Python datetime.weekday() Method
-
Syntax of Python
datetime.weekday()
Method -
Example 1: Use the
datetime.weekday()
Method in Python -
Example 2: Find the Day of a Week on a Random Date Using the
datetime.weekday()
Method -
Example 3: Get the Date and Time With the Day’s Name Using the
datetime.weekday()
Method
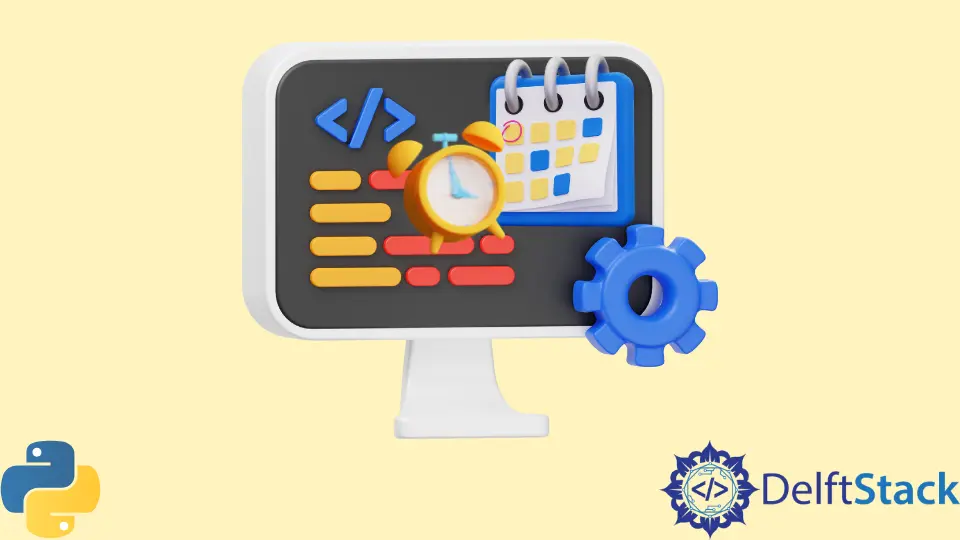
Python datetime.weekday()
method is an efficient way of finding the day of the week as an integer. Monday is represented by 0 and Sunday by 6.
It is similar to another Python function called self.date().weekday()
.
Syntax of Python datetime.weekday()
Method
datetime.weekday()
Parameters
This method doesn’t accept any parameter.
Return
The return type of this method is an integer number representing the day of the week. The following is the mapping of integer values assigned to the days of a week.
0 |
Monday |
1 |
Tuesday |
2 |
Wednesday |
3 |
Thursday |
4 |
Friday |
5 |
Saturday |
6 |
Sunday |
Example 1: Use the datetime.weekday()
Method in Python
from datetime import datetime
datetime_object = datetime.now()
print("Datetime is:", datetime_object)
dayNo = datetime_object.weekday()
print("Day of the week is:", dayNo)
Output:
Datetime is: 2022-08-30 16:14:22.871489
Day of the week is: 1
The above code shows 1
representing the day Tuesday.
Example 2: Find the Day of a Week on a Random Date Using the datetime.weekday()
Method
import datetime
datetime_object = datetime.datetime(2017, 10, 20)
print("The day of the week is:", datetime_object.weekday())
Output:
The day of the week is: 4
This method comes in handy when we want to schedule our dates according to a particular day.
Example 3: Get the Date and Time With the Day’s Name Using the datetime.weekday()
Method
import datetime
weekDays = (
"Monday",
"Tuesday",
"Wednesday",
"Thursday",
"Friday",
"Saturday",
"Sunday",
)
datetime_object = datetime.datetime(2022, 8, 22, 00, 00, 00)
dayNo = weekDays[datetime_object.weekday()]
print("{} is for {}".format(datetime_object, dayNo))
nextDay = datetime_object.replace(day=2)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
nextDay = datetime_object.replace(day=3)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
nextDay = datetime_object.replace(day=4)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
nextDay = datetime_object.replace(day=5)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
nextDay = datetime_object.replace(day=6)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
nextDay = datetime_object.replace(day=7)
dayNo = weekDays[nextDay.weekday()]
print("{} is for {}".format(nextDay, dayNo))
Output:
2022-08-22 00:00:00 is for Monday
2022-08-02 00:00:00 is for Tuesday
2022-08-03 00:00:00 is for Wednesday
2022-08-04 00:00:00 is for Thursday
2022-08-05 00:00:00 is for Friday
2022-08-06 00:00:00 is for Saturday
2022-08-07 00:00:00 is for Sunday
The above code can easily print the date and time along with its corresponding weekday name.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn