How to Convert Int to Byte in Java
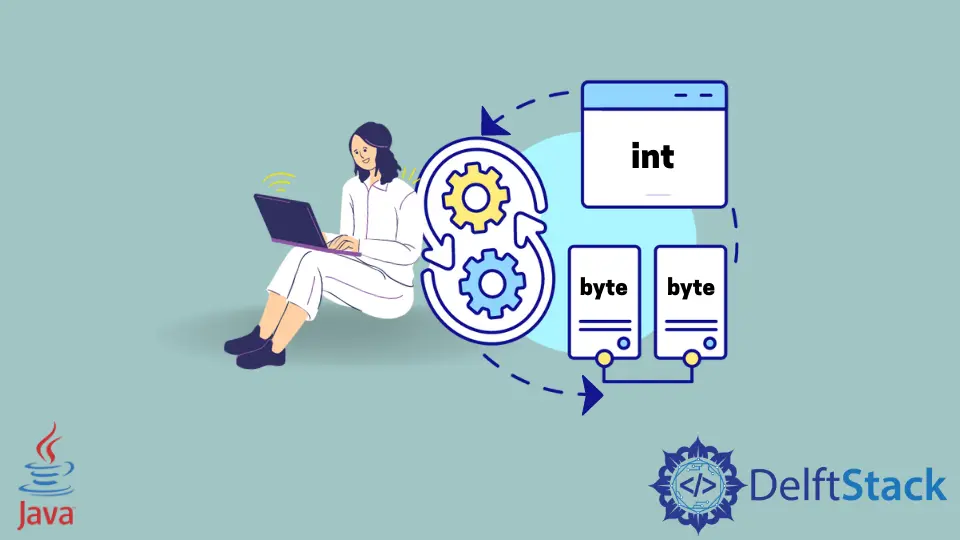
This article explores the significance of converting int
to byte
in Java, shedding light on various methods such as type casting, byteValue()
, and unsigned conversion, offering developers versatile approaches for efficient memory usage and data manipulation.
In Java, int
and byte
are both primitive types and are used to store numeric values. Both are used to store signed and unsigned values but have different storage ranges.
The byte
range is -128 to 127
, and the int
range is -2,147,483,648
to 2,147,483,647
. So, clearly, we can see that int
can store a larger value than the byte
type.
While converting int
to byte
, some data gets lost due to memory. Let’s see some examples.
Importance of Converting int
to byte
Converting int
to byte
in Java is essential for efficient memory usage and data manipulation. It reduces storage space, particularly when dealing with large datasets, and ensures compatibility with byte-oriented data structures.
Additionally, certain operations, like bitwise manipulations, are simplified when working with bytes. Hence, this conversion optimizes resource utilization and facilitates streamlined processing in Java programs.
Methods on How to Convert int
to byte
Type Casting Method
Type casting in converting int
to byte
in Java is crucial for precision and control. It allows an explicit definition of the conversion process, minimizing the risk of data loss or unexpected behavior.
Unlike other methods, type casting provides a clear and direct way to convert integer values to bytes, ensuring a reliable and predictable outcome in Java programs.
Let’s delve into a simple yet effective code example that demonstrates the conversion of an int
to a byte
using type casting:
public class IntToByteConversion {
public static void main(String[] args) {
// Step 1: Define an int value
int intValue = 127;
// Step 2: Convert int to byte using type casting
byte byteValue = (byte) intValue;
// Step 3: Display the results
System.out.println("Original int value: " + intValue);
System.out.println("Converted byte value: " + byteValue);
}
}
In this code breakdown, we begin by defining an int
variable called intValue
with a value of 127
. This value will be the starting point for our conversion to a byte.
The crucial conversion occurs next, employing type casting with the (byte)
syntax to explicitly convert the int
value to a byte
. Finally, to confirm the success of the conversion, we display both the original int
value and the resulting byte
value in the console.
Upon running the code, you can expect the following output:
This output showcases the successful conversion of the int
value to a byte
, highlighting the efficacy of the type casting method in Java. As you explore more complex scenarios, keep in mind the potential for data loss or overflow issues, and choose your conversion methods judiciously based on the specific requirements of your application.
byteValue()
Method
Using byteValue()
in Java for int-to-byte
conversion offers simplicity and readability. It leverages a dedicated method from the Byte
class, providing a clear and direct conversion path.
This method is particularly advantageous in scenarios where code clarity and adherence to object-oriented principles are priorities, offering an efficient alternative to direct casting or bitwise operations.
Let’s dive into a practical example that demonstrates the conversion of an int
to a byte
using the byteValue()
method:
public class IntToByteConversion {
public static void main(String[] args) {
// Step 1: Define an int value
int intValue = 127;
// Step 2: Convert int to byte using byteValue() method
byte byteValue = Byte.valueOf((byte) intValue);
// Step 3: Display the results
System.out.println("Original int value: " + intValue);
System.out.println("Converted byte value: " + byteValue);
}
}
In this code walkthrough, we initiate the process by defining an int
variable named intValue
with a value of 127
. This value serves as the starting point for our conversion to a byte.
The focal point of the conversion lies in the subsequent line, where we utilize the Byte.valueOf()
method. This method takes (byte) intValue
as its argument, producing a Byte
object.
To obtain the primitive byte
value, we then apply the byteValue()
method. Finally, we confirm the successful conversion by printing both the original int
value and the resulting byte
value to the console.
Upon executing the code, you should observe the following output:
This output confirms the successful conversion of the int
value to a byte
using the byteValue()
method. The clarity of this method makes it a valuable tool for developers seeking an elegant and reliable solution for integer-to-byte
conversion in Java.
Unsigned Conversion
Unsigned conversion in Java, such as using bitwise AND
(&
) with 0xFF
, is crucial for representing int
values as bytes without introducing negative interpretations. It ensures accurate byte representation, essential for scenarios involving raw binary data or operations that treat integers as unsigned.
This method provides a clear and direct approach, minimizing the risk of data loss or unexpected behavior, making it valuable in comparison to other conversion methods.
Let’s explore a comprehensive example demonstrating how to convert an int
to a byte
using unsigned conversion:
public class IntToByteUnsignedConversion {
public static void main(String[] args) {
// Step 1: Define an int value
int intValue = 255;
// Step 2: Convert int to byte using unsigned conversion
byte byteValue = unsignedConversion(intValue);
// Step 3: Display the results
System.out.println("Original int value: " + intValue);
System.out.println("Converted byte value: " + byteValue);
}
// Step 4: Implement unsigned conversion method
private static byte unsignedConversion(int value) {
return (byte) (value & 0xFF);
}
}
In our code breakdown, we start by defining an int
variable, intValue
, with a value of 255
. This value acts as the initial integer for our conversion to a byte.
The core conversion occurs in the unsignedConversion()
method, where a bitwise AND
operation is executed between the int
value and the hexadecimal 0xFF
. This operation isolates the least significant 8 bits, effectively representing an unsigned byte.
Finally, to confirm the successful conversion, we print both the original int
value and the resulting byte
value to the console.
Upon executing the code, you should observe the following output:
This output confirms the successful unsigned conversion of the int
value to a byte
. The resulting -1
is a representation of the unsigned byte 255
, showcasing the effectiveness of this method in handling unsigned integers in Java.
Conclusion
Converting int
to byte
in Java is a critical process with implications for memory optimization and efficient data manipulation. The type casting method simplifies this conversion, which is crucial for scenarios demanding smaller memory footprints.
Additionally, the byteValue()
method, associated with the Byte
class, offers readability and convenience, particularly when leveraging class-specific functionalities. Meanwhile, unsigned conversion, employing bitwise AND
with 0xFF
, emerges as a robust technique vital for scenarios requiring the treatment of integers as unsigned entities.
Each method addresses specific needs, providing developers with versatile tools for effective int-to-byte conversion in Java.
Related Article - Java Int
- How to Convert Int to Char in Java
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java