How to Initialize Char in Java
- Initialize Char With Empty Char Value in Java
- Initialize Char With NULL Value in Java
- Initialize Char With Default Value in Java
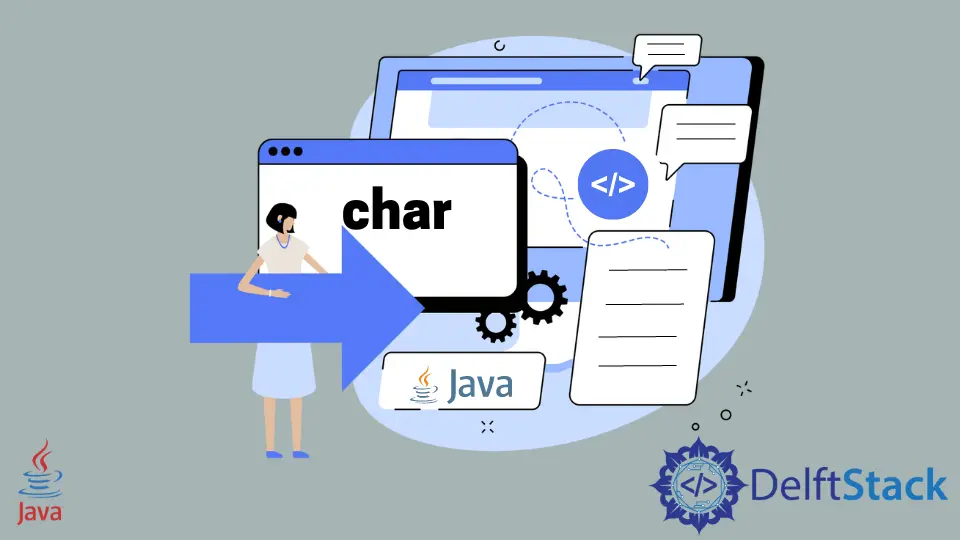
This tutorial introduces how to initialize char and the initial value of char type in Java.
To initialize a char in Java, we can use any char value such as an empty char, or \0
, or even a char value itself. While creating a char variable, we must first understand if the declared variable is local or instance because if the variable is local, then we must initialize it at the time of declaration. If the variable is an instance, we don’t need to worry about initializing because the Java compiler will automatically do this.
The default value of a char value is \0
or \u0000
. Let’s see the examples.
Initialize Char With Empty Char Value in Java
In this example, we created a char variable and initialized it with an empty char value. This value does not make sense but avoids the compilation error since the variable is local. Later we assigned this with a new char value, and the old value was replaced. See the example below.
public class SimpleTesting {
public static void main(String[] args) {
char ch = ' '; // empty char value
System.out.println("char value : " + ch);
// assign new value
ch = 'R';
System.out.println(ch);
}
}
Output:
char value :
R
Initialize Char With NULL Value in Java
In Java, the local variable must be initialized before use. So, it is necessary to provide a value such as \0
that indicates empty or null. Here in the code, we assigned a \0
to the char to initialize it.
public class SimpleTesting {
public static void main(String[] args) {
char ch = '\0'; // equivalent zero char value
System.out.println("char value : " + ch);
// assign new value
ch = 'R';
System.out.println(ch);
}
}
Output:
char value :
R
Initialize Char With Default Value in Java
In Java, each instance variable is set to its default at the time of object creation. The default value of char type is \u0000
, and if we want to initialize a char value with the default value, just create it as an instance variable and let the Java compiler do the rest of the work.
public class SimpleTesting {
char ch; // no initialization
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
System.out.println("char value : " + simpleTesting.ch);
// assign new value
char ch = simpleTesting.ch = 'R';
System.out.println(ch);
}
}
Output:
char value :
R
If you want to see and print the default value, just cast the value, and you will see it is 0
. Yes, the default char value is equal to the 0 integer value.
public class SimpleTesting {
char ch; // no initialization
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
System.out.println("char value : " + simpleTesting.ch);
System.out.println("int equilence char value : " + (int) simpleTesting.ch);
}
}
Output:
char value :
int equilence char value : 0