How to Represent Empty Char in Java
- Create an Empty Char in Java
- Create Empty Characters in Java Using Empty String Literal
- Create an Empty Char in Java Using the Character Class
- Create an Empty Char in Java Using the Unicode Null Character
-
Create an Empty Char in Java Using the
MIN_VALUE
Constant - Create an Empty Char in Java Using Apache Commons Lang Library
- Conclusion
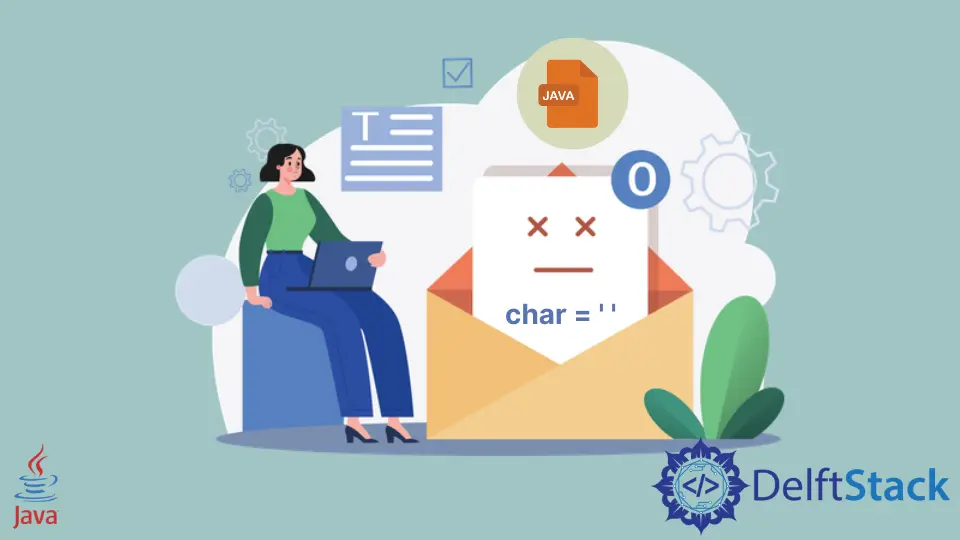
This tutorial will guide you on how to represent an empty char in the Java programming language.
While in Java, it is possible to have an empty char[]
array, the concept of an empty char itself is not applicable. This is because the term char
inherently denotes a character, and an empty char lacks meaningful representation.
Attempting to create an empty char results in a compile-time error, as an empty char value doesn’t align with the nature of a char.
Create an Empty Char in Java
Let’s explore an example where we attempt to create an empty char, resulting in a compile-time error:
public class EmptyCharExample {
public static void main(String[] args) {
char ch = '';
System.out.println(ch);
}
}
Output:
EmptyCharExample.java:3: error: empty character literal
char ch = '';
^
1 error
The compiler error indicates that the attempted creation of an empty char using single quotes is invalid. Java does not recognize the concept of an empty char through the conventional means of assigning an empty character constant.
However, all is not lost, as we can navigate this limitation by assigning a char with a space, resulting in successful compilation and the printing of an empty space to the console.
public class EmptyCharExample {
public static void main(String[] args) {
char ch = ' ';
System.out.println(ch);
}
}
It is important to differentiate between a space char and an empty char, as Java treats them distinctively. While a space character is a valid assignment, denoting the presence of a character with a space, an empty char remains an uncharted territory in Java’s character constants.
Create Empty Characters in Java Using Empty String Literal
One of the most intuitive and widely employed methods for representing an empty character in Java involves using the empty string literal.
The empty string literal in Java is represented simply as ""
. It signifies a string with zero characters, and when used to represent an empty character, it provides a clear and widely accepted way of conveying the absence of character data.
Let’s explore how to create an empty character using the empty string literal:
public class EmptyCharacterExamples {
public static void main(String[] args) {
String emptyString = "";
System.out.println("Empty String: '" + emptyString + "'");
}
}
The expected output should be:
Empty String: ''
While the empty string literal provides a highly readable and conventional means of representing empty characters, it’s essential to consider its appropriateness in the context of the specific application or scenario. This method is most suitable when the absence of a character is represented by an empty character sequence, aligning with common practices and enhancing code readability.
Create an Empty Char in Java Using the Character Class
While the usage of an empty string literal is a widely adopted method for representing empty characters in Java, the Java language offers an alternative avenue through the Character
class.
The Character
class is part of the java.lang
package and serves as a wrapper class for the primitive data type char
. It provides a set of utility methods for working with character data in an object-oriented manner.
Let’s explore how to create an empty character using the Character
class and its '\0'
constant:
public class EmptyCharacterExamples {
public static void main(String[] args) {
char emptyChar = '\0';
System.out.println("Empty Character using Character Class: '" + emptyChar + "'");
}
}
The expected output should be:
Empty Character using Character Class: ''
It is worth noting that while the empty string literal provides a highly readable and conventional means of representing empty characters, the Character
class shines in contexts where precision and control over the primitive char
type are paramount.
This includes low-level programming tasks or scenarios where a more granular level of character manipulation is required.
Create an Empty Char in Java Using the Unicode Null Character
The Unicode null character represented as \u0000
, is a special character in the Unicode character set. Traditionally associated with indicating the end of a string, in Java, it serves a dual purpose by offering an alternative means to represent an empty character.
Let’s explore how to create an empty character using the Unicode null character:
public class EmptyCharacterExamples {
public static void main(String[] args) {
char emptyChar = '\u0000';
System.out.println("Empty Unicode Character: '" + emptyChar + "'");
}
}
The expected output should be:
Empty Unicode Character: ''
While the Unicode null character provides a unique approach to representing empty characters in Java, its usage should be carefully considered. It is less frequently employed than other methods, and its inclusion should be driven by specific project requirements, especially those related to cross-language compatibility and character array manipulation.
Create an Empty Char in Java Using the MIN_VALUE
Constant
The Character
class in Java is a part of the java.lang
package and serves as a wrapper class for the primitive data type char
. It provides methods and constants for working with characters in an object-oriented manner.
To represent an empty character using the Character
wrapper class, we can use the MIN_VALUE
constant. This constant holds the smallest representable value for the char
type, which, in this context, serves as a symbolic representation of an empty character.
Let’s explore how to create an empty character using the Character
class and its MIN_VALUE
constant:
public class EmptyCharacterExamples {
public static void main(String[] args) {
char emptyChar = Character.MIN_VALUE;
System.out.println("Empty Character using Wrapper Class: '" + emptyChar + "'");
}
}
In this example, the MIN_VALUE
constant is assigned to a char
variable, effectively representing an empty character. The result is then displayed, showcasing the empty character representation.
The expected output should be:
Empty Character using Wrapper Class: ''
An alternative approach to creating an empty character involves directly using the null literal. Since the Character
class is derived from the Object
class, assigning null
as an instance effectively represents an empty character.
See the example below:
public class SimpleTesting {
public static void main(String[] args) {
Character ch = null;
System.out.println(ch);
}
}
Output:
null
Create an Empty Char in Java Using Apache Commons Lang Library
Apache Commons Lang is a collection of utility classes and functions for various purposes, including string manipulation, object serialization, and other common tasks. The library is widely adopted in the Java community for its comprehensive set of tools that simplify and enhance everyday programming challenges.
The StringUtils
class within Apache Commons Lang includes a constant named EMPTY
, which represents an empty string. This constant proves useful in scenarios where an empty character representation is required.
Let’s explore how to create an empty character using the StringUtils
class:
import org.apache.commons.lang3.StringUtils;
public class EmptyCharacterExamples {
public static void main(String[] args) {
String emptyString = StringUtils.EMPTY;
System.out.println("Empty Character using Apache Commons Lang: '" + emptyString + "'");
}
}
In this example, we import the StringUtils
class from the Apache Commons Lang library. The StringUtils.EMPTY
constant is then used to obtain an empty string, and we effectively create an empty char.
The expected output should be:
Empty Character using Apache Commons Lang: ''
Conclusion
In Java, representing an empty char poses an interesting challenge due to the inherent nature of the char
data type, which is designed to hold characters and not empty values. Here, we’ve uncovered distinct approaches, each with its own set of advantages and considerations.
-
Empty String Literal: Using
""
provides a simple and readable way to signify the absence of characters, suitable for clean representations of null or undefined states. -
Character Class and
\0
Constant: TheCharacter
class with\0
allows for direct empty character representation, useful for precision in primitivechar
type control. -
Unicode Null Character: Represented as
\u0000
, it offers cross-language compatibility and serves in diverse linguistic projects and character arrays. -
MIN_VALUE
Constant and Null Literal: UsingMIN_VALUE
or assigningnull
provides adaptability in scenarios favoring object-oriented representations. -
Apache Commons Lang Library:
StringUtils
from Apache Commons Lang simplifies code, enhancing productivity and maintaining consistency.
In conclusion, the choice depends on project requirements. Understanding these methods empowers developers to make informed decisions based on application needs.