How to Concatenate Two Arrays in Java
-
ArrayUtil.addAll()
Method to Concatenate Two Arrays in Java -
Use the
arraycopy()
Method to Concatenate Two Arrays in Java -
Incremental
Method to Concatenate Arrays in Java
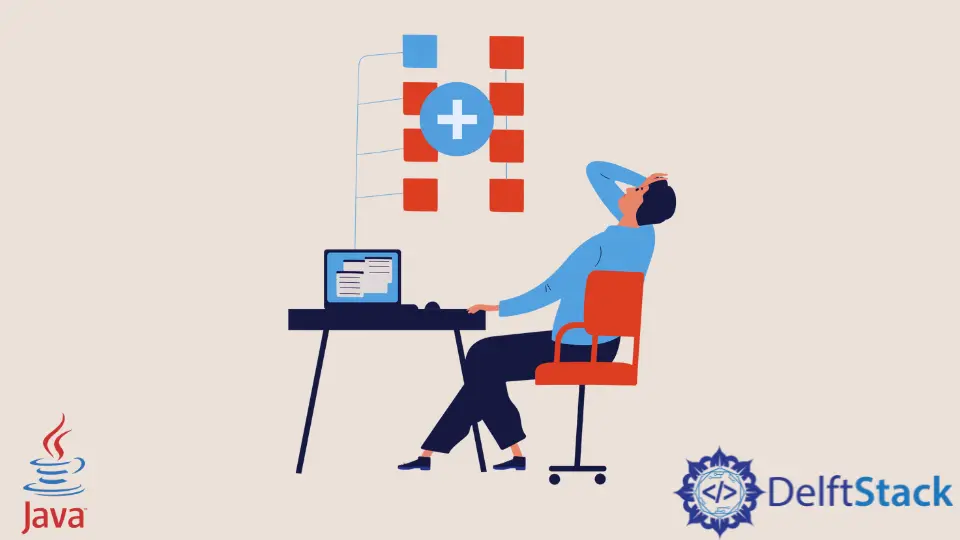
In this tutorial, we will see how to concatenate two arrays in Java. This can be done using different methods depending upon the requirement. In some cases, the user needs to perform duplication as well before merging arrays; as per requirement.
ArrayUtil.addAll()
Method to Concatenate Two Arrays in Java
The first method is ArrayUtil.addAll()
. It takes the values of arrays and merges them into one. Since this method is commons
of apache
; hence in order to use this method, apache.commons.lang3
method needs to be imported first into the compiler.
Example Codes:
import java.util.Arrays;
import org.apache.commons.lang3.ArrayUtils;
public class SimpleTesting {
public static void main(String[] args) {
int[] Array1 = new int[] {00, 10, 20, 30, 40, 50};
int[] Array2 = new int[] {60, 70, 80, 90, 100};
int[] Concate = ArrayUtils.addAll(Array1, Array2);
System.out.println("Array1: " + Arrays.toString(Array1));
System.out.println("Array2: " + Arrays.toString(Array2));
System.out.println("Concatenated Array: " + Arrays.toString(Concate));
}
}
Output:
Array1: [0, 10, 20, 30, 40, 50]
Array2: [60, 70, 80, 90, 100]
Concatenated Array: [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
Use the arraycopy()
Method to Concatenate Two Arrays in Java
Another method to concatenate two arrays in Java is arraycopy()
method. This method takes the values of arrays and merges them into one. The below example shows how this can be done for integer arrays.
Example Codes:
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] Array1 = {00, 10, 20, 30, 40, 50};
int[] Array2 = {60, 70, 80, 90, 100};
int lenArray1 = Array1.length;
int lenArray2 = Array2.length;
int[] concate = new int[lenArray1 + lenArray2];
System.arraycopy(Array1, 0, concate, 0, lenArray1);
System.arraycopy(Array2, 0, concate, lenArray1, lenArray2);
System.out.println("Array1: " + Arrays.toString(Array1));
System.out.println("Array2: " + Arrays.toString(Array2));
System.out.println("Concatenated Array: " + Arrays.toString(concate));
}
}
Output:
Array1: [0, 10, 20, 30, 40, 50]
Array2: [60, 70, 80, 90, 100]
Concatenated Array: [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100]
Incremental
Method to Concatenate Arrays in Java
Another method to perform arrays concatenation is to use the incremental method which is a manual code using for
loop. It works on the positioning of values inside individual arrays to be placed sequentially inside the concatenated one. The below method shows the working of integer arrays.
Example Codes:
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
int[] Array1 = {00, 10, 20, 30, 40, 50};
int[] Array2 = {60, 70, 80, 90, 100};
int len = Array1.length + Array2.length;
int[] Concate = new int[len];
int position = 0;
for (int object : Array1) {
Concate[position] = object;
position++;
}
for (int object : Array2) {
Concate[position] = object;
position++;
}
System.out.println("Array1: " + Arrays.toString(Array1));
System.out.println("Array2: " + Arrays.toString(Array2));
System.out.println("Concatenated Array: " + Arrays.toString(Concate));
}
}
Output:
Array1: [0, 10, 20, 30, 40, 50]
Array2: [60, 70, 80, 90, 100]
Concatenated Array: [0, 10, 20, 30, 40, 50, 60, 70, 80, 90, 100]