How to Remove Substring From String in Java
-
Use the
replace()
Method to Remove a Substring From a String in Java -
Use the
StringBuffer.replace()
Method to Remove a Substring From a String in Java -
Use the
replaceAll()
Method to Remove a Substring From a String in Java -
Use the
substring()
Method to Remove a Substring From a String in Java - Use the Apache Commons Lang Library to Remove a Substring From a String in Java
- Conclusion
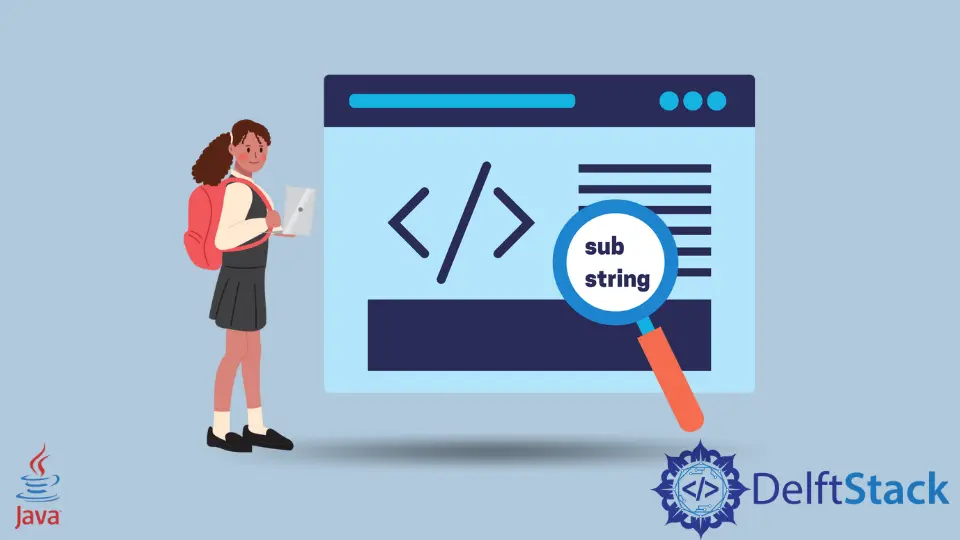
String manipulation is a fundamental aspect of Java programming, and there are various techniques to tailor strings to specific requirements. One common task is removing unwanted substrings from a given string.
In this article, we will explore several methods to achieve this in Java, ranging from the classic replace()
method to more advanced techniques using regular expressions and the Apache Commons Lang library.
Use the replace()
Method to Remove a Substring From a String in Java
One widely used method for removing a specific substring from a given string is the replace()
method provided by the String
class.
Syntax:
public String replace(char oldChar, char newChar)
Here, oldChar
represents the character or substring to be replaced, and newChar
is the character or substring that will replace the occurrences of oldChar
in the original string. The method returns a new string with the substitutions applied.
Let’s delve into a practical example to see how the replace()
method works:
public class StringManipulation {
public static void main(String[] args) {
String originalString = "This is an example string with some characters to replace.";
char charToRemove = 'a';
char replacementChar = '_';
String modifiedString = originalString.replace(charToRemove, replacementChar);
System.out.println("Original String: " + originalString);
System.out.println("Modified String: " + modifiedString);
}
}
Output:
Original String: This is an example string with some characters to replace.
Modified String: This is _n ex_mple string with some ch_r_cters to repl_ce.
As you can see, we start by declaring a string originalString
containing the text we want to modify. We then specify the character to be removed (charToRemove
) and the character to replace it (replacementChar
).
In this case, we aim to replace all occurrences of the character a
with an underscore (_
).
Then, the replace()
method is invoked on the originalString
. This will effectively replace all the occurrences of the specified character (a
) with the replacement character (_
), and the result is stored in the variable modifiedString
.
Finally, we print both the original and modified strings to the console using System.out.println()
to observe the changes. When you run this code yourself, you will see the output demonstrating the removal of the specified substring from the original string.
Use the StringBuffer.replace()
Method to Remove a Substring From a String in Java
In Java, when it comes to manipulating strings, the StringBuffer
class provides a versatile toolset. One powerful method within this class is replace()
, allowing us to efficiently remove or replace substrings within a specified index range.
The replace()
method in StringBuffer
has the following syntax:
public StringBuffer replace(int start, int end, String str)
Here, start
and end
denote the beginning and ending index of the specified range. Importantly, start
is inclusive, and end
is exclusive, meaning the actual range is [start, end-1]
.
The parameter str
represents the string that will replace the content within the specified range. The method returns the modified StringBuffer
object.
Now, let’s dive into a practical example to understand how the StringBuffer.replace()
method works:
public class StringBufferManipulation {
public static void main(String[] args) {
StringBuffer originalString =
new StringBuffer("This is an example StringBuffer with some characters to replace.");
System.out.println("Original String: " + originalString);
originalString.replace(5, 12, "modified");
System.out.println("Modified String: " + originalString);
}
}
Output:
Original String: This is an example StringBuffer with some characters to replace.
Modified String: This modifiedxample StringBuffer with some characters to replace.
In this example, we begin by declaring a StringBuffer
named originalString
, which contains the text we want to modify.
The critical line is originalString.replace(5, 12, "modified");
, where the replace()
method is invoked on the originalString
. In this instance, it replaces the characters from index 5
to 11
with the string modified
.
The method modifies the originalString
in place, and there is no need to assign the result to a new variable. After the replacement, we print both the original and modified strings to the console using System.out.println()
.
Running this code will display the changes made to the original string through the replace()
method.
The StringBuffer.replace()
method is particularly useful when dealing with mutable strings, allowing for efficient modifications within specified ranges.
Use the replaceAll()
Method to Remove a Substring From a String in Java
Alternatively, we can use the replaceAll()
method, part of the String
class, to replace or remove specific substrings. This is especially useful when dealing with patterns specified by regular expressions.
The replaceAll()
method in Java’s String
class has the following syntax:
public String replaceAll(String regex, String replace)
Here, regex
represents the pattern of a regular expression, and replace
is the string that will replace substrings matching the specified pattern. The method returns a new string with the substitutions applied.
Let’s explore a practical example to understand how the replaceAll()
method works:
public class StringManipulation {
public static void main(String[] args) {
String originalString = "This is an example string with some characters to remove.";
System.out.println("Original String: " + originalString);
String modifiedString = originalString.replaceAll("example", "");
System.out.println("Modified String: " + modifiedString);
}
}
As with the previous examples, we start by declaring a string originalString
containing the text we want to modify. The originalString
is then printed to the console using System.out.println()
, showing the original string before it is modified.
Next. the replaceAll()
method is invoked on the originalString
. In this case, it removes all occurrences of the substring example
by replacing it with an empty string.
The method creates a new string (modifiedString
) with the specified substitutions, leaving the original string unchanged. Afterward, we print both the original and modified strings to the console using System.out.println()
.
Executing this code will demonstrate the removal of the specified substring from the original string.
Output:
Original String: This is an example string with some characters to remove.
Modified String: This is an string with some characters to remove.
Now, in certain scenarios, you may encounter the need to remove substrings that match a specific pattern all at once. The replaceAll()
method in Java, coupled with regular expressions, provides an elegant solution to address such requirements.
public class StringManipulation {
public static void main(String[] args) {
String originalString =
"This example showcases multiple patterns: abcTest123, xyzTest456, and pqrTest789.";
System.out.println("Original String: " + originalString);
String modifiedString = originalString.replaceAll("Test.*?\\b", "");
System.out.println("Modified String: " + modifiedString);
}
}
Output:
Original String: This example showcases multiple patterns: abcTest123, xyzTest456, and pqrTest789.
Modified String: This example showcases multiple patterns: abc, xyz, and pqr.
In this example, we start with a string originalString
containing multiple occurrences of substrings following the pattern Test
combined with any combination of characters and digits.
The critical line here is:
String modifiedString = originalString.replaceAll("Test.*?\\b", "");
Let’s break down the regular expression "Test.*?\\b"
used as the pattern:
"Test"
: This matches the literal charactersTest
.".*?"
: This matches any character (except for a newline) zero or more times, but in a non-greedy manner.\\b
: This represents a word boundary, ensuring that only whole words matching the pattern are considered.
The method then replaces all occurrences of substrings matching this pattern with an empty string, effectively removing them.
replace()
and replaceAll()
methods replace all occurrences. The difference between them is that the replaceAll()
method uses a regular expression pattern.Use the substring()
Method to Remove a Substring From a String in Java
The substring()
method is another approach to removing a specific substring from a given string. This method allows us to extract a portion of a string by specifying the starting and ending indices, and with a bit of creativity, we can utilize it to remove substrings effectively.
The substring()
method in Java’s String
class has the following syntax:
public String substring(int beginIndex) public String substring(int beginIndex, int endIndex)
Here, beginIndex
represents the starting index of the substring, and endIndex
represents the ending index. If endIndex
is not specified, the substring extends to the end of the original string.
The method returns a new string that represents the specified substring.
Let’s delve into an example to understand how the substring()
method can be used to remove a substring:
public class StringManipulation {
public static void main(String[] args) {
String originalString = "This is an example string with a substring to remove.";
System.out.println("Original String: " + originalString);
int startIndex = originalString.indexOf("substring");
int endIndex = startIndex + "substring".length();
String modifiedString =
originalString.substring(0, startIndex) + originalString.substring(endIndex);
System.out.println("Modified String: " + modifiedString);
}
}
Output:
Original String: This is an example string with a substring to remove.
Modified String: This is an example string with a to remove.
In this example, we start with a string originalString
that contains the text we want to modify. The key lines are:
int startIndex = originalString.indexOf("substring");
int endIndex = startIndex + "substring".length();
String modifiedString =
originalString.substring(0, startIndex) + originalString.substring(endIndex);
Where:
startIndex
is determined usingindexOf("substring")
, which finds the starting index of the substring we want to remove.endIndex
is then calculated as the sum ofstartIndex
and the length of the substring.modifiedString
is constructed by concatenating the substring beforestartIndex
and the substring afterendIndex
.
This effectively removes the specified substring from the original string. Finally, we print both the original and modified strings to the console using System.out.println()
.
Use the Apache Commons Lang Library to Remove a Substring From a String in Java
The Apache Commons Lang library offers a set of utilities for enhancing Java’s core functionalities, and among them is StringUtils.remove()
, a convenient method for removing substrings from strings.
The StringUtils.remove()
method in Apache Commons Lang has the following syntax:
public static String remove(String str, String remove)
Here, str
is the original string from which we want to remove a substring, and remove
is the specific substring we wish to eliminate. The method returns a new string with the specified substring removed.
Let’s dive into an example to understand how the StringUtils.remove()
method can simplify substring removal:
import org.apache.commons.lang3.StringUtils;
public class StringManipulation {
public static void main(String[] args) {
String originalString = "This is an example string with a substring to remove.";
System.out.println("Original String: " + originalString);
String modifiedString = StringUtils.remove(originalString, "substring");
System.out.println("Modified String: " + modifiedString);
}
}
Output:
Original String: This is an example string with a substring to remove.
Modified String: This is an example string with a to remove.
In this example, the crucial line is:
String modifiedString = StringUtils.remove(originalString, "substring");
Here, the StringUtils.remove()
method is invoked with originalString
as the target string and substring
as the substring to be removed. The method efficiently removes all occurrences of the specified substring and returns the modified string.
As you can notice, this approach with the Apache Commons Lang library simplifies the code, making it more concise and readable. We can easily remove unwanted substrings without the need for complex index calculations or concatenation.
Conclusion
In the diverse landscape of Java programming, the ability to manipulate strings with precision is a crucial skill. As we’ve seen, there are multiple avenues for removing substrings from a given string, each with its own strengths and use cases.
Whether opting for the simplicity of the replace()
method, the flexibility of regular expressions with replaceAll()
, the index-based approach with substring()
, or the streamlined convenience of the Apache Commons Lang library, developers can choose the method that best aligns with their specific needs.
Armed with these techniques, Java programmers are well-equipped to navigate the intricacies of string manipulation, enhancing the versatility and efficiency of their code.