How to Convert Byte Array in Hex String in Java
-
HEX_ARRAY[]
Method to Convert Byte Array to Hex String in Java -
Hex.encodeHexString()
Method to Convert Byte Array to Hex String in Java -
DatatypeConverter()
Method to Convert Byte Array in Hex String in Java -
append(.format)
Method for Conversion of Byte Array Into Hex String in Java
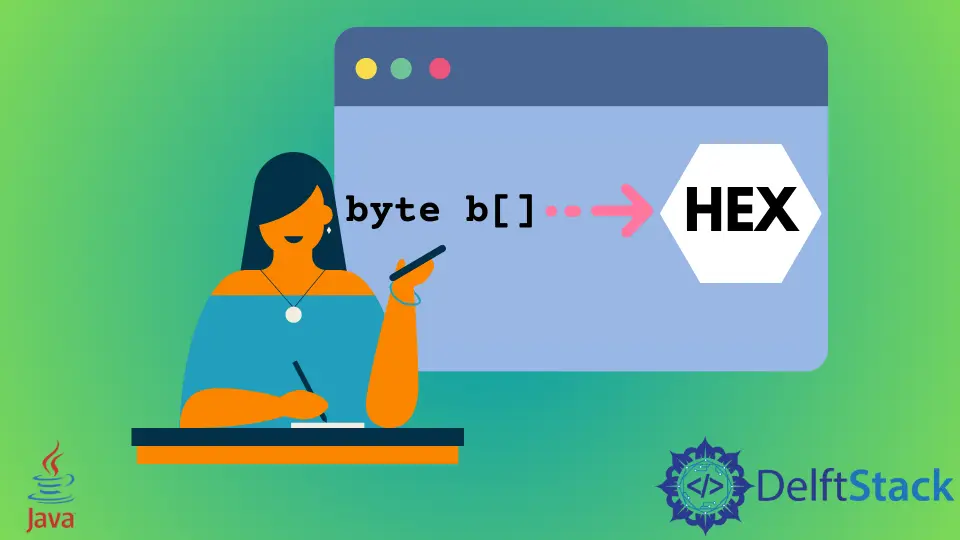
We have introduced how to convert byte array to string in Java in another article.
In this tutorial, we will learn how to convert byte array to hex string in Java.
HEX_ARRAY[]
Method to Convert Byte Array to Hex String in Java
The first method we will be starting with, for this conversion we will use HEX_ARRAY[]
consisting of all possible hex values. This method is faster than any other alternative.
Example Codes:
public class SimpleTesting {
public static void main(String[] args) {
char[] HEX_ARRAY = "0123456789ABCDEF".toCharArray();
byte b[] = new byte[2];
b[0] = 20;
b[1] = 10;
char[] hexChars = new char[b.length * 2];
for (int j = 0; j < b.length; j++) {
int v = b[j] & 0xFF;
hexChars[j * 2] = HEX_ARRAY[v >>> 4];
hexChars[j * 2 + 1] = HEX_ARRAY[v & 0x0F];
}
System.out.println(hexChars);
}
}
Output:
140A
Hex.encodeHexString()
Method to Convert Byte Array to Hex String in Java
The second method to convert byte array to hex string in Java is Hex.encodeHexString()
method. Since its an apache
’s commons library, hence method apache.commons.codec.binary.Hex()
needs to be imported first inside the compiler.
Example Codes:
import java.util.Arrays;
import org.apache.commons.codec.binary.Hex;
public class SimpleTesting {
public static void main(String[] args) {
byte[] byteArray = new byte[] {'S', 'i', 'm', 'p', 'l', 'e', 'T', 'e', 's', 't', 'i', 'n', 'g'};
System.out.println("Byte Array: ");
System.out.println(Arrays.toString(byteArray));
System.out.println("Hex String Conversion: " + Hex.encodeHexString(byteArray));
}
}
Output:
Byte Array:
[83, 105, 109, 112, 108, 101, 84, 101, 115, 116, 105, 110, 103]
Hex String Conversion: 53696d706c6554657374696e67
DatatypeConverter()
Method to Convert Byte Array in Hex String in Java
Another approach is javax.xml.bind.DatatypeConverter.printHexBinary()
method in Java. This method takes the bytes and converts it into Hex
from an array.
Example Codes:
import java.util.Arrays;
import javax.xml.bind.*;
public class SimpleTesting {
public static void main(String[] args) {
byte byteArray[] = {(byte) 00, (byte) 10, (byte) 20, (byte) 30, (byte) 40};
String hexString = javax.xml.bind.DatatypeConverter.printHexBinary(byteArray);
System.out.println("Byte Array: ");
System.out.println(Arrays.toString(byteArray));
System.out.println("Hex String Conversion: " + hexString);
}
}
Output:
Byte Array:
[0, 10, 20, 30, 40]
Hex String Conversion: 000A141E28
append(.format)
Method for Conversion of Byte Array Into Hex String in Java
Another method that can be used is to include append(.format)
using stringBuilder
in Java. It works by converting every single value from byte array
and convert one by one to hex string
accordingly.
Example Codes:
public class SimpleTesting {
public static void main(String[] args) {
byte a[] = new byte[2];
a[0] = 20;
a[1] = 10;
StringBuilder sb = new StringBuilder(a.length * 2);
for (byte b : a) sb.append(String.format("%02x", b));
System.out.println(sb);
}
}
Output:
140a
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java