How to Count Repeated Elements in an Array in Java
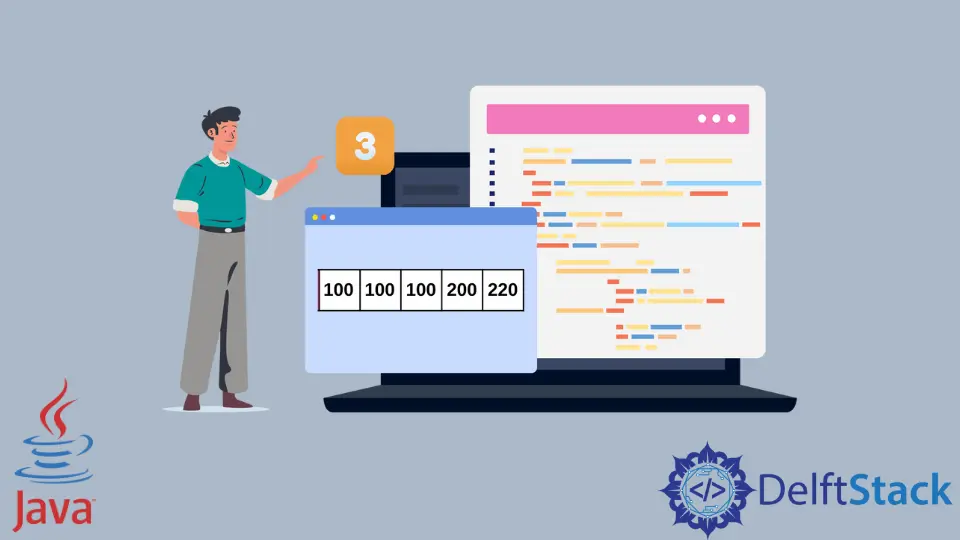
This tutorial demonstrates the method of counting repeated elements in an array in Java.
Count Repeated Elements in an Array in Java
We can create a program to count the duplicate elements in an array. The array can either be unsorted or sorted.
This tutorial demonstrates methods to count repeated elements in sorted and unsorted arrays.
Follow the steps below to count the repeated elements in an array:
-
First of all, get the input array.
-
Then, create one temporary array.
-
The next step is to traverse through the input array.
-
During traversing, check if the current element is present in the temporary array. Then we need to skip checking for the current element.
-
If the current element is unavailable, keep comparing the current element and all the next elements.
-
If, at any stage, a match is found, add that element to the temporary array.
-
The final step is to display the total repeated elements from the temp array.
Let’s implement the above steps in the Java code:
package delftstack;
public class Example {
public static void main(String[] args) {
int InputArray[] = {100, 220, 100, 400, 200, 100, 200, 600, 400, 700};
int TemporaryArray[] = new int[InputArray.length];
int RepeatCount = 0;
for (int x = 0; x < InputArray.length; x++) {
int element = InputArray[x];
boolean flag = false;
for (int y = 0; y < RepeatCount; y++) {
if (TemporaryArray[y] == element) {
flag = true;
break;
}
}
if (flag) {
continue;
}
for (int y = x + 1; y < InputArray.length; y++) {
if (InputArray[y] == element) {
TemporaryArray[RepeatCount++] = element;
break;
}
}
}
System.out.println("The Total Repeated elements in the array: " + RepeatCount);
System.out.println("The Repeated elements are : ");
for (int x = 0; x < RepeatCount; x++) {
System.out.print(TemporaryArray[x] + " ");
}
}
}
The code above will count the repeated numbers and then also print them. See the output:
The Total Repeated elements in the array: 3
The Repeated elements are :
100 400 200
The above can work for both sorted and unsorted arrays. But for more simplification, we can create a program that will only work for the sorted arrays.
See the example:
package delftstack;
public class Example {
public static void main(String[] args) {
int InputArray[] = {100, 100, 100, 200, 200, 220, 400, 400, 600, 700};
int TemporaryArray[] = new int[InputArray.length];
int RepeatCount = 0;
for (int x = 1; x < InputArray.length; x++) {
int element = InputArray[x];
if (element == TemporaryArray[RepeatCount]) {
continue;
}
for (int y = x + 1; y < InputArray.length; y++) {
if (InputArray[y] == element) {
TemporaryArray[RepeatCount++] = element;
break;
}
}
}
System.out.println("The Total Repeated elements in the array: " + RepeatCount);
System.out.println("The Repeated elements are : ");
for (int x = 0; x < RepeatCount; x++) {
System.out.print(TemporaryArray[x] + " ");
}
}
}
Now, this program will work fine for the sorted array. It is the more simplified version.
See the output:
The Total Repeated elements in the array: 3
The Repeated elements are :
100 200 400
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook