The Swap Method in Java
-
Swap Two Elements in a Java List With the
swap
Method - Swap Two Characters in a Java String
- Swap Two Objects in Java
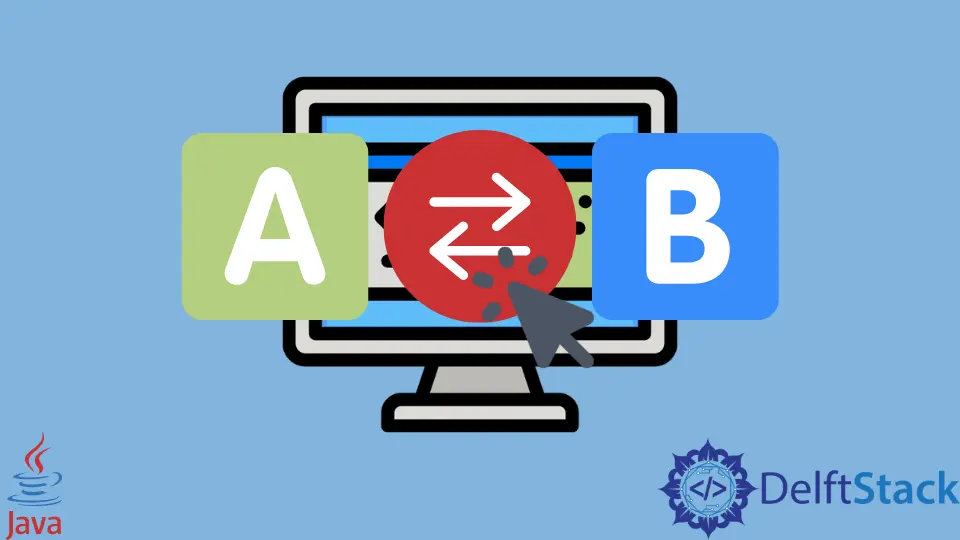
The swap()
method is used to exchange the position of two elements, characters, or objects in Java. This method can be applied to a list, a string, or an object.
In this article, we will discuss the use of the swap()
method in:
- Swapping two elements in a list
- Swapping two characters in a string
- Swapping two objects
Swap Two Elements in a Java List With the swap
Method
This is a method used to exchange two specific elements in defined positions without impacting other elements in a list. If one of the specified indexes is higher than the list’s size, then the method returns an out of bound
exception. The swap()
will give an output of the list with the elements in the indexes swapped.
Syntax:
public static void swap(List mylist, int m, int n)
This method takes three parameters as its arguments - the list that the swap()
method will be applied on and the two indexes which are to be exchanged.
Code example:
import java.util.ArrayList;
import java.util.Collections;
public class PerformSwap {
public static void main(String[] args) {
ArrayList<String> lettersList = new ArrayList<String>();
lettersList.add("a");
lettersList.add("b");
lettersList.add("c");
lettersList.add("d");
lettersList.add("e");
lettersList.add("f");
lettersList.add("g");
System.out.println("Original List: " + lettersList);
Collections.swap(lettersList, 0, 6);
System.out.println("\nSwapped List: " + lettersList);
}
}
Output:
Original List: [a, b, c, d, e, f, g]
Swapped List: [g, b, c, d, e, f, a]
In the example above, we have exchanged the letter g
on index 6 with the letter a
on index 0
. The swap
method has only exchanged these two letters without interfering with any other list elements.
Swap Two Characters in a Java String
One of the string value’s main properties is that it is immutable, meaning it cannot be changed. To perform the swap operation, we first have to copy the String
object to a StringBuilder
or a character array. These two data types allow us to perform swap operation on the copied object. Below, we’ll perform the swap operation using char array and StringBuilder
to create a new string with swapped characters.
Perform String Swap Using Char Array in Java
The swap method has three parameters - the String we are going to perform swap on and the two indexes of the characters we exchange. To perform a character swap, we first create a temporary character storage object - tempo
. This temporary object stores the first character as we replace it with the second character and then passes this character to the second character to complete the exchange process.
There are three steps involved:
-
Convert the String to a char array object
-
Get the length of the object
-
Swap the indexes of the char array
Code example:
public class SwapString {
static char[] swap(String mystring, int i, int j) {
char ch[] = mystring.toCharArray();
char tempo = ch[i];
ch[i] = ch[j];
ch[j] = tempo;
return ch;
}
public static void main(String args[]) {
String theS = "Favourite";
System.out.println(swap(theS, 5, 2));
System.out.println(swap(theS, 0, theS.length() - 1));
System.out.println(theS);
}
}
Output:
Farouvite
eavouritF
Favourite
Perform String Swap Using StringBuilder in Java
Code example:
public class SwapString {
static String swap(String mystring, int i, int j) {
StringBuilder mysb = new StringBuilder(mystring);
mysb.setCharAt(i, mystring.charAt(j));
mysb.setCharAt(j, mystring.charAt(i));
return mysb.toString();
}
public static void main(String args[]) {
String theS = "Favorite";
System.out.println(swap(theS, 5, 2));
System.out.println(swap(theS, 0, theS.length() - 1));
// Original String
System.out.println(theS);
}
}
Output:
Faiorvte
eavoritF
Favorite
Swap Two Objects in Java
The swap
method can also be used to swap the two objects’ attributes. Objects swap can be performed on objects with one attribute and also on objects with more than one attribute.
Swap Object With One Attribute
Suppose we have a class called House
with some attributes such as the number of bedrooms and the number of bathrooms. Let’s create two objects of House
- house1
and house2
. House
has only one attribute - value
. Our goal is to swap the data in house1
and house2
.
Code example:
public class SwapObjects {
public static void swap(House house1, House house2) {
House temp = house1;
house1 = house2;
house2 = temp;
}
public static void main(String[] args) {
House house1 = new House();
House house2 = new House();
house1.value = 5;
house2.value = 2;
// swap using objects
swap(house1, house2);
System.out.println(house1.value + ", " + house2.value);
}
}
class House {
public int value;
}
Output:
5, 2
Swap Object With More Than One Attribute in Java
We use a Wrapper
class to swap the attribute of two objects that have multiple attributes. If we perform the swap without the wrapper class, the function swap will only create a copy of the object references.
public class SwapObjects {
public static void main(String[] args) {
House house1 = new House(5, 3);
House house2 = new House(2, 1);
Wrapper whs1 = new Wrapper(house1);
Wrapper whs2 = new Wrapper(house2);
// swap using wrapper of objects
swap(whs1, whs2);
whs1.house.print();
whs2.house.print();
}
public static void swap(Wrapper whs1, Wrapper whs2) {
House temp = whs1.house;
whs1.house = whs2.house;
whs2.house = temp;
}
}
class House {
int bedroom, bathroom;
// Constructor
House(int bedroom, int bathroom) {
this.bedroom = bedroom;
this.bathroom = bathroom;
}
// Utility method to print object details
void print() {
System.out.println("bedrooms = " + bedroom + ", bathrooms = " + bathroom);
}
}
class Wrapper {
House house;
Wrapper(House house) {
this.house = house;
}
}
Output:
bedrooms = 2, bathrooms = 1
bedrooms = 5, bathrooms = 3
The wrapper class swaps objects even if the member’s class doesn’t give access to the user class. While applying the swap()
method to an object, you can select the approach to use based on the number of attributes in an object.