Char vs String in Java
- Creating Char in Java
- Creating String in Java
- Char to String Conversion in Java
- String to Char Conversion in Java
- Char Primitive to Character Object in Java
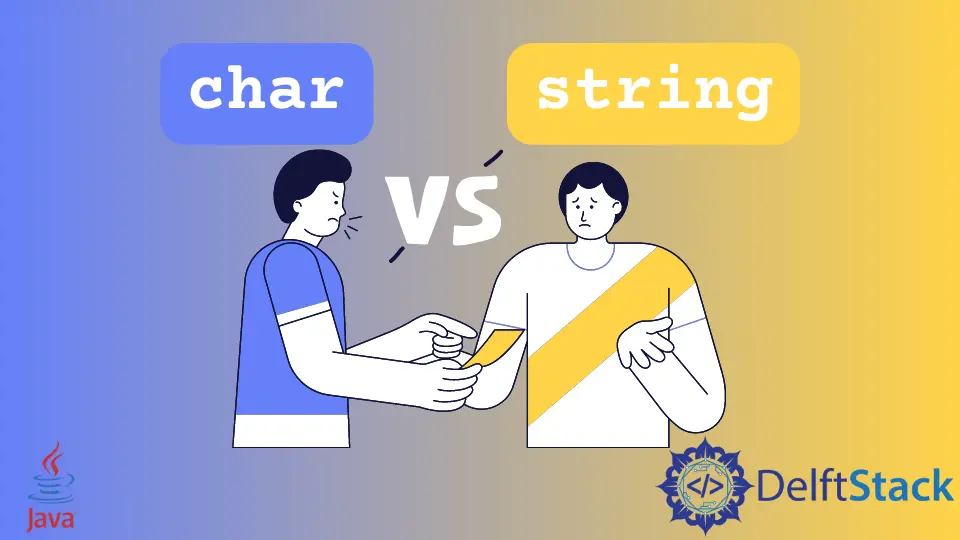
This tutorial introduces the difference between char and String in Java.
In Java, char is a primitive data type that is used to hold a single character. It means a single character of the UTF-16 character set. In comparison, String is a class that holds a sequence of characters and can be thought of as an array of chars.
You can think of a string like Delft
that consists of D
, e
, l
, f
, t
characters. So, basically, a group of char forms a string. Let’s understand with some examples.
Creating Char in Java
In Java, to create a char, we use a single quote that encloses the character. A character can be any of UTF-16. Here, we used a variety of characters to understand the character set. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
char ch1 = 'a';
System.out.println(ch1);
char ch2 = 'A';
System.out.println(ch2);
char ch3 = '1';
System.out.println(ch3);
char ch4 = '@';
System.out.println(ch4);
}
}
Output:
a
A
1
@
Creating String in Java
Here, we create a String by using double quotes. A string can contain any character of the UTF-16 set, such as numbers, special characters, spaces, etc. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
String str1 = "Hello,";
System.out.println(str1);
String str2 = "This is";
System.out.println(str2);
String str3 = "4 Line String";
System.out.println(str3);
String str4 = "Example!";
System.out.println(str4);
}
}
Output:
Hello,
This is
4 Line String
Example!
Char to String Conversion in Java
Char and String both use characters to create, and sometimes when we need to get a string from a char, we can use the toString()
method of character class. This method returns a String object of primitive char value. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
char ch = 'A';
System.out.println(ch);
String str = Character.toString(ch);
System.out.println(str);
}
}
Output:
A
A
String to Char Conversion in Java
Similarly, we can convert a string to char by using the charAt()
method of the String class. This method returns a char of the specified index. We can get any char from the String by just specifying an index value.
public class SimpleTesting {
public static void main(String[] args) {
String str = "Hello";
System.out.println(str);
char ch = str.charAt(0);
System.out.println(ch);
}
}
Output:
Hello
H
Char Primitive to Character Object in Java
If you are working with primitive chars and want to convert them into objects, use the Character class. You just assign the char to Character reference, and Java will do the rest automatically.
When a primitive value gets assigned to a reference, then it automatically converts to an object. This process is called boxing in Java, and its reverse process is called unboxing.
public class SimpleTesting {
public static void main(String[] args) {
char ch = 'A';
System.out.println("primitive char " + ch);
Character chr = ch;
System.out.println("char object " + chr);
char ch2 = chr;
System.out.println("primitive char " + ch2);
}
}
Output:
primitive char A
char object A
primitive char A
Related Article - Java Char
- How to Convert Int to Char in Java
- How to Initialize Char in Java
- How to Represent Empty Char in Java
- How to Convert Char to Uppercase/Lowercase in Java
- How to Check if a Character Is Alphanumeric in Java