Python Tutorial - Variables and Data Types
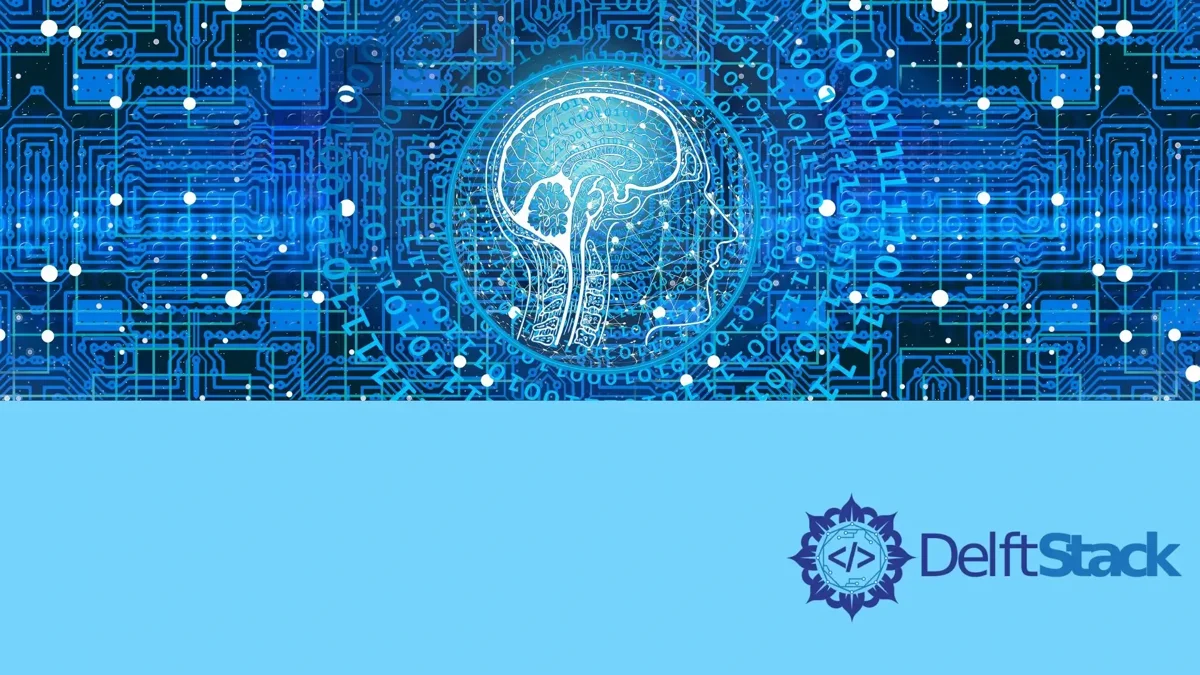
In this section, you will learn variables and data types in Python programming.
Python Variable
A variable is a memory location that has a name and a value (some data).
Variables have unique names so that memory locations can be differentiated. They have the same naming rules to follow as that of identifiers. Check identifiers naming rules.
In Python, it is not necessary to declare a variable (specifying the data type) before using that variable. If you want to create a variable, just write a valid name for the variable and assign it some value using assignment operator. The data type of variable will be defined internally according to the type of value that you assign to it.
Variable Assignment
An assignment operator =
is used to assign a value to a variable. You can assign any type of value to a variable. The data type of variable will be defined internally.
The following code demonstrates how an assignment operator can be used to assign values of different data types:
x = 3
y = 4.124
z = "Python"
Here you have int
, float
and string
variables.
Multiple Assignments
You can also assign values to multiple variables in a single Python statement. This is called multiple assignments. Consider the code below:
x, y, z = 2, 4.124, "Python"
Here 2 is assigned to x
, 4.124 is assigned to y
and string “Python” is assigned to z
.
If you want to assign the same value to different variables, you can write:
x = y = z = "Blue"
Now all the three variables are equal.
Python Data Type
Whenever you assign a value to a variable, the data type of the variable is also specified. Python is an object-oriented programming, and everything in Python is an object because Python has no primitive
or unboxed
types.
In Python, the data types of variables are actually class of which variables are the instances (objects).
The following are some of data types in Python:
Numbers
Numbers such as integers, floating point, and complex numbers are Python numbers. In Python, integers are represented by int
, floating points by float
and complex numbers by complex
class.
The built-in type()
function can be used to check the data type of a variable or to which class a variable belongs.
The isinstance()
function is used to check whether the object is an instance of the specific clas or a subclass thereof. Its first argument is the object itself and the second one is the data type to be compared with.
x = 3
print("type of", x, "is", type(x))
x = 3.23
print("type of", x, "is", type(x))
x = 3 + 3j
print("is", x, "a complex number:", isinstance(x, complex))
type of 3 is <class 'int'>
type of 3.23 is <class 'float'>
is (3+3j) a complex number: True
List
A list is an ordered sequence of elements of (different) data types. A list can be created in Python by using square brackets []
and inside square brackets you have list items that are separated by commas.
x = [2, 4.5, "blue"]
To access the member of the list you can use index access operator which in Python is known as slicing operator []
. The indexing of the elements of list starts from 0. Consider the example below:
x = [2, 4, 6, 8, 10]
print("x[0] =", x[0]) # displays the first element
print(
"x[0:2] =", x[0:2]
) # displays first two elements of list that is at location 0 and 1
print("x[2:] =", x[2:]) # displays elements from location 2 (third element)
x[0] = 2
x[0:2] = [2, 4]
x[2:] = [6, 8, 10]
The items of Python list can be changed (updated, deleted, added). In another words, Python list is mutable.
Tuple
Tuple is also an ordered list of items the same as list. The only difference between a tuple and a list is that the tuple element is immutable.
You can define a Python tuple using parenthesis and elements are separated with commas:
x = (3, "pink", 3 + 8j)
print("x[0] =", x[0])
print("x[0:2] =", x[0:2])
x[0] = 4 # Error because tuples are immutable
x[0] = 3
x[0:2] = (3, 'pink')
TypeError: 'tuple' object does not support item assignment
String
A string is a sequence of characters. A string can be created using single or double quotation marks. A multiline string can also be created by using triple quotation marks.
x = "String of characters"
x = """Multiline
string"""
To access characters of string slicing operator is used.
x = "Python Programming"
print("x[0] =", x[0])
print("x[4:15] =", x[4:15])
x[4] = "l" # Error because strings are immutable
x[0] = P
x[4:15] = on Programm
TypeError: 'str' object does not support item assignment
Set
Set contains unique items and is unordered list of elements. A set in Python can be created using curly braces {}
and the items are separated by commas.
x = {3, 5, 7, 2, 4, 5}
print(x) # prints the set variable
print(type(x)) # checking type of x
{2, 3, 4, 5, 7}
<class 'set'>
Dictionary
Dictionary is a Python native data type whose elements are key-value pairs. A dictionary contains unordered elements. A piece of data or value of the dictionary can be retrieved if you know the key.
A dictionary can be created using curly braces {}
with each element to be a pair containing key and value.
Keys and values in Python dictionary can be of any type.
Consider the example below:
x = {1: "blue", "key": "pink"}
print(type(x))
print("x[1] = ", x[1]) # using key to retrive value
print("x['key'] =", x["key"]) # using key to retrive value
<class 'dict'>
x[1] = blue
x['key'] = pink
Type Conversion
Python variables can be converted into other types, for example, you can convert int
data type into float
data type. For type conversion you can use conversion function like int()
, float()
, str()
, etc.
x = 8
print("Value of x = ", int(x))
print("Value of x = ", float(x))
Value of x = 8
Value of x = 8.0
If you want to convert float
value to int
value then the decimal part will be truncated.
y = 3.4
print("Converting float to int:", int(y))
Converting float to int: 3
If you want to convert string
data type to another type, you must provide compatible values, otherwise you will get a ValueError
:
print("Converting string to float:", float("33.9"))
print("Converting string to float:", float("text"))
Converting string to float: 33.9
Traceback (most recent call last):
File "<pyshell#12>", line 1, in <module>
float('a')
ValueError: could not convert string to float: text
You can convert one sequence into another, for example, set
to a tuple
or a string
to list
and so on by using functions like list()
, set()
, tuple()
, etc.
print(set([4, 5, 6]))
print(tuple({8, 9, 10}))
print(list("Python"))
{4, 5, 6}
(8, 9, 10)
["P", "y", "t", "h", "o", "n"]
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook