Python Regex Escape
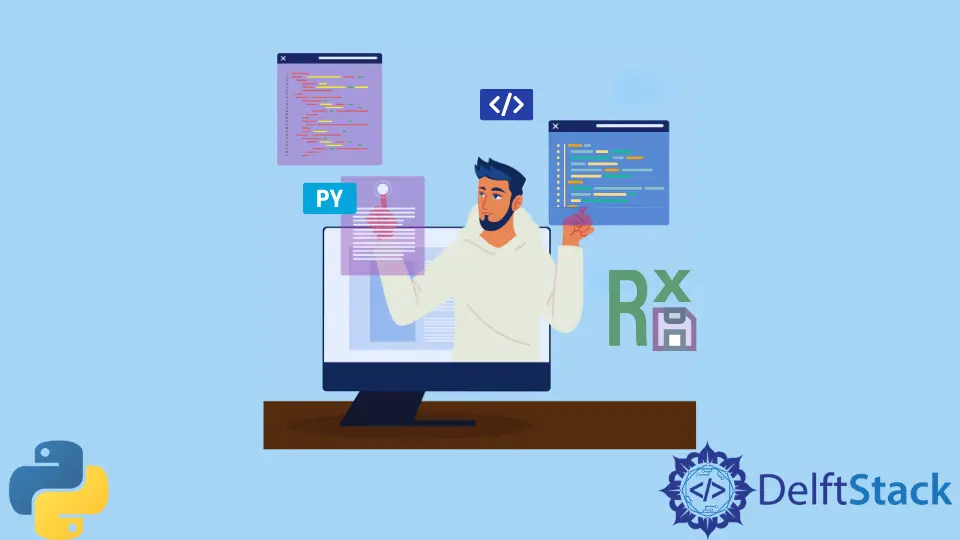
This article is about Python regex escape. Additionally, we will cover Python regex escape and how to use it with proper example code, as well as multiple uses for Python Regex.
Furthermore, Python supports search and replace operations on strings using regex (or regular expressions).
Python Regex Escape
RegEx
is a tremendous technique for matching text according to a predefined pattern. Comparing a text with a particular pattern can detect whether it is present or absent.
It can also break a pattern into subpatterns. In Python, regex escape can perform a wide range of tasks.
It can perform search and replace operations, replace patterns in text, and check if a string contains a specific pattern. Using regex, we will also perform search and replace operations today.
Regular expressions in Python can be expressed using the re
module from the standard library. A regular expression and a string consider their primary purpose, which is to perform a search.
A match will either be returned if there is a first match or not.
Python has a default module, re
, that assists with search and replacement. Our code must import the module at the beginning to use it.
import re
Example Code:
import re
match = re.search(
r"Analytics",
"DataScience: Data Science & Analytics\ subfield of AI",
)
print(match)
print(match.group())
print("Begin the Index:", match.start())
print("Let's finish the Index:", match.end())
Output:
<re.Match object; span=(28, 37), match='Analytics'>
Analytics
Begin the Index: 28
Let's finish the Index: 37
Syntax for Python Regex Escape
A raw property is represented by the r
character, (r'property')
here, not by a regex. Unlike a regular string, a plain string will not interpret the \
character as an escape character.
Due to the way regular expression engines use the /
character for escaping, it uses /
characters to escape.
Let’s elaborate on this with Python.
import re
# When an escape() is used in the first case, it returns a string with BackSlash '/' before every non-Alphanumeric character. In the second case, it returns a string with BackSlash '/' before '' only.
print(re.escape("It was really a wonderful experience so far"))
print(re.escape("I am curious about the experience [a-9], she said \t ^Awesome"))
Output:
It\ was\ really\ a\ wonderful\ experience\ so\ far
I\ am\ curios\ about\ the\ experience\ \[a\-9\],\ she\ said\ \ \ \^Awesome
Also, if you want to check if a word exists in a string with a regular expression in Python, commas can be used to separate the string.
As long as there are no escape characters in the string, the r
makes the string a raw string, which does not process escape characters.
Additionally, re.match
matches from the start of the string. This means it searches for exact matches between the string and the pattern.
Use re.search
if you need to match anything in the string anywhere. The following is an example.
import re
line = "This,is,a,practice,string"
re.match("practice", line)
re.search("practice", line)
Output:
<re.Match object; span=(10, 18), match='practice'>
Further, we’ll learn about the multiple uses of Python regex.
Multiple Use of Python Regex
Search and Replace With Regex
In the regex method, a string is searched, and then some other value is replaced. Python’s re.sub()
function in the re
module is used to accomplish this.
Syntax:
re.sub(pattern, replacement, string, count=0, flags=0)
We can search and find a string using a regular expression using Python. In this case, the string is the variable containing the string that you want to operate on.
We can specify how many times you want the pattern to be replaced if it occurs multiple times in the string. 0 is the default value; in any case, it is optional.
There is no need to specify regex flags.
Example Code:
import re
str = "abid304@outlook.com"
print(re.sub("[a-z]*@", "abc@", str))
Output:
abid304abc@outlook.com
Multipattern Replacement With Regex
Multiple patterns can be replaced at once using regex. The following syntax can be used to accomplish this easily.
Syntax:
re.sub(pattern_11 | pattern_22, replacement, string, count=0, flags=0)
Example code:
import re
str = "MachineLearning is the Subfield of AI"
print(re.sub(r"(\s)|(-)", ",", str))
Output:
MachineLearning,is,the,Subfield,of,AI
Replace Multiple Patterns With Multiple Replacements Using Regex
Regex can also be used to replace multiple patterns with different replacements. As shown in the following example, a minor modification is necessary.
Example code:
import re
def convrt_case(mtch_obj):
if mtch_obj.group(1) is not None:
return mtch_obj.group(1).lower()
if mtch_obj.group(2) is not None:
return mtch_obj.group(2).upper()
str = "mACHINE lEARNING IS THE sUBFIELD OF ai"
print(re.sub(r"([A-Z]+)|([a-z]+)", convrt_case, str))
Output:
Machine Learning is the Subfield of AI
As shown in this example, the string contains both uppercase and lowercase letters that need to be replaced. The uppercase needs to be replaced with the lowercase and vice versa.
We will create two groups and then add a function to replace them.
In Python, the regex escape sub()
method is used to replace a string. The replaced string is returned by a Python built-in method in the re
module.
Make sure you import the re
module. In this method, the pattern in the string is searched and replaced with a new expression.
We hope this Python article has helped you understand how to use regex escape
in Python more effectively.
My name is Abid Ullah, and I am a software engineer. I love writing articles on programming, and my favorite topics are Python, PHP, JavaScript, and Linux. I tend to provide solutions to people in programming problems through my articles. I believe that I can bring a lot to you with my skills, experience, and qualification in technical writing.
LinkedIn