Natural Ordering in Java
-
the
naturalOrder()
Function in Java -
General Use of the
naturalOrder()
Function in Java -
Use the
nullsFirst()
Function With thenaturalOrder()
Function in Java -
Use the
nullsLast()
Function With thenaturalOrder()
Function in Java
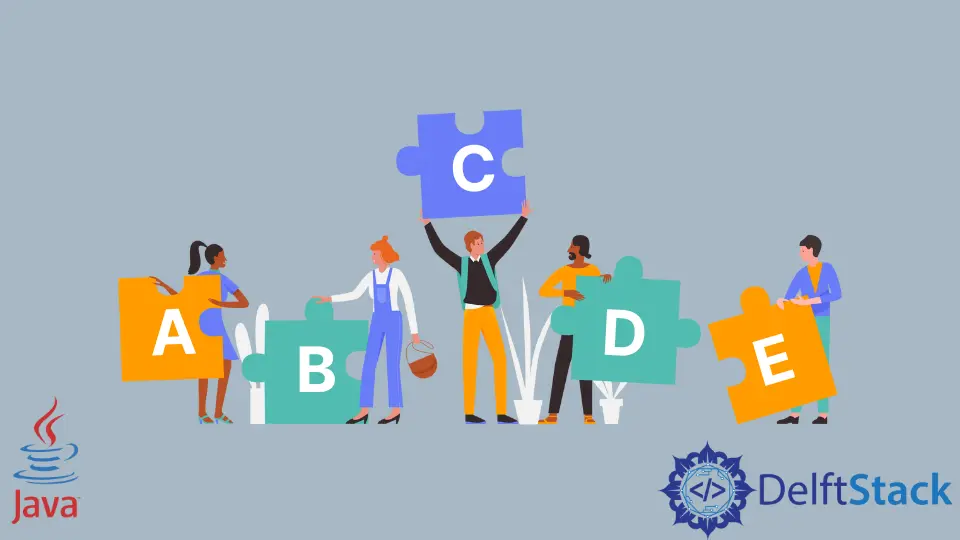
If you are a programmer, you should have experience working with arrays. Sorting an array is a common operation used to reorganize the array in a specified order.
The most commonly used order in Java is the Natural Order. This article will show how we can use the naturalOrder()
function to order an array.
Also, we will see necessary examples and explanations to make the topic easier.
the naturalOrder()
Function in Java
naturalOrder()
is a function from the Comparator
interface in Java. It is a function that’s purpose is to compare the element of an array or a collection of objects.
It can be passed by Array.sort()
or Collections.sort()
. We can also use this for reordering the array elements.
In addition, we can also use the nullsFirst()
and the nullsLast()
methods with the naturalOrder()
method you have null values in your array.
General Use of the naturalOrder()
Function in Java
In the example, we will see the general use of the naturalOrder()
function. We ordered an array with some simple data.
The code for our example will be as follows.
import java.util.Arrays;
import java.util.Comparator;
class Main {
public static void main(String[] args) {
String[] str = {"B", "C", "A", "E", "G"};
Arrays.sort(str, Comparator.naturalOrder());
System.out.println(Arrays.toString(str));
}
}
After taking a string array with some values, we sorted the array by using the line Arrays.sort(str, Comparator.naturalOrder());
, and lastly, we printed the array.
The output is shown below:
[A, B, C, E, G]
Use the nullsFirst()
Function With the naturalOrder()
Function in Java
We can use the nullsFirst()
function with the naturalOrder()
. The function nullsFirst()
will order all the null valued elements of an array first if your array contains some null value,
To understand this, let’s consider the below example:
import java.util.Arrays;
import java.util.Comparator;
class Main {
public static void main(String[] args) {
String[] str = {"B", "C", null, "A", "E", "G"};
Arrays.sort(str, Comparator.nullsFirst(Comparator.naturalOrder()));
System.out.println(Arrays.toString(str));
}
}
After taking a string array with some values, we sorted the array by using the line:
Arrays.sort(str, Comparator.nullsFirst(Comparator.naturalOrder()));
And lastly, we printed the array. The output should look like the following:
[null, A, B, C, E, G]
Use the nullsLast()
Function With the naturalOrder()
Function in Java
Also, we can use the nullsLast()
function with naturalOrder()
. The function nullsLast()
will order all the null valued elements of an array last if your array contains some null value.
To understand this, let’s consider the below example:
import java.util.Arrays;
import java.util.Comparator;
class Main {
public static void main(String[] args) {
String[] str = {"B", "C", null, "A", "E", "G"};
Arrays.sort(str, Comparator.nullsLast(Comparator.naturalOrder()));
System.out.println(Arrays.toString(str));
}
}
After taking a string array with some values, we sorted the array using the line:
Arrays.sort(str, Comparator.nullsLast(Comparator.naturalOrder()));
And lastly, we printed the array. The output will look like the following:
[A, B, C, E, G, null]
Please note that the code examples shared here are in Java, and you must install Java on your environment if your system doesn’t contain Java.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn