Case Insensitive Regex in Python
- Case Insensitive Regex in Python
-
Match a String Using the Case Insensitive
re.IGNORECASE
andre.I
Flag in Python -
Match a String Using the Case Insensitive Marker
(?i)
in Python - Conclusion
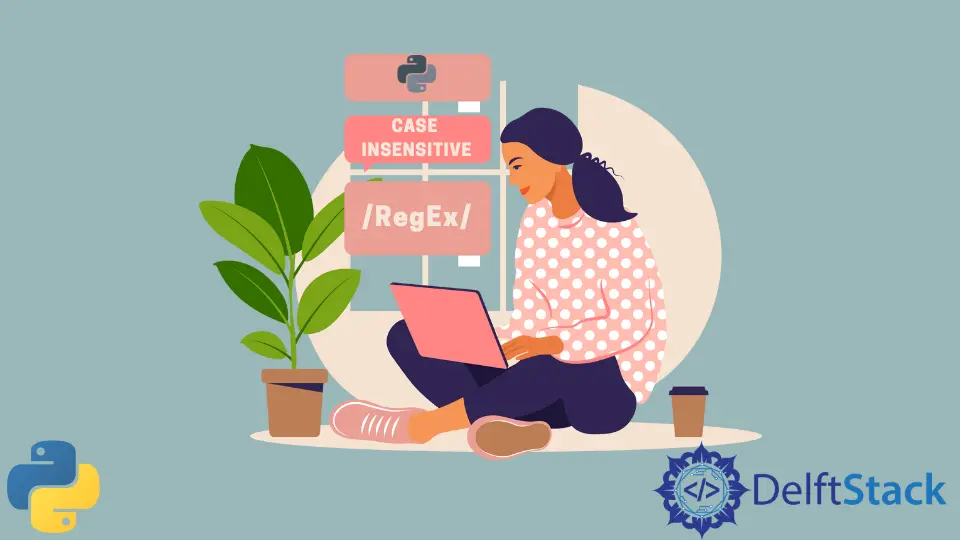
Regular expressions match a particular string within a text in Python. They form a search pattern and check if this search pattern is present in the text or not.
In this article, we will be studying the case-insensitive regex in Python. The different ways of performing the case insensitive searches in a text are explained further.
Case Insensitive Regex in Python
Search patterns are made up of a sequence of characters and can be specified using regex rules. However, to work with regular Python expressions, you first need to import the re
module.
Case insensitive means that the text should be considered equal in lowercase and uppercase. We need to apply case-insensitive searches in our daily lives very often.
One such example is whenever we search for some commodity, say, a Bag
. The information about the Bags will be displayed on the screen.
However, if we search bag
in lowercase letters or use mixed cases such as bAG
, it should also display the same results. Therefore, we need to treat different case letters to be the same to search the results in specific scenarios easily.
This is why we use regular expressions which check the case-insensitive patterns within a text. So, let us discuss how to extract a search pattern from a text using regular expressions.
Match a String Using the Case Insensitive re.IGNORECASE
and re.I
Flag in Python
When you want to match a string in Python using a case-insensitive approach, you can leverage the re.IGNORECASE
flag or the re.I
method within the re.findall
function. This ensures that the pattern matching is not sensitive to the case of the characters in the input string.
Let’s delve into a concise example that demonstrates the power of the re.IGNORECASE
flag using the re.findall
method.
Example:
import re
# Define the pattern to search for
pattern_to_search = "python"
# Define the text to search in
text_to_search_in = "Python is a powerful programming language. python is versatile."
# Use re.findall with re.IGNORECASE flag directly
matches = re.findall(pattern_to_search, text_to_search_in, re.IGNORECASE)
# Output the matches
print(matches)
Now, let’s break down this simple yet effective code snippet.
We begin by importing the re
module, which provides the necessary tools for working with regular expressions in Python. Then, we set the regex pattern we want to search for (pattern_to_search
) and the text in which we want to perform the search (text_to_search_in
).
Next, we use re.findall
with re.IGNORECASE
. By directly calling re.findall
with the regex pattern, input text, and the re.IGNORECASE
flag, we instruct Python to perform a case-insensitive search and return all matches.
Finally, we print the result directly. The re.findall
method returns a list of matches, and in this case, it outputs the matches without the need for additional variables or if
statements.
Output:
['Python', 'python']
In this output, you can see that the case-insensitive regex search successfully identified both instances of the pattern python
in the input text, regardless of their capitalization.
The re.IGNORECASE
flag, which is used above, can also be written as re.I
. This re.I
flag is also used to search a case-insensitive pattern within a text.
Example:
import re
# Define the pattern to search for
pattern_to_search = "python"
# Define the text to search in
text_to_search_in = "Python is a powerful programming language. python is versatile."
# Use re.findall with re.I directly
matches = re.findall(pattern_to_search, text_to_search_in, re.I)
# Output the matches
print(matches)
Now, let’s break down this straightforward code snippet step by step.
We begin by importing the re
module, a Python module that provides support for regular expressions. Then, we set the regex pattern we want to search for (pattern_to_search
) and the text in which we want to perform the search (text_to_search_in
).
Next, we use re.findall
with re.I
. By directly calling re.findall
with the regex pattern, input text, and the re.I
method, we instruct Python to perform a case-insensitive search and return all matches.
Finally, we print the result directly. The re.findall
method returns a list of matches, and in this case, it outputs the matches without the need for additional variables or if
statements.
Output:
['Python', 'python']
In this output, we can see that the case-insensitive regex search successfully identified both instances of the pattern python
in the input text, regardless of their capitalization.
Match a String Using the Case Insensitive Marker (?i)
in Python
If we want to match a string in Python using a case-insensitive approach, we can use the (?i)
marker within the regular expression pattern. This marker instructs the regex engine to perform the matching without considering the case of the characters in the input string.
Let’s dive into a concise example that demonstrates the simplicity and effectiveness of the (?i)
method using the re.findall
approach.
Example:
import re
# Define the pattern to search for
pattern_to_search = "(?i)python"
# Define the text to search in
text_to_search_in = "Python is a powerful programming language. python is versatile."
# Use re.findall with the (?i) method directly
matches = re.findall(pattern_to_search, text_to_search_in)
# Output the matches
print(matches)
Now, let’s dissect this concise code snippet step by step.
We start by importing the re
module, a Python module that provides support for regular expressions. Then, we set the regex pattern we want to search for (pattern_to_search
) and the text in which we want to perform the search (text_to_search_in
).
We then use re.findall
with (?i)
. By directly calling re.findall
with the regex pattern, input text, and the (?i)
method, we instruct Python to perform a case-insensitive search and return all matches.
Finally, we print the result directly. The re.findall
method returns a list of matches, and in this case, it outputs the matches without the need for additional variables or if
statements.
['Python', 'python']
In this output, we can see that the case-insensitive regex search successfully identified both instances of the pattern python
in the input text, regardless of their capitalization.
Conclusion
The re.IGNORECASE
flag, the re.I
method, and the (?i)
marker offer versatile solutions for achieving case-insensitive regex matching in Python. By seamlessly integrating these techniques, developers can efficiently search for patterns without being sensitive to character cases.
Whether you choose the explicitness of re.IGNORECASE
, the concise re.I
method, or the marker-based (?i)
approach, all methods deliver powerful outcomes, enhancing the versatility of your regex-based solutions.