How to Compare Two Dates in Python
-
Use the
date()
Method to Compare Two Dates in Python -
Use the
datetime()
Method to Compare Two Dates in Python -
Use the
timedelta()
Method to Compare Two Dates in Python -
Use the
time
Module to Compare Two Dates in Python - Conclusion
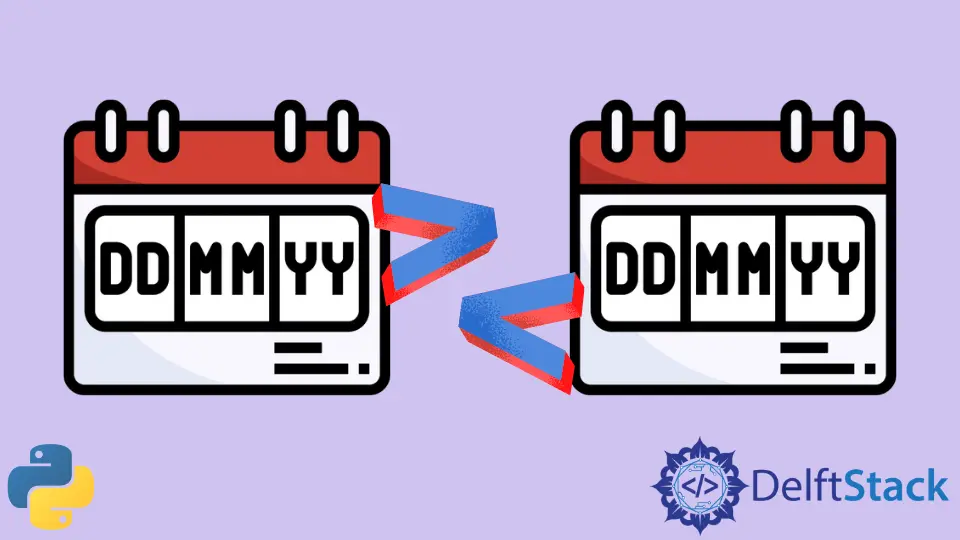
One of the fundamental aspects of working with dates and times in Python is the ability to compare them.
Comparing dates in Python is a crucial skill for many applications. Consider a scenario where we are developing a hotel reservation system.
To determine room availability, we must compare the user’s desired check-in and check-out dates with the existing bookings. By comparing these dates, we can easily identify conflicting reservations, calculate the duration of the stay, and provide accurate availability information to the user.
In Python, dates are primarily represented using the datetime
module, which provides classes and functions to work with dates and times. The datetime
module allows us to create date objects that store information such as the year, month, day, hour, minute, and second.
This tutorial will compare two dates in Python, enabling us to analyze and manipulate temporal data effectively.
Use the date()
Method to Compare Two Dates in Python
The date()
method in Python’s datetime
module is a powerful tool for comparing dates. This method allows us to create date objects representing specific dates without considering the time component.
Syntax:
date(year, month, day)
Parameters:
year
: The year value as an integer.month
: The month value as an integer (1-12).day
: The day value as an integer (1-31).
Python also provides powerful comparison operators to compare dates and determine their relationships. By using these operators, such as greater than (>
), less than (<
), equal to (==
), and not equal to (!=
), we can easily compare two dates and perform various time-based operations.
The following code uses the date()
method with comparison operators to compare two dates in Python.
from datetime import date
previous_date = date(2023, 6, 9)
current_date = date(2023, 6, 29)
print(current_date > previous_date)
Output:
First, we import the date
class from the datetime
module. This class allows us to create date objects that represent specific dates.
Next, we create two date objects: previous_date
and current_date
. In this case, previous_date
represents June 9, 2023, and current_date
represents June 29, 2023.
We use the comparison operator (>
) to compare these dates to check if current_date
is greater than previous_date
. Based on the comparison result, the comparison operator returns a Boolean value (True
or False
).
Finally, we print the comparison result using the print
statement. In this case, the output will be True
since June 29, 2023, is greater than June 9, 2023.
Use the datetime()
Method to Compare Two Dates in Python
In addition to the date()
method, Python’s datetime
module provides the datetime()
method, which offers more precise control over date and time comparisons. This method allows us to create datetime objects that include date and time components.
Using the comparison operators with the datetime()
method, we can perform detailed date and time comparisons in Python.
Syntax:
datetime(year, month, day, hour=0, minute=0, second=0, microsecond=0)
Parameters:
year
: The year value as an integer.month
: The month value as an integer (1-12).day
: The day value as an integer (1-31).hour
: The hour value as an integer (0-23) [Optional].minute
: The minute value as an integer (0-59) [Optional].second
: The second value as an integer (0-59) [Optional].microsecond
: The microsecond value as an integer (0-999999) [Optional].
Compared to the date()
method discussed earlier, the datetime()
method provides additional parameters for specifying the time components. This allows for more precise comparisons that consider both date and time values.
By utilizing the datetime()
method combined with comparison operators, we can accurately compare two dates in Python while considering their date and time aspects.
The following code uses the datetime()
method with comparison operators to compare two dates in Python.
from datetime import datetime
first_date = datetime(2020, 12, 16)
second_date = datetime(2015, 12, 16)
result = first_date < second_date
print(result)
Output:
First, we import the datetime
class from the datetime
module. This class allows us to create datetime objects representing specific dates and times.
Next, we create two datetime objects: first_date
and second_date
. In this case, first_date
represents December 16, 2020, and second_date
represents December 16, 2015.
To compare these datetime objects, we use the comparison operator (<
) to check if first_date
is less than second_date
. Based on the comparison result, the comparison operator returns a Boolean value (True
or False
).
Finally, we assign the result of the comparison to the variable result
and print it using the print
statement. In this case, the output will be False
since December 16, 2020, is not less than December 16, 2015.
Use the timedelta()
Method to Compare Two Dates in Python
The timedelta()
method in Python’s datetime
module provides a convenient way to perform arithmetic operations on dates and times. Using comparison operators with the timedelta()
method, we can compare two dates and calculate the difference between them in terms of days, seconds, or microseconds.
Syntax:
timedelta(
days=0, seconds=0, microseconds=0, milliseconds=0, minutes=0, hours=0, weeks=0
)
Parameters:
days
: The number of days as an integer [Optional].seconds
: The number of seconds as an integer [Optional].microseconds
: The number of microseconds as an integer [Optional].milliseconds
: The number of milliseconds as an integer [Optional].minutes
: The number of minutes as an integer [Optional].hours
: The number of hours as an integer [Optional].weeks
: The number of weeks as an integer [Optional].
The following code uses the timedelta()
method with comparison operators to compare two dates in Python.
from datetime import datetime, timedelta
first_date = datetime(2023, 6, 29)
second_date = datetime(2023, 6, 9)
difference = first_date - second_date
if difference > timedelta(days=7):
print("The difference between the dates is greater than 7 days.")
else:
print("The difference between the dates is less than or equal to 7 days.")
Output:
In this code, we create two datetime objects, first_date
and second_date
, representing June 29, 2023, and June 9, 2023, respectively. We then calculate the difference between the dates using the subtraction operator -
and store it in the difference
variable.
By comparing the difference
with a timedelta
object representing 7 days (timedelta(days=7)
), we can determine if the difference between the dates is greater than or equal to 7 days. Based on the comparison result, the corresponding message will be printed.
Use the time
Module to Compare Two Dates in Python
In addition to the datetime
module, Python’s time
module offers functionalities for comparing dates and times. One such function is time.strptime()
, which converts a date string into a struct_time
object that can be compared.
Syntax:
time.strptime(date_string, format)
Parameters:
date_string
: The input date string to be parsed.format
: The format string specifying the expected date format.
The time.strptime()
method parses the given date_string
using the provided format
and returns a struct_time
object representing the date and time components. The struct_time
object can then be compared using comparison operators.
Compared to the previously discussed methods, time.strptime()
is useful when working with date strings in specific formats. It provides more flexibility in handling different date formats, allowing for accurate comparison and manipulation of dates in Python.
The following code uses the time.strptime()
method to compare two dates in Python.
import time
first_date = "30/11/2020"
second_date = "12/10/2019"
formatted_date1 = time.strptime(first_date, "%d/%m/%Y")
formatted_date2 = time.strptime(second_date, "%d/%m/%Y")
print(formatted_date1 > formatted_date2)
Output:
First, we import the time
module, which provides various time-related functionalities.
Next, we have two date strings: first_date
and second_date
. These date strings are in the day/month/year
format.
To compare these dates, we use the time.strptime()
function, which parses the date strings according to the specified format %d/%m/%Y
and returns a struct_time
object for each date. We store the formatted dates in the formatted_date1
and formatted_date2
variables.
Finally, we compare the formatted dates using the comparison operator (>
) and print the result.
Conclusion
In this tutorial, we explored various methods for comparing dates in Python: date()
, datetime()
, timedelta()
, and time.strptime()
. Each method has its strengths and is suitable for different scenarios.
The date()
method from the datetime
module allows us to create and compare date objects, disregarding the time component. It is useful for date-specific comparisons and provides a straightforward approach.
On the other hand, the datetime()
method extends the functionality by including date and time components. This method allows for more precise comparisons that consider both aspects.
The timedelta()
method provides a way to calculate the difference between two dates and times. It allows us to perform arithmetic operations on dates, such as adding or subtracting durations. This method is particularly useful for measuring intervals and time-based calculations.
Lastly, the time.strptime()
function from the time
module is beneficial when working with date strings in specific formats. It allows us to parse date strings and convert them into struct_time
objects, enabling accurate comparison and manipulation of dates.
With this knowledge, we can confidently tackle date comparison challenges and handle temporal data in your Python programming projects.
Syed Moiz is an experienced and versatile technical content creator. He is a computer scientist by profession. Having a sound grip on technical areas of programming languages, he is actively contributing to solving programming problems and training fledglings.
LinkedIn