How to Generate Random String in Java
- Generate Random String in Java With the Regular Expression
-
Generate Random Alphanumeric String in Java Using the
Math.random()
Method - Generating Using Charset
- Use Apache Commons Lang
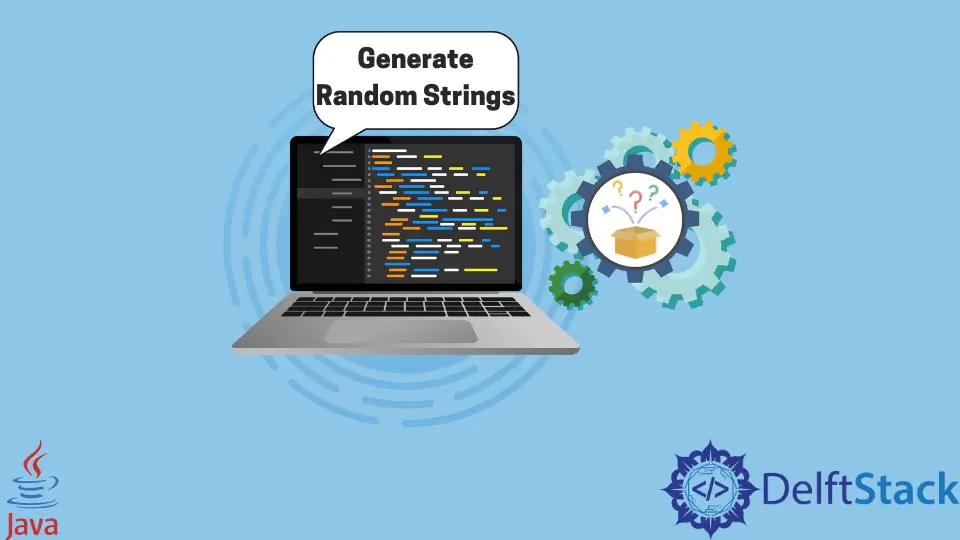
An alphanumeric string contains numeric and alphabetical characters. In Java, there are numerous cases where alphanumeric strings are utilized. For instance, in generating a password after the user registers on an application; in generating a primary key entry to identify an element in a database; in generating session ids, among others.
This article will discuss four methods to generate a random alphanumeric string in Java.
- the regular expression
- the
Math.random()
method - the
Charset
Apache Commons Lang
Generate Random String in Java With the Regular Expression
This method is easy and gives an alphanumerical string that contains uppercase in lowercase characters and digits.
The first thing is to take chars that are between 0 and 256.
The second step is to filter the chars to be left with the elements we want to include in our random String. Keep in mind that the chars we want to remain with are 0-9, and A-Z, so we can create a filter that removes all the other chars.
The next step involves selecting the random chars and adding them to a StringBuffer
. We then create a String from the StringBuffer using the toString()
method.
Below is a sample code.
import java.nio.charset.*;
import java.util.*;
class GenerateAlphaNumericString {
static String getRandomString(int i) {
// bind the length
bytearray = new byte[256];
byte[] bytearray;
String mystring;
StringBuffer thebuffer;
String theAlphaNumericS;
new Random().nextBytes(bytearray);
mystring = new String(bytearray, Charset.forName("UTF-8"));
thebuffer = new StringBuffer();
// remove all spacial char
theAlphaNumericS = mystring.replaceAll("[^A-Z0-9]", "");
// random selection
for (int m = 0; m < theAlphaNumericS.length(); m++) {
if (Character.isLetter(theAlphaNumericS.charAt(m)) && (i > 0)
|| Character.isDigit(theAlphaNumericS.charAt(m)) && (i > 0)) {
thebuffer.append(theAlphaNumericS.charAt(m));
i--;
}
}
// the resulting string
return thebuffer.toString();
}
public static void main(String[] args) {
// the random string length
int i = 15;
// output
System.out.println("A random string: " + getRandomString(i));
}
}
Output:
A random string: 4H4V7FWP8ZUKEGW
Generate Random Alphanumeric String in Java Using the Math.random()
Method
It involves creating a string that contains all the possible characters manually. We then create a random String of defined length by selecting characters and digits from the string we created previously.
First, we defined the two variables that we will need - the StringBuilder
and String
. The String
will contain all letters of the alphabet as uppercase and digits 0-9. This string should have no symbol or space because the indexes of the characters in the string will be used to select characters randomly.
Secondly, define the iteration that is limited to the length of the random string we want to create.
Third, generate a random index using the Math.random()
method. Get the character from the previously created string that is the index’s position generated randomly and append it to the StringBuilder
. Then we can get the random string from the StringBuilder by using the toString()
method.
Below is a sample code.
class AlphaNumericStringGenerator {
static String getRandomString(int i) {
String theAlphaNumericS;
StringBuilder builder;
theAlphaNumericS = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"
+ "0123456789";
// create the StringBuffer
builder = new StringBuilder(i);
for (int m = 0; m < i; m++) {
// generate numeric
int myindex = (int) (theAlphaNumericS.length() * Math.random());
// add the characters
builder.append(theAlphaNumericS.charAt(myindex));
}
return builder.toString();
}
public static void main(String[] args) {
// the random string length
int i = 15;
// output
System.out.println("A random string: " + getRandomString(i));
}
}
Output:
A random string: BCQJPZLG3OC1MQD
Generating Using Charset
We use the Charset, which is in the java.nio.charset
package. A charset stands for character set and represents a mapping between characters and numbers. The process of creating an alphanumeric string using Charset is explained below.
The first step is to take chars between 0 and 256. The Random()
method is then applied to the array of the characters above. The third step is to check whether each char is alphabetic or numeric by iteration. If it is either of them, we add that character to the end of the string until we will the defined length. The characters appended to the StringBuilder
are converted to the desired string using the toString()
method.
Below is a sample code.
import java.nio.charset.*;
import java.util.*;
class AlphaNumericStringGenerator {
static String getRandomString(int i) {
byte[] bytearray;
String mystring;
StringBuffer thebuffer;
bytearray = new byte[256];
new Random().nextBytes(bytearray);
mystring = new String(bytearray, Charset.forName("UTF-8"));
// Create the StringBuffer
thebuffer = new StringBuffer();
for (int m = 0; m < mystring.length(); m++) {
char n = mystring.charAt(m);
if (((n >= 'A' && n <= 'Z') || (n >= '0' && n <= '9')) && (i > 0)) {
thebuffer.append(n);
i--;
}
}
// resulting string
return thebuffer.toString();
}
public static void main(String[] args) {
// the random string length
int i = 15;
// output
System.out.println("A random string: " + getRandomString(i));
}
}
Output:
A random string: XKT0JAHPRPZ6A3X
Use Apache Commons Lang
This is the easiest method to implement because a third party handles the implementation, in this case, the Apache
package.
The Apache Commons Lang
package provides many helper utilities related to string manipulation in Java. Because it is a third party, it must first be added as a dependency in the project.
We can use the RandomStringUtils class
to generate the random string This class has three methods that can give random strings:
RandomStringUtils.randomNumeric
: create a random string of specified length.RandomStringUtils.randomAlphabetic
: create a random alphabetic string with uppercase and lowercase charactersRandomStringUtils.randomAlphanumeric
: creates a random alphanumeric string with the specified length.
Below is a code sample.
import org.apache.commons.lang3.RandomStringUtils;
public class Index {
public static void main(String[] args) {
System.out.println(RandomStringUtils.randomAlphanumeric(15) toUpperCase(););
}
}
Output:
RM0WMYOKN7AS0KA
The RandomStringUtils.randomAlphanumeric
directly implements the random alphanumeric with the option of having alphabets in lowercase or uppercase or both. This is an efficient way when you don’t want to implement the string generation within your code. The Apache library is powerful and has a host of other features such as basic numerical methods, concurrency, and object reflection.