How to Convert Int to Char in Java
-
(Char)
to Convert Int to Char in Java -
Character.forDigit()
to Convertint
tochar
in Java -
toString()
Method to Convert Int to Char in Java
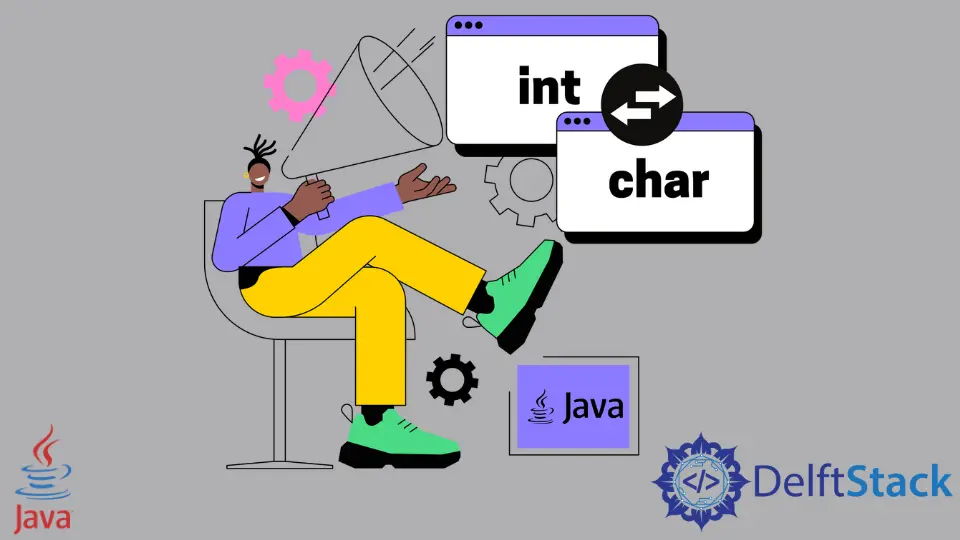
In this tutorial, we will learn methods to convert int
to a char
in Java. The methods could be (char)
, Character.forDigit()
and toString()
.
(Char)
to Convert Int to Char in Java
This method uses TypeCasting
to get the char
of the int
by getting its ASCII value. It can be done with different approaches depending upon requirements.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
int value_int = 65;
char value_char = (char) value_int;
System.out.println(value_char);
}
}
Output:
A
If we put an integer
value in a single quote, it will store the actual character in the char
variable (values should be from 0 to 9):
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
int value_int = '1';
char value_char = (char) value_int;
System.out.println(value_char);
}
}
Output:
1
Another way to do this if the digit is between 0 and 9 is by adding character '0'
to int
:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
int value_int = 1;
char value_char = (char) (value_int + '0');
System.out.println(value_char);
}
}
Output:
1
Character.forDigit()
to Convert int
to char
in Java
To get the actual char
value, we can also use Character.forDigit()
method to convert int
to char
in Java. It determines the character
representation for a specific digit in the specified radix. It returns null if the value of the radix or digit is invalid.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
// radix 10 is for decimal number, for hexa use radix 16
int radix = 10;
int value_int = 6;
char value_char = Character.forDigit(value_int, radix);
System.out.println(value_char);
}
}
Output:
6
To get the hex value, use radix 16
in Character.forDigit()
method.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
// radix 16 is for Hexa number
int radix = 16;
int value_int = 12;
char value_char = Character.forDigit(value_int, radix);
System.out.println(value_char);
}
}
Output:
c
toString()
Method to Convert Int to Char in Java
Another approach is to convert int
to char
in Java is by converting it into string
using the toString()
method and get char
value from that string
.
Example Codes:
// java 1.8
package simpletesting;
public class SimpleTesting {
public static void main(String[] args) {
int value_int = 1;
char value_char = Integer.toString(value_int).charAt(0);
System.out.println(value_char);
}
}
Output:
1
Related Article - Java Int
- How to Convert Int to Double in Java
- List of Ints in Java
- How to Convert Integer to Int in Java
- How to Check if Int Is Null in Java
- How to Convert Int to Byte in Java