How to Determine Leap Year in Python
- Introduction to Leap Year
-
Determine Leap Year Through a Simple
if-else
Construct in Python -
Determine Leap Year Through the
Calendar
Module in Python -
Determine Leap Year Through the Conditional Operator With the
Calendar
Module in Python
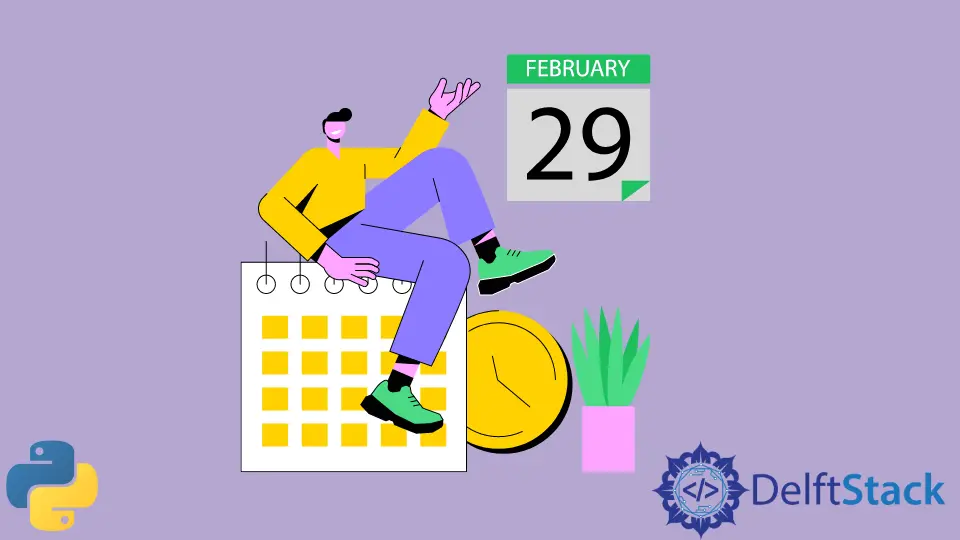
This article will discuss the terminology known as a leap year. We will also see a Python implementation to check whether a particular year is a leap year or not.
Introduction to Leap Year
The earth takes 6 more hours than 365 days to complete its circle around the sun.
Suppose we do not add one extra day in February (i.e., 29th). In that case, it will eventually cause us to see many religious and political events in different seasons (though the dates remain the same).
It suggests we add one day to every 4th year as 6 x 4 = 24
. However, it is not as simple because the extra 6 hours are not exact.
The earth completes its revolution in approximately 365 days, 5 hours, 48 mins, and 46 seconds making a difference of 11 minutes and 14 seconds from the statement discussed in the previous passage. This subtle difference makes us perform more complex calculations to keep dates in the same season.
Therefore, we say that a year must follow the following conditions to qualify for a leap year:
- Straight Disqualification: If the year under consideration is not evenly divisible by 4, it does not qualify for a leap year. For example, 2077 is not evenly divisible by 4 (i.e.,
2077 % 4 != 0
), so it is not a leap year. - If the year is evenly divisible by 4 but not evenly divisible by 100, then the year will be the leap year. For example, 2024 is properly divisible by 4 but not by 100 (i.e.,
2024 % 4 == 0
and2024 % 100 != 0
), so it qualifies for being a leap year. - If the number is evenly divisible by 4 and 100, then we will check whether the year is also divisible by 400. If yes, the year will be the leap year; otherwise, the year will not be a leap year.
For example, 4000 is evenly divisible by 4, 100, and 400, so it is a leap year. Mathematically, if year % 4 == 0
, year % 100 == 0
and year % 400 == 0
, then the year is a leap year.
The following section provides a complete Python implementation that will determine whether a year qualifies to be a leap year or not. After having a fundamental understanding of the leap year concept, let’s look at different implementations to check if a year is a leap or not.
Determine Leap Year Through a Simple if-else
Construct in Python
The following implementation uses a simple if-else
construct to determine a leap year.
- For a given year input, we first check if the second condition is satisfied; if so, we show our positive judgment (i.e., the year is a leap year).
- If the input year does not satisfy the compound condition given in line #3, we check if the input year follows the third condition and display our decision accordingly.
- Now, if the year does not follow any of the conditions mentioned above, we conclude that the year is not a leap year.
Example Code:
yearValue = int(input("Enter a year: "))
# here, yearValue means the year we want to check.
if yearValue % 4 == 0 and yearValue % 100 != 0: # condition 2
print(yearValue, "is a leap year")
elif yearValue % 4 == 0 and yearValue % 100 == 0: # condition 3
if yearValue % 400 == 0:
print(yearValue, "qualifies as a leap year")
else:
print(yearValue, "does not qualify as a leap year")
else:
print(yearValue, "does not qualify as a leap year") # Condition 1
Now, we will evaluate our program on each of the three conditions by taking different input values.
Output Evaluating the 1st Condition:
Enter a year: 2027
2027 does not qualify as a leap year
Output Evaluating the 2nd Condition:
Enter a year: 2024
2024 is a leap year
Output Evaluating the 3rd Condition:
Enter a year: 4000
4000 qualifies as a leap year
Determine Leap Year Through the Calendar
Module in Python
Python’s calendar
module is one of the reliable tools to perform computations involving dates. It follows the European convention (i.e., displays Monday as the first day of the week) and serves as Python’s equivalent to the Unix cal
program.
It provides several utilities to take date inputs and manipulations (including displaying the calendar). A detailed list of the functionalities offered by the calendar
module can be found here.
The isleap()
method is one of the functionalities of the calendar
module. Let’s look at the following example to understand the use of the calendar
module and the isleap()
method.
Example Code:
import calendar
yearValue = int(input("Enter a year: "))
if calendar.isleap(yearValue):
print(yearValue, "qualifies as a leap year")
else:
print(yearValue, "does not qualify as a leap year")
The first line of the above code imports the calendar
module. The second line takes an integer year input from the user and assigns it to the yearValue
variable.
The calendar.isLeap()
method takes an integer year value and returns true
or false
if the argument is a leap year or not. Therefore, we can apply decision statements to display our personalized messages.
Let’s test this code by running the program for different years:
First Run:
Enter a year: 2027
2027 does not qualify as a leap year
Second Run:
Enter a year: 2024
2024 qualifies as a leap year
Third Run:
Enter a year: 4000
4000 qualifies as a leap year
Determine Leap Year Through the Conditional Operator With the Calendar
Module in Python
We can combine the conditional operator with the calendar
module to achieve the same. The conditional operator is a ternary conditional construct that can be used as a replacement for a simple if-else
construct.
Example Code:
import calendar
y = int(input("Enter a year: "))
# [statement_on_True] if [condition] else [statement_on_false]
print(y, "is a leap year") if (calendar.isleap(y)) else print(y, "is not a leap year")
The above code uses the conditional operator to display whether a year is a leap year or not. The syntax of the conditional operator is well-commented in the code on line #4.
Outputs for several runs:
First Run:
Enter a year: 2027
2027 is not a leap year
Second Run:
Enter a year: 2024
2024 is a leap year
Third Run:
Enter a year: 4000
4000 is a leap year
If we want to skip using the calendar
module, we can use simple compound conditions in conjunction with the ternary conditional operator. If you’re looking for ways to explore date calculations further, you may find this article on How to Calculate Date Using Python Timedelta Months helpful.
Moreover, many other options exist to implement the desired program (e.g., match-case
construct). We let this on geeks to explore and implement different variations.