How to Slice an Array in Java
- Slice an Array in Java by Duplicating Elements
-
Slice an Array in Java Using the
copyOfRange()
Method - Slice an Array in Java Using Java 8 Stream
-
Slice an Array in Java Using the
System.arraycopy
Method -
Slice an Array in Java Using
asList
andsubList
- Conclusion
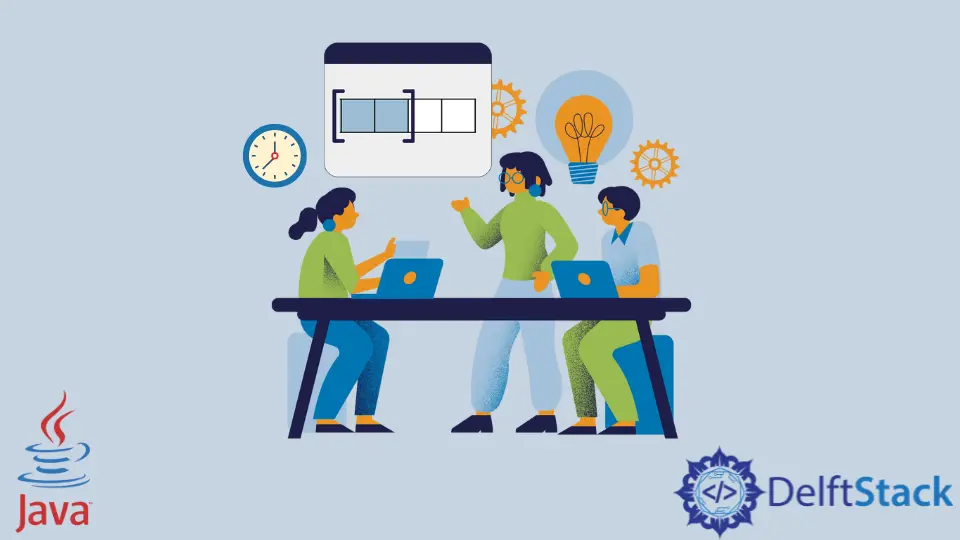
Array manipulation is a fundamental aspect of Java programming, and the ability to efficiently extract specific segments, or slices, from an array is a valuable skill. Whether you’re working with numerical data, strings, or custom objects, understanding the various methods available for array slicing can greatly enhance your coding efficiency.
In this article, we will discuss multiple techniques to slice arrays in Java. From traditional looping methods to leveraging built-in Java functions and Java 8 Streams, this article will provide you with a toolbox of approaches suited for different scenarios.
Slice an Array in Java by Duplicating Elements
Array slicing involves creating a new array that holds a subset of elements from the original array. This subset can be a continuous range or a set of elements based on specific conditions.
While Java provides standard methods for array manipulation, custom slicing operations can be useful in certain scenarios. Duplicating elements during the slicing process allows for more advanced manipulations without affecting the original array.
The process of array slicing by duplicating elements follows a straightforward set of steps:
-
Start with the declaration of the original array that you wish to slice.
-
Identify the indices that mark the beginning and end of the desired subarray within the original array.
-
Generate a new array to store the sliced elements. The size of this array is determined by subtracting the start index from the end index.
-
Use a loop to copy the elements from the original array to the new sliced array, starting from the specified start index.
-
Finally, return the newly created array containing the sliced elements.
Let’s now examine a Java program that demonstrates array slicing by duplicating elements.
import java.util.Arrays;
public class Copy {
public static int[] getSlice(int[] arr, int stIndx, int enIndx) {
int[] slicedArr = new int[enIndx - stIndx];
for (int i = 0; i < slicedArr.length; i++) {
slicedArr[i] = arr[stIndx + i];
}
return slicedArr;
}
public static void main(String args[]) {
int[] arr = {20, 65, 87, 19, 55, 93, 76, 98, 54, 21};
int stIndx = 2, enIndx = 6;
int[] slicedArr = getSlice(arr, stIndx, enIndx + 1);
System.out.println("Slice of an Array: " + Arrays.toString(slicedArr));
}
}
The getSlice
method is defined to perform the array slicing by duplicating elements. It takes three parameters: the original array (arr
), the start index (stIndx
), and the end index (enIndx
).
Inside the method, an empty array (slicedArr
) is created with a size equal to the difference between the end and start indices.
Then, a for
loop iterates through the elements, copying them from the original array to the sliced array. The sliced array is then returned.
In the main
method, we provide a sample original array (arr
), set the start and end indices (stIndx
and enIndx
), call the getSlice
method to obtain the sliced array, and finally, print the result using Arrays.toString()
.
Output:
Slice of an Array: [87, 19, 55, 93, 76]
This output represents the sliced array containing elements from index 2 to index 6 (inclusive) of the original array.
Slice an Array in Java Using the copyOfRange()
Method
Let’s discuss another method for array slicing using the copyOfRange()
method provided by the Java Arrays
class. This method simplifies the process of creating a subarray by directly specifying the range of elements to include.
The copyOfRange()
method is a static method provided by the java.util.Arrays
class in Java.
Its syntax is as follows:
public static T[] copyOfRange(T[] original, int from, int to)
Where:
T[] original
: The original array from which a range of elements is to be copied. The type parameterT
represents the type of elements in the array.int from
: The starting index (inclusive) of the range to be copied.int to
: The ending index (exclusive) of the range to be copied.
The copyOfRange()
method returns a new array containing the specified range of elements from the original array. The type of elements in the returned array is the same as the type of elements in the original array.
It’s important to note that the to
index is exclusive, meaning the element at the to
index itself is not included in the copied range. The method ensures that the indices are within the bounds of the original array, and it may throw exceptions such as ArrayIndexOutOfBoundsException
or IllegalArgumentException
if the indices are invalid.
Now, let’s dive into a detailed example to demonstrate this method.
import java.util.Arrays;
public class Copy1 {
public static int[] slice(int[] arr, int stIndx, int enIndx) {
int[] slicedArr = Arrays.copyOfRange(arr, stIndx, enIndx);
return slicedArr;
}
public static void main(String args[]) {
int[] arr = {20, 65, 87, 19, 55, 93, 76, 98, 54, 21};
int stIndx = 3, enIndx = 8;
int[] slicedArr = slice(arr, stIndx, enIndx);
System.out.println("Slice of Array: " + Arrays.toString(slicedArr));
}
}
In the slice
method, the Arrays.copyOfRange()
method is employed to create a subarray. This method takes three parameters: the original array (arr
), the start index (stIndx
), and the end index (enIndx
).
Internally, this method handles the creation of a new array and copies the specified range of elements from the original array to the new one.
In the main
method, we provide a sample original array (arr
), set the start and end indices (stIndx
and enIndx
), call the slice
method to obtain the sliced array, and finally, print the result using Arrays.toString()
.
Output:
Slice of Array: [19, 55, 93, 76, 98]
This output represents the sliced array containing elements from index 3 to index 8 of the original array, demonstrating the effectiveness of the copyOfRange()
method for array slicing in Java.
Slice an Array in Java Using Java 8 Stream
In modern Java development, the Stream API introduced in Java 8 provides a powerful and concise way to manipulate collections, including arrays.
The Stream API provides a declarative way to process elements in a collection. For array slicing, we can use the Arrays.stream()
method to create a stream from the original array and then apply various stream operations to achieve the desired slice.
The approach involves several steps to obtain a sliced array using Java 8 Stream:
-
Determine the start and end indices that define the range of elements to be included in the sliced array.
-
Utilize the
IntStream.range()
method to generate a stream of indices within the specified range. -
Apply the
map()
function to convert the indices into corresponding elements from the original array. -
Use the
toArray()
method to convert the stream of elements into an array. -
Store the result in a variable to obtain the sliced array.
Now, let’s dive into a detailed example to illustrate how this method works.
import java.util.Arrays;
import java.util.stream.IntStream;
public class NewClice {
public static int[] findSlice(int[] arr, int stIndx, int enIndx) {
int[] sliceArr = IntStream.range(stIndx, enIndx).map(i -> arr[i]).toArray();
return sliceArr;
}
public static void main(String args[]) {
int[] arr = {20, 65, 87, 19, 55, 93, 76, 98, 54, 21, 657};
int stIndx = 2, enIndx = 8;
int[] sliceArr = findSlice(arr, stIndx, enIndx + 1);
System.out.println("Slice of array for the specific range is: " + Arrays.toString(sliceArr));
}
}
In the findSlice
method, the Java 8 Stream API is employed to create a subarray. The IntStream.range(stIndx, enIndx)
generates a stream of indices within the specified range.
The map(i -> arr[i])
function maps each index to the corresponding element from the original array. Finally, the toArray()
method converts the stream of elements into an array.
In the main
method, we provide a sample original array (arr
), set the start and end indices (stIndx
and enIndx
), call the findSlice
method to obtain the sliced array, and finally, print the result using Arrays.toString()
.
Output:
Slice of array for the specific range is: [87, 19, 55, 93, 76, 98, 54]
This output represents the sliced array containing elements from index 2 to index 8 (inclusive) of the original array, showcasing the concise and expressive nature of Java 8 Stream for array slicing.
Slice an Array in Java Using the System.arraycopy
Method
A traditional and efficient method for array slicing in Java is utilizing the System.arraycopy
method.
The System.arraycopy
method is part of the System
class and is designed specifically for efficiently copying data between arrays. This method offers a direct and low-level approach to array manipulation.
Unlike some other methods, System.arraycopy
allows for efficient bulk copying of elements between arrays, making it a practical choice for array manipulation.
The System.arraycopy
method in Java has the following syntax:
public static void arraycopy(Object src, int srcPos, Object dest, int destPos, int length)
Where:
src
: The source array from which elements will be copied.srcPos
: The starting position in the source array from which to begin copying.dest
: The destination array where elements will be copied.destPos
: The starting position in the destination array where elements will be copied.length
: The number of elements to be copied from the source array to the destination array.
This method is used for efficient array copying, allowing you to copy a range of elements from one array to another. It handles various scenarios, such as copying between arrays of different types, copying overlapping ranges, and more.
It is a static method of the System
class in Java and is often used for array manipulation and slicing.
Let’s explore a comprehensive example to illustrate the use of System.arraycopy
for array slicing.
import java.util.Arrays;
public class ArrayCopyExample {
public static int[] sliceArray(int[] originalArray, int startIndex, int endIndex) {
int length = endIndex - startIndex;
int[] slicedArray = new int[length];
System.arraycopy(originalArray, startIndex, slicedArray, 0, length);
return slicedArray;
}
public static void main(String[] args) {
int[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int startIndex = 2;
int endIndex = 7;
int[] slicedArray = sliceArray(originalArray, startIndex, endIndex);
System.out.println("Sliced Array: " + Arrays.toString(slicedArray));
}
}
In the sliceArray
method, we start by calculating the length of the subarray, which is essential for creating the new array. An empty array (slicedArray
) is then created with the calculated length.
Here, the System.arraycopy
method is employed to efficiently copy the specified range of elements from the originalArray
to the slicedArray
. The method parameters include the source array, start index in the source array, destination array, destination index in the destination array, and the length of the copied region. The slicedArray
is then returned.
In the main
method, we provide a sample originalArray
, set the start and end indices for slicing, call the sliceArray
method to obtain the sliced array, and finally, print the result using Arrays.toString()
.
Output:
Sliced Array: [3, 4, 5, 6, 7]
This output represents the sliced array containing elements from index 2 to index 7 (exclusive) of the original array, showcasing the effectiveness of System.arraycopy
for array slicing in Java.
Slice an Array in Java Using asList
and subList
Java provides another convenient way to slice an array using the asList
method from the Arrays
class and the subList
method from the List
interface. This method allows you to work with arrays more easily by converting them to lists and then extracting sublists based on specified indices.
The syntax of the asList
method is as follows:
public static <T> List<T> asList(T... a)
Where:
T
: The type of the elements in the array.a
: The array to be converted to a list.
This method returns a fixed-size list backed by the specified array. Any changes to the returned list will be reflected in the original array and vice versa.
On the other hand, the syntax of the subList
method is as follows:
List<E> subList(int fromIndex, int toIndex)
Where:
E
: The type of elements in the list.fromIndex
: The starting index (inclusive) of the sublist.toIndex
: The ending index (exclusive) of the sublist.
This method returns a view of the portion of the list between the specified fromIndex
(inclusive) and toIndex
(exclusive). Changes to the sublist will be reflected in the original list and vice versa.
The process involves the following steps:
-
Use
Arrays.asList
to convert the original array into a list. This method returns a fixed-size list backed by the original array. -
Apply the
subList
method on the list, specifying the start and end indices to obtain a view of the desired sublist. -
Convert the sublist back to an array using
toArray
to obtain the sliced array.
Here’s a comprehensive example to illustrate this method.
import java.util.Arrays;
import java.util.List;
public class ListSliceExample {
public static Integer[] sliceArray(Integer[] originalArray, int startIndex, int endIndex) {
List<Integer> originalList = Arrays.asList(originalArray);
List<Integer> slicedList = originalList.subList(startIndex, endIndex);
Integer[] slicedArray = slicedList.toArray(new Integer[0]);
return slicedArray;
}
public static void main(String[] args) {
Integer[] originalArray = {1, 2, 3, 4, 5, 6, 7, 8, 9};
int startIndex = 2;
int endIndex = 7;
Integer[] slicedArray = sliceArray(originalArray, startIndex, endIndex);
System.out.println("Sliced Array: " + Arrays.toString(slicedArray));
}
}
In the sliceArray
method, we begin by converting the original array to a list using Arrays.asList
, creating a list view backed by the original array. Next, the subList
method is applied to obtain the desired sublist based on the specified start and end indices.
Finally, the sublist is converted back to an array using toArray
.
In the main
method, a sample originalArray
is provided, and start and end indices for slicing are set. The sliceArray
method is then called to obtain the sliced array, which is printed using Arrays.toString()
.
Output:
Sliced Array: [3, 4, 5, 6, 7]
This output represents the sliced array containing elements from index 2 to index 7 (exclusive) of the original array, showcasing the simplicity and convenience of using asList
and subList
for array slicing in Java.
Conclusion
Java offers a variety of methods for efficiently slicing arrays, each catering to different preferences and use cases. Whether through traditional loops, stream operations, or leveraging built-in methods like System.arraycopy
, asList
, and subList
, you have the flexibility to choose the method that aligns best with your coding style and project requirements.
Understanding these methods allows you to manipulate arrays with ease, facilitating the extraction of specific subsets and enhancing the overall efficiency and readability of their code.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn