How to Calculate Date Using Python Timedelta Months
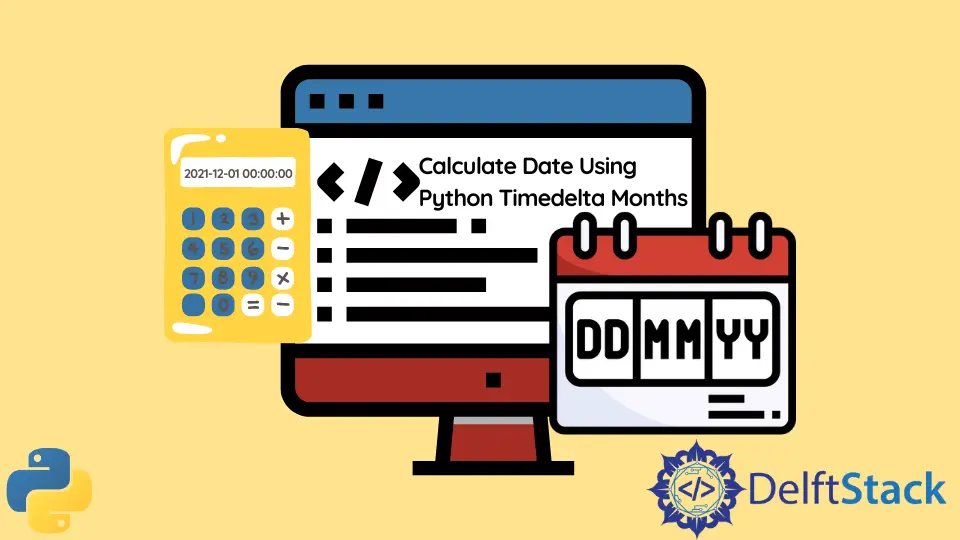
In this guide, we will learn the use of datetime
in Python using the timedelta
. We’ll see how we can calculate the date six months from the current date or any other date.
Let’s dive in!
Python datetime
Function
Well, first, we’ll look at how the datetime
function works and what’s the drawback that limits the functionalities for us. The first thing that you need to know is to import the datetime
in your code.
import datetime
After that, you will create the instance of the datetime
. Once you have created the instance, you can use its arithmetic functions.
You can subtract a day and a month. Take a look at the following code.
# instance of datetime
date = datetime.datetime(2022, 2, 1)
# subtracting 1 from the month
date = date.replace(month=date.month - 1)
print(date)
Output:
2022-01-01 00:00:00
As you can see in the above code, with the help of an arithmetic function, we have subtracted a month from the date we set earlier. But here’s a catch, what if we try to subtract a month from the above result?
The code will give us an error. Take a look.
date = date.replace(month=date.month - 1)
Output:
date = date.replace(month=date.month-1)
ValueError: month must be in 1..12
The datetime
function doesn’t allow us to use arithmetic functions and subtract a whole year because it doesn’t support it. Similarly, if we were to add 1 or 2 while the current date lies in the last days of December, it would give out the same error.
# if you add 1 in date, it will throw an error because it doesn't support it
date = datetime.datetime(2022, 12, 1)
date_ = date.replace(month=date_1.month + 1)
Output:
date = date.replace(month=date.month+1)
ValueError: month must be in 1..12
So, coming back to the question, how can we calculate the date six months from the current date or any other date? The answer lies in using relativedelta
.
Use relativedelta()
to Calculate Date Using Python
Before we go ahead and use relativedelta
in our Python code, we need to install dateutil
to import the relativedelta
from it. Run the following in your command prompt to install the dateutil
.
pip install python-dateutil
Once installed, you need to import the relativedelta
from it.
from dateutil import relativedelta
After that, we need to use both the datetime
and relativedelta
to solve the current problem. Take a look at the following code.
date = datetime.datetime(2022, 1, 1)
# created the instance of the datetime and set the current date as 2022,1,1
Now, we’ll create the instance of the relativedelta
and set the month’s value as 1
.
r_date = relativedelta.relativedelta(months=1)
Now, all we need to do is to subtract the instance of relativedelta
from the instance of the datetime
. It will give us our desired answer.
# if you subtract the relativedelta variable with the date instance, it will work correctly and change the year too.
new_date = date - r_date
print(new_date)
Output:
2021-12-01 00:00:00
Similarly, if we were to add 1 to the date, it would give us the desired output.
Now we can use this technique to calculate any date from the current date. That answers how we can calculate the date of six months from the current date.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn