How to Convert Java String Into Byte
-
getBytes()
Method to Convert Java String Into Byte -
getBytes()
Method With Specific Encoding to Convert Strings in Java
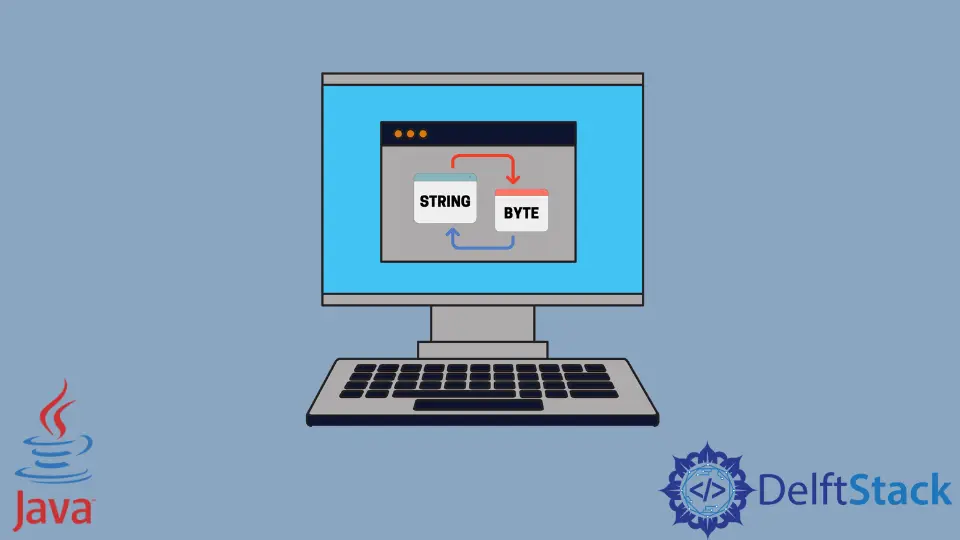
In this tutorial, we will learn how to convert Java string into byte. This conversion can be done using different classes depending upon the requirement. In some cases, the user needs to perform encoding as well while converting into bytes; as per requirement.
getBytes()
Method to Convert Java String Into Byte
The first method we start with is the getBytes()
method of Java. This method will take the string and convert it into Bytes inside an array.
Example Codes:
import java.text.*;
import java.util.Arrays;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
String string = "Simple Testing";
byte[] bytes = string.getBytes();
System.out.println("String: " + string);
System.out.println("Bytes: " + Arrays.toString(bytes));
}
}
Output:
String: Simple Testing
Bytes: [83, 105, 109, 112, 108, 101, 32, 84, 101, 115, 116, 105, 110, 103]
getBytes()
Method With Specific Encoding to Convert Strings in Java
In order to convert string array to byte
in UTF-8 encoding for compatible platforms, we could use getBytes(StandardCharsets.UTF-8)
method. It works similarly to the default getBytes()
method and returns the output encoded in the given encoding format.
Example Codes:
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.text.*;
import java.util.Arrays;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
String string = "Simple Testing with UTF-8 Encoding";
byte[] bytes = string.getBytes(StandardCharsets.UTF_8);
System.out.println("String: " + string);
System.out.println("Bytes: " + Arrays.toString(bytes));
}
}
Output:
String: Simple Testing with UTF-8 Encoding
Bytes: [83, 105, 109, 112, 108, 101, 32, 84, 101, 115, 116, 105, 110, 103, 32, 119, 105, 116, 104, 32, 85, 84, 70, 45, 56, 32, 69, 110, 99, 111, 100, 105, 110, 103]
Another encoding format that can be used with getBytes()
method is defaultCharset
for relevant compatible platforms.
Example Codes:
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.text.*;
import java.util.Arrays;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
String string = "Simple Testing with default Charset Encoding";
byte[] bytes = string.getBytes(Charset.defaultCharset());
System.out.println("String: " + string);
System.out.println("Bytes: " + Arrays.toString(bytes));
}
}
Output:
String: Simple Testing with default Charset Encoding
Bytes: [83, 105, 109, 112, 108, 101, 32, 84, 101, 115, 116, 105, 110, 103, 32, 119, 105, 116, 104, 32, 100, 101, 102, 97, 117, 108, 116, 32, 67, 104, 97, 114, 115, 101, 116, 32, 69, 110, 99, 111, 100, 105, 110, 103]
Another encoding format that can be used with getBytes()
method is UTF-16
for the latest compatible platforms.
Example Codes:
import java.nio.charset.Charset;
import java.nio.charset.StandardCharsets;
import java.text.*;
import java.util.Arrays;
import java.util.Date;
public class SimpleTesting {
public static void main(String[] args) {
String string = "Simple Testing with UTF-16 Encoding";
byte[] bytes = string.getBytes(StandardCharsets.UTF_16BE);
System.out.println("String: " + string);
System.out.println("Bytes: " + Arrays.toString(bytes));
}
}
Output:
String: Simple Testing with UTF-16 Encoding
Bytes: [0, 83, 0, 105, 0, 109, 0, 112, 0, 108, 0, 101, 0, 32, 0, 84, 0, 101, 0, 115, 0, 116, 0, 105, 0, 110, 0, 103, 0, 32, 0, 119, 0, 105, 0, 116, 0, 104, 0, 32, 0, 85, 0, 84, 0, 70, 0, 45, 0, 49, 0, 54, 0, 32, 0, 69, 0, 110, 0, 99, 0, 111, 0, 100, 0, 105, 0, 110, 0, 103]
Related Article - Java String
- How to Perform String to String Array Conversion in Java
- How to Remove Substring From String in Java
- How to Convert Byte Array in Hex String in Java
- How to Generate Random String in Java
- The Swap Method in Java