How to Convert Char to Uppercase/Lowercase in Java
-
Convert a Character to Uppercase/Lowercase Using the
toUpperCase()
/toLowerCase()
Method - Convert a Character to Uppercase/Lowercase Using Binary Operations and Hexadecimal
-
Convert a Character to Uppercase and to Lowercase Using
capitalize()
/lowerCase
Fromcom.apache.commons
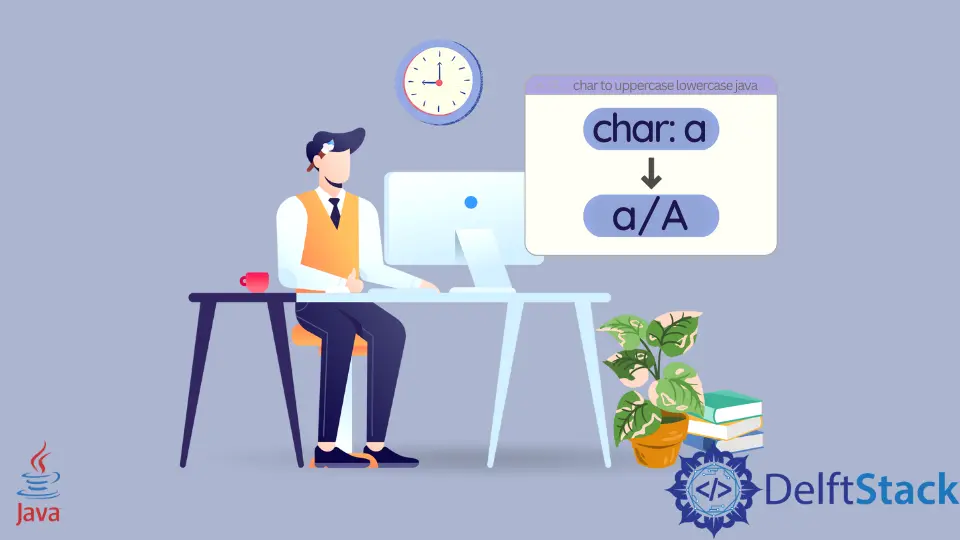
This tutorial introduces methods to convert a character to a lowercase/uppercase character. We have four methods that we will see with examples below.
Convert a Character to Uppercase/Lowercase Using the toUpperCase()
/toLowerCase()
Method
Character
is a wrapper class for char
and provides several methods to manipulate the character like toUpperCase()
and toLowerCase()
. Though these methods cannot handle all the Unicode characters, they can handle the common alphabets.
We have two char
variables, char1
has a lowercase character while char2
have an uppercase character. To convert char1
to an uppercase character, we call the toUpperCase()
static method from the Character
class and pass char1
as an argument. The same goes to convert char2
to lowercase; we call the toLowerCase()
method.
public class CharUpperLowerCase {
public static void main(String[] args) {
char char1, char2;
char1 = 'a';
char2 = 'B';
char char1UpperCase = Character.toUpperCase(char1);
char char2LowerCase = Character.toLowerCase(char2);
System.out.println(char1UpperCase);
System.out.println(char2LowerCase);
}
}
Output:
A
b
Convert a Character to Uppercase/Lowercase Using Binary Operations and Hexadecimal
As every character has an ASCII value and a binary representation, we can perform binary operations. We use the hexadecimal 0x5f
whose binary representation is 1011111 and 0x20
representing 0100000 in binary.
char1
has the character a
and its binary value is 01100001, we append &
the symbol representing binary AND operation and 0x5f
to the character. As we know that 0x5f
has the binary value of 1011111 and when we perform the AND operation that is between 01100001 AND 1011111 we get the value 01000001 that is the binary representation of uppercase A
.
char2
contains the uppercase character B
, and its binary code is 01000010. We append the |
symbol equal to the OR binary operation and 0x20
having the binary value of 0100000. Now 01000010 OR 0100000 will be performed, and the result will be 01100010 that is the same as the lowercase character b
.
Notice that as we use the char
type to hold the characters, the binary values are converted into their representing characters without any casting.
public class CharUpperLowerCase {
public static void main(String[] args) {
char char1, char2;
char1 = 'a' & 0x5f;
char2 = 'B' | 0x20;
System.out.println(char1);
System.out.println(char2);
}
}
Output:
A
b
Convert a Character to Uppercase and to Lowercase Using capitalize()
/lowerCase
From com.apache.commons
In this example, we use the StringUtils
class present in Apache Commons Library. First, we include the library in our project using the following maven dependency.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.11</version>
</dependency>
StringUtils
as the name indicates, provides utility methods to manipulate strings. We have two strings with a single character in each. string1
has a lowercase a
. we use StringUtils.capitalize()
and pass string1
as the argument to convert it to uppercase. string2
has an uppercase B
. We can use StringUtils.lowerCase()
and pass string2
as an argument to convert it to lowercase.
We get the result in the string type, but our goal is to get the value in char
data type, so, we use charAt(0)
to get the single and only character in both the Strings as a char
.
import org.apache.commons.lang3.StringUtils;
public class CharUpperLowerCase {
public static void main(String[] args) {
char char1, char2;
String string1 = "a";
String string2 = "B";
String string1UpperCase = StringUtils.capitalize(string1);
String string2LowerCase = StringUtils.lowerCase(string2);
char1 = string1UpperCase.charAt(0);
char2 = string2LowerCase.charAt(0);
System.out.println(char1);
System.out.println(char2);
}
}
Output:
A
b
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn