is used to retrieve the day of the week in the ISO week numbering system. This method returns an integer where Monday is 1
and Sunday is 7
.
To utilize it, simply call the isoweekday()
method on a datetime
object, as shown below:
day_of_week_iso = date.isoweekday()
After executing this line of code, the variable day_of_week_iso
will contain an integer representing the day of the week in the ISO system.
Here’s a complete example of how to get the day of the week from a given date in Python using the isoweekday()
method:
from datetime import datetime
date = datetime(2023, 11, 10)
day_of_week_iso = date.isoweekday()
days_iso = [
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday",
"Sunday",
]
day_name_iso = days_iso[day_of_week_iso - 1]
print(f"The ISO day of the week for {date.date()} is {day_name_iso}.")
To make the result more human-readable, we create a list called days_iso
containing the names of the days of the week in the correct order. We then use the day_of_week_iso
integer to index this list and retrieve the corresponding day name.
The day name is stored in the variable day_name_iso
.
Finally, we print the result using an f-string, displaying a message that includes the ISO day of the week for the specified date. In this specific case, when you run the code, it will output:
The ISO day of the week for 2023-11-10 is Friday.
This means that November 10, 2023
, falls on a Friday, according to the ISO week numbering system. The code demonstrates how to use the isoweekday()
method in Python to extract this information and present it in a user-friendly format.
Let’s have another example:
from datetime import datetime
print(datetime.today().isoweekday())
When you run this code, it will produce the following output:
2
Here, the datetime.today()
function is used to obtain the current date and time as a datetime
object. The .isoweekday()
method is then applied to this datetime
object.
In the provided example, the output is 2
, which is equivalent to Tuesday, as Monday is represented as 1
.
Use the calendar
Module to Get the Name of the Day in Python
When you need to find the name of the day of the week for a given date in English, Python provides a convenient solution using the calendar
module. This module offers a straightforward approach through the day_name()
method, which efficiently manages an array of the days of the week.
In this array, Monday is positioned at the 0th index, making it simple to obtain the name of the day of the week for a specific date.
To get started, you need to import the necessary modules, which include the datetime
module for handling dates and the calendar
module to work with the days of the week. Here’s how you can import them:
from datetime import date
import calendar
The datetime
module enables you to work with dates and times, while the calendar
module provides functions and data structures for managing various aspects of calendars, including the names of the days of the week.
Before determining the day of the week for a given date, you must create a date
object that represents that specific date. You can do this using the date()
constructor from the datetime
module.
For instance, to create a date
object for May 20, 2023
, you can use the following code:
curr_date = date(2023, 5, 20)
Now, the curr_date
variable holds a date
object representing May 20, 2023
.
The key to obtaining the day of the week in English is the day_name()
method provided by the calendar
module. This method efficiently manages an array of the names of the days of the week.
The name of the day corresponding to a specific date can be retrieved by providing the index obtained from the weekday()
method on the date
object. The weekday()
method returns an integer where Monday is 0
, Tuesday is 1
, and so on.
The day_name()
method is then used to fetch the English name of the day.
Here’s an example of how to use this method:
day_name = calendar.day_name[curr_date.weekday()]
The day_name
variable will now hold the English name of the day corresponding to the date represented by curr_date
.
Let’s put it all together with a full example:
from datetime import date
import calendar
curr_date = date(2023, 5, 20)
day_name = calendar.day_name[curr_date.weekday()]
print(f"The day of the week for {curr_date} is {day_name}.")
When you run this code, it will output:
The day of the week for 2023-05-20 is Saturday.
This code demonstrates how to effectively use Python’s calendar
module to determine and display the name of the day of the week in English for a given date. It’s a practical and intuitive approach that can be applied in a variety of applications, such as scheduling tasks, date-related calculations, and more.
Use the strftime()
Method to Get the Name of the Day in Python
When you need to retrieve the name of the day of the week in English in Python, the strftime()
method provides an alternative and flexible approach. This method, part of Python’s datetime
module, is commonly used for formatting dates and times.
In this case, we can leverage the %A
directive within strftime()
to obtain the full name of the weekday.
The %A
directive is a format code used with the strftime()
method, and it returns the full name of the weekday. It allows you to easily extract the English name of the day for a specific date.
For instance, when you utilize %A
, the method will return Monday, Tuesday, Wednesday, and so on, depending on the date you provide.
Before working with the strftime()
method, you must import the datetime
module. Here’s how to do that:
from datetime import datetime
This module provides functions and classes to handle dates and times, including the strftime()
method.
Let’s look at a practical example of how to use the strftime()
method to get the English name of the day of the week:
from datetime import datetime
english_day_name = datetime.today().strftime("%A")
print(english_day_name)
When you execute this code, it will produce the following output:
Tuesday
In this example, we employ the %A
directive with strftime()
to obtain the full name of the weekday for the current date. The resulting english_day_name
variable will store the English name of the day.
Here’s another example of how to get the English name of the day of the week for a specific date using strftime()
:
from datetime import datetime
specific_date = datetime(2023, 10, 27)
english_day_name = specific_date.strftime("%A")
print(f"The English name of the day for {specific_date.date()} is {english_day_name}.")
In this example, we first create a datetime
object named specific_date
representing October 27, 2023
. We then use the strftime()
method with the %A
directive to obtain the full name of the weekday for that specific date.
The resulting english_day_name
variable will hold the English name of the day (e.g., Monday, Tuesday, Wednesday, etc.).
When you run this code, it will output:
The English name of the day for 2023-10-27 is Friday.
This demonstrates how to extract the English name of the day of the week for any given date using the strftime()
method in Python.
Use Pandas Timestamp
Method to Get the Name of the Day in Python
Using the Timestamp
method from pandas
, you can also efficiently extract the day of the week from a given date.
Before you can utilize pandas
and its Timestamp
method, you need to import the library. Here’s how to do that:
import pandas as pd
The pandas
library is a powerful tool for data manipulation and analysis, and it includes functions for handling date and time data.
To extract the day of the week from a given date, you first need to create a Timestamp
object that represents that date. The Timestamp
constructor accepts a date in the YYYY-MM-DD
format as its argument.
Here’s an example of how to create a Timestamp
object for a specific date:
# Create a `Timestamp` object for a specific date
date = pd.Timestamp("2023-10-27")
Now, you have a Timestamp
object named date
that represents October 27, 2023
.
The Timestamp
object in pandas
provides a convenient day_name()
method. This method returns the name of the day of the week for the date represented by the Timestamp
object. It returns the day’s name in English.
Here’s how to use it:
# Use the `day_name()` method to get the English name of the day of the week
day_name = date.day_name()
The day_name
variable now holds the English name of the day of the week for the given date.
Let’s put it all together with a complete example:
import pandas as pd
date = pd.Timestamp("2023-10-27")
day_name = date.day_name()
print(f"The day of the week for {date.date()} is {day_name}.")
When you run this code, it will output:
The day of the week for 2023-10-27 is Friday.
This example demonstrates how to efficiently use Timestamp
to extract the English name of the day of the week for a given date.
Conclusion
In Python, there are multiple ways to get the name of the day of the week, depending on your specific needs and the libraries at your disposal. You can choose from the datetime
module’s weekday()
and isoweekday()
methods, the calendar
module’s day_name()
method, or the strftime()
method for more flexible formatting.
Additionally, the pandas
library provides a convenient Timestamp
method for this purpose. Each of these methods serves as a valuable tool for working with date and time data, enabling you to effectively handle temporal information in your Python applications.
Whether you’re working with standard dates, ISO week numbering, or need to display day names in English, Python has you covered with these versatile date manipulation techniques.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn