How to Remove Duplicates From Array in Java
- Use a Temporary Array to Remove Duplicates From an Array in Java
- Use a Separate Index to Remove Duplicates From an Array in Java
-
Use the
Arrays.sort()
Method to Remove Duplicates From an Array in Java
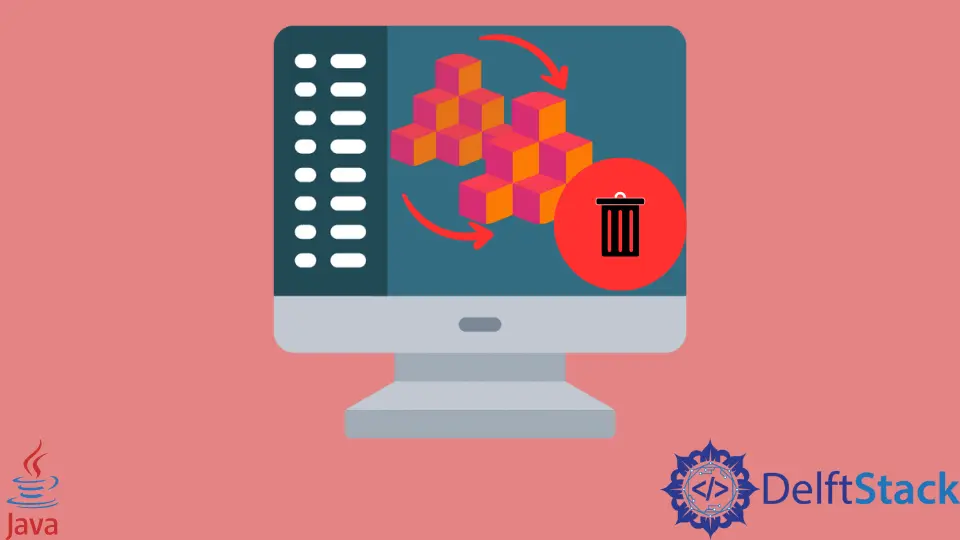
An array is a collection that can store elements of similar types with their fixed memory location assigned to them. An array allows storing duplicate values also.
This tutorial will demonstrate how to efficiently remove duplicates from an array in Java in different ways.
Use a Temporary Array to Remove Duplicates From an Array in Java
In this method, the main point is to traverse the input array and then copy the unique elements from the original array to a temporary array. To achieve this, we will use the for
loop and the if
statement. Finally, the elements from the temporary array are copied to the original array, and we print it.
See the code below.
public class Main{
public static int remove_Duplicate_Elements(int arr[], int n){
if (n==0 || n==1){
return n;
}
int[] tempA = new int[n];
int j = 0;
for (int i=0; i<n-1; i++){
if (arr[i] != arr[i+1]){
tempA[j++] = arr[i];
}
}
tempA[j++] = arr[n-1];
for (int i=0; i<j; i++){
arr[i] = tempA[i];
}
return j;
}
public static void main (String[] args) {
int arr[] = {56,56,77,78,78,98,98};
int length = arr.length;
length = remove_Duplicate_Elements(arr, length);
for (int i=0; i<length; i++)
System.out.print(arr[i]+" ");
}
}
Output:
56 77 78 98
Note that we can implement the above program on sorted arrays only.
Use a Separate Index to Remove Duplicates From an Array in Java
Here, the if
statement is used to check if the element is equal to its next element. If not then, that particular element is added at some index in that similar array only. This is done to show that in the array, this particular element is added only once.
This method is also applicable only if the array is sorted.
We implement this in the following code snippet.
public class Main {
public static int remove_Duplicates(int a[], int n) {
if (n == 0 || n == 1) {
return n;
}
int j = 0;
for (int i = 0; i < n - 1; i++) {
if (a[i] != a[i + 1]) {
a[j++] = a[i];
}
}
a[j++] = a[n - 1];
return j;
}
public static void main(String[] args) {
int a[] = {11, 24, 24, 37, 37, 44, 47, 47, 56, 56};
int n = a.length;
int j = 0;
j = remove_Duplicates(a, n);
for (int i = 0; i < j; i++) System.out.print(a[i] + " ");
}
}
Output:
11 24 37 44 47 56
Use the Arrays.sort()
Method to Remove Duplicates From an Array in Java
The Arrays.sort()
function can sort arrays in Java. This method is not only used for Linked Lists, ArrayLists, etc., but we can also use it for primitive data types.
Time and space complexity is not fixed if we use this method. It totally depends upon the implementation of the code.
For example,
import java.util.Arrays;
public class Main {
public static int remove_Duplicate_Elements(int arr[], int n) {
if (n == 0 || n == 1) {
return n;
}
int[] tempA = new int[n];
int j = 0;
for (int i = 0; i < n - 1; i++) {
if (arr[i] != arr[i + 1]) {
tempA[j++] = arr[i];
}
}
tempA[j++] = arr[n - 1];
for (int i = 0; i < j; i++) {
arr[i] = tempA[i];
}
return j;
}
public static void main(String[] args) {
int arr[] = {11, 7, 2, 2, 7, 8, 8, 8, 3};
Arrays.sort(arr);
int length = arr.length;
length = remove_Duplicate_Elements(arr, length);
for (int i = 0; i < length; i++) System.out.print(arr[i] + " ");
}
}
Output:
2 3 7 8 11
As observed, the duplicate elements from the unsorted arrays are also removed in the same manner as sorted arrays. But here, one additional method is used to sort the unsorted array.
It is to be noted that one should check whether the array is sorted or not and then proceed with the next step of removing the duplicates.