How to Check if a Character Is Alphanumeric in Java
-
Check if a Character Is Alphanumeric Using
Character.isLetterOrDigit()
in Java - Check if a Character Is Alphanumeric Using ASCII Values in Java
-
Check if a Character Is Alphanumeric Using
Character.isLetter()
andCharacter.isDigit()
in Java - Check if a Character Is Alphanumeric Using Regular Expressions (Regex) in Java
- Conclusion
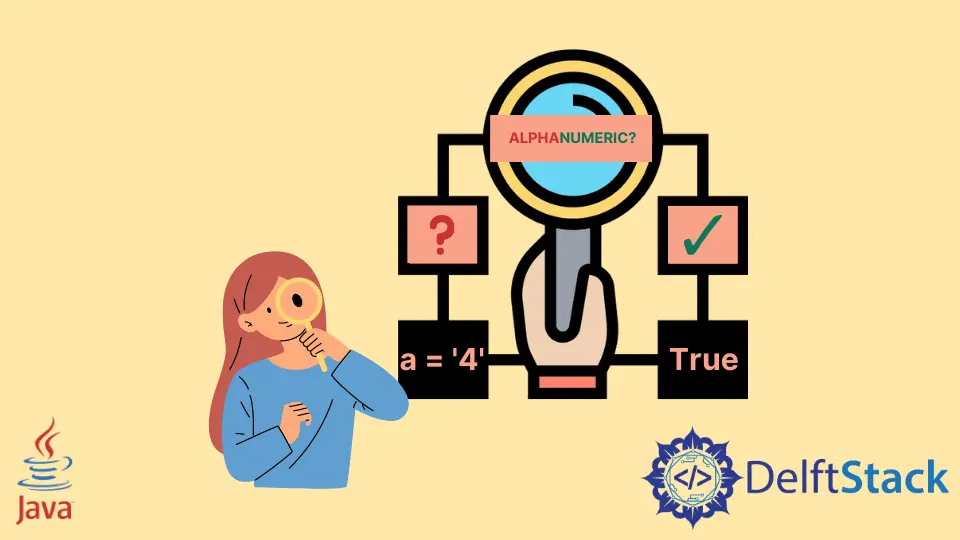
In Java, determining whether a character is alphanumeric (i.e., a letter or a digit) is a common programming task.
In this article, we’ll walk you through the methods and techniques to accomplish this task in Java. By the end of this read, you’ll have a clear grasp of how to tell whether a character falls into the alphanumeric category.
Check if a Character Is Alphanumeric Using Character.isLetterOrDigit()
in Java
The Character.isLetterOrDigit()
method is a convenient tool provided by the Java language that allows us to efficiently accomplish this task. This method checks if a specified character is a letter (a-z or A-Z) or a digit (0-9).
Let’s start by examining a simple example that demonstrates how to use the Character.isLetterOrDigit()
method to check if a character is alphanumeric:
public class CheckCharAlpha {
public static void main(String[] args) {
char a = '4';
boolean letterOrDigit = Character.isLetterOrDigit(a);
System.out.println("Is the character alphanumeric? " + letterOrDigit);
}
}
Output:
Is the character alphanumeric? true
In this example, we declared a character a
with the value '4'
. We then used the Character.isLetterOrDigit()
method to check whether the character is alphanumeric.
As you can see, we passed the character a
as an argument to the Character.isLetterOrDigit()
method. This should return true
if the character is a letter or a digit; otherwise, it should return false
.
Finally, we printed the result, which in this case is true
since the character '4'
is a digit.
Check if a Character Is Alphanumeric Using ASCII Values in Java
Another approach to help determine whether a character is alphanumeric (a letter or a digit) is by using the ASCII values of characters. ASCII values are integer representations of characters, and we can use them to check if a character is alphanumeric.
Let’s begin by examining an example:
public class CheckCharAlpha {
public static void main(String[] args) {
boolean isAlphanumeric = isAlphaNumeric('k');
System.out.println("Is the character alphanumeric? " + isAlphanumeric);
}
public static boolean isAlphaNumeric(char char1) {
return (char1 >= 'a' && char1 <= 'z') || (char1 >= 'A' && char1 <= 'Z')
|| (char1 >= '0' && char1 <= '9');
}
}
Output:
Is the character alphanumeric? true
Here, we declared a character char1
with the value 'k'
. We then used the ASCII values of characters to check if the character is a letter or a digit.
Note that the ASCII values for these characters (a-z, A-Z, 0-9) are within specific ranges, and we can leverage this knowledge to perform the check.
return (char1 >= 'a' && char1 <= 'z') || (char1 >= 'A' && char1 <= 'Z')
|| (char1 >= '0' && char1 <= '9');
The above expression is used to check if the character is a lowercase letter, an uppercase letter, or a digit.
Finally, we printed the result, which in this case is true
since the character 'k'
is a lowercase letter.
Check if a Character Is Alphanumeric Using Character.isLetter()
and Character.isDigit()
in Java
The Character.isLetter()
method in Java is used to determine whether a specified character is a letter (alphabetic character). It considers both uppercase and lowercase letters (a-z, A-Z) as letters.
Method Signature:
public static boolean isLetter(char ch)
Parameter:
ch
- The character to be checked.
Return Value:
- Returns
true
if the specified character is a letter; otherwise, returnsfalse
.
On the other hand, the Character.isDigit()
method in Java is used to determine whether a specified character is a digit (numeric character). It checks for characters from 0 to 9.
Method Signature:
public static boolean isDigit(char ch)
Parameters:
ch
- The character to be checked.
Return Value:
- Returns
true
if the specified character is a digit; otherwise, returnsfalse
.
Here’s a simple example that demonstrates how to use Character.isLetter()
and Character.isDigit()
to check if a character is alphanumeric:
public class CheckCharAlpha {
public static void main(String[] args) {
char a = '4';
boolean isAlphanumeric = Character.isLetter(a) || Character.isDigit(a);
System.out.println("Is the character alphanumeric? " + isAlphanumeric);
}
}
Output:
Is the character alphanumeric? true
In this example, we declared a character a
with the value '4'
. We then used the Character.isLetter()
and Character.isDigit()
methods to check whether the character is a letter or a digit.
The Character.isLetter()
method returns true
if the character is a letter, and the Character.isDigit()
method returns true
if the character is a digit.
Finally, we printed the result, which in this case is true
since the character '4'
is a digit.
Check if a Character Is Alphanumeric Using Regular Expressions (Regex) in Java
In Java, regular expressions, often referred to as regex or regexp, are sequences of characters that form a search pattern. This can also be used to determine whether a character is alphanumeric (a letter or a digit).
Let’s have a simple example that demonstrates how to use a regular expression to check if a character is alphanumeric:
import java.util.regex.*;
public class CheckCharAlpha {
public static void main(String[] args) {
char a = '4';
boolean isAlphanumeric = String.valueOf(a).matches("[a-zA-Z0-9]");
System.out.println("Is the character alphanumeric? " + isAlphanumeric);
}
}
Is the character alphanumeric ? true
In this example, we used the String.valueOf(a).matches("[a-zA-Z0-9]")
method to check whether the character is a letter (a-z or A-Z) or a digit (0-9). The regular expression [a-zA-Z0-9]
matches any alphanumeric character.
We then printed the result of the regular expression match, indicating that the character '4'
is alphanumeric.
Conclusion
To summarize, this article presented multiple methods to determine if a character is alphanumeric in Java. The Character.isLetterOrDigit()
method, ASCII values comparison, and the use of Character.isLetter()
and Character.isDigit()
were demonstrated.
Additionally, it showcased employing regular expressions to achieve the same purpose. These techniques provide us with versatile approaches to efficiently perform the task of character classification into letters or digits in Java programming.
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn