Contar elementos repetidos en una matriz en Java
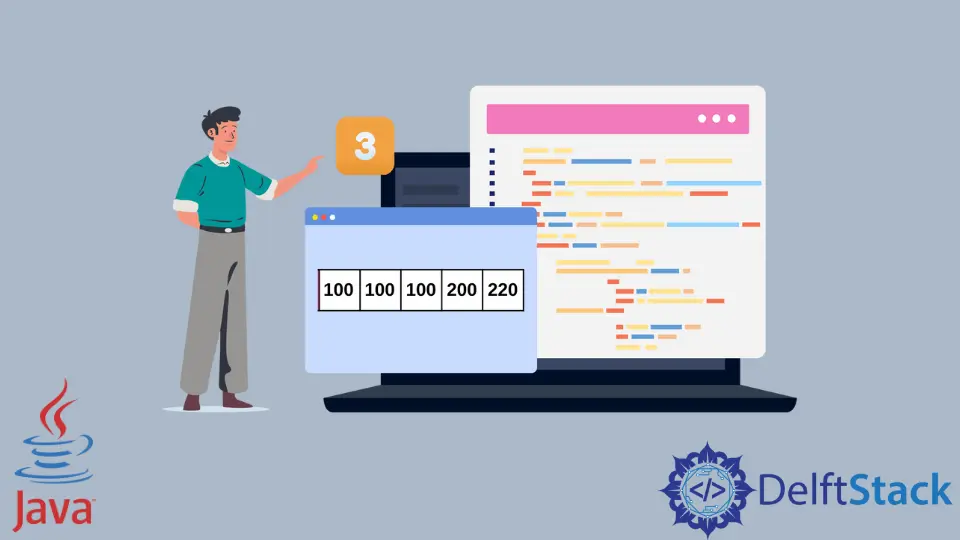
Este tutorial demuestra el método de contar elementos repetidos en una matriz en Java.
Contar elementos repetidos en una matriz en Java
Podemos crear un programa para contar los elementos duplicados en una matriz. La matriz puede estar ordenada o no ordenada.
Este tutorial demuestra métodos para contar elementos repetidos en matrices ordenadas y no ordenadas.
Siga los pasos a continuación para contar los elementos repetidos en una matriz:
-
En primer lugar, obtenga la matriz de entrada.
-
Luego, cree una matriz temporal.
-
El siguiente paso es atravesar la matriz de entrada.
-
Durante el recorrido, verifique si el elemento actual está presente en la matriz temporal. Luego, debemos omitir la verificación del elemento actual.
-
Si el elemento actual no está disponible, siga comparando el elemento actual y todos los elementos siguientes.
-
Si, en cualquier etapa, se encuentra una coincidencia, agregue ese elemento a la matriz temporal.
-
El paso final es mostrar el total de elementos repetidos de la matriz temporal.
Implementemos los pasos anteriores en el código Java:
package delftstack;
public class Example {
public static void main(String[] args) {
int InputArray[] = {100, 220, 100, 400, 200, 100, 200, 600, 400, 700};
int TemporaryArray[] = new int[InputArray.length];
int RepeatCount = 0;
for (int x = 0; x < InputArray.length; x++) {
int element = InputArray[x];
boolean flag = false;
for (int y = 0; y < RepeatCount; y++) {
if (TemporaryArray[y] == element) {
flag = true;
break;
}
}
if (flag) {
continue;
}
for (int y = x + 1; y < InputArray.length; y++) {
if (InputArray[y] == element) {
TemporaryArray[RepeatCount++] = element;
break;
}
}
}
System.out.println("The Total Repeated elements in the array: " + RepeatCount);
System.out.println("The Repeated elements are : ");
for (int x = 0; x < RepeatCount; x++) {
System.out.print(TemporaryArray[x] + " ");
}
}
}
El código anterior contará los números repetidos y luego también los imprimirá. Ver la salida:
The Total Repeated elements in the array: 3
The Repeated elements are :
100 400 200
Lo anterior puede funcionar tanto para matrices ordenadas como no ordenadas. Pero para una mayor simplificación, podemos crear un programa que solo funcione para las matrices ordenadas.
Ver el ejemplo:
package delftstack;
public class Example {
public static void main(String[] args) {
int InputArray[] = {100, 100, 100, 200, 200, 220, 400, 400, 600, 700};
int TemporaryArray[] = new int[InputArray.length];
int RepeatCount = 0;
for (int x = 1; x < InputArray.length; x++) {
int element = InputArray[x];
if (element == TemporaryArray[RepeatCount]) {
continue;
}
for (int y = x + 1; y < InputArray.length; y++) {
if (InputArray[y] == element) {
TemporaryArray[RepeatCount++] = element;
break;
}
}
}
System.out.println("The Total Repeated elements in the array: " + RepeatCount);
System.out.println("The Repeated elements are : ");
for (int x = 0; x < RepeatCount; x++) {
System.out.print(TemporaryArray[x] + " ");
}
}
}
Ahora, este programa funcionará bien para la matriz ordenada. Es la versión más simplificada.
Ver la salida:
The Total Repeated elements in the array: 3
The Repeated elements are :
100 200 400
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook