How to StringUtils in Java
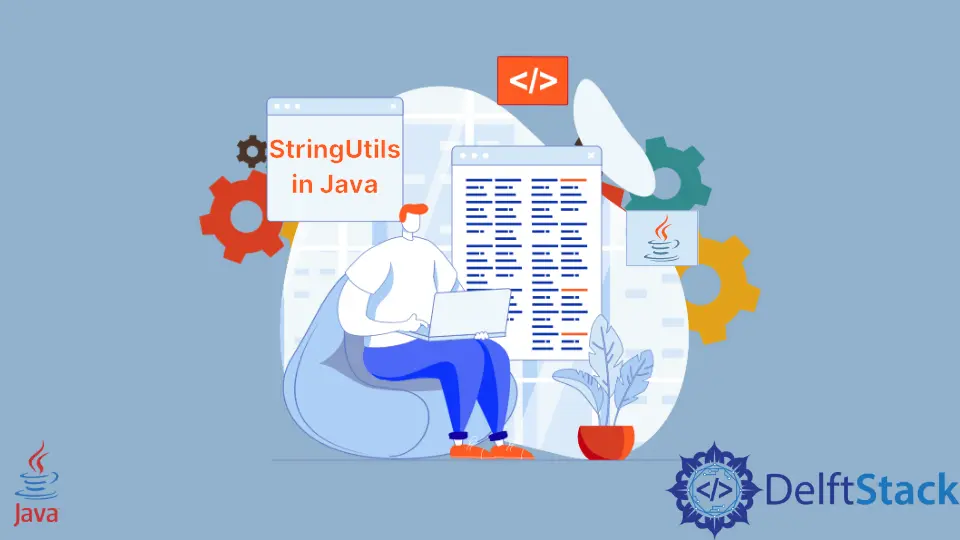
This tutorial introduces what StringUtils
is and how to utilize it in handling String in Java.
StringUtils
is a class used to handle String and provides more utility methods than the Java String
class. This class does not belong to the Java package; instead, it belongs to the Apache Commons Library
.
To use this class in your package, you must first include its JAR file in your project and import the StringUtils
class in your Java source code.
If you are working with the maven project, use the below dependency in the pom.xml
file. It will add the required JARs to your current project.
<!-- https://mvnrepository.com/artifact/org.apache.commons/commons-lang3 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
This class can be found in the org.apache.commons.lang3
package and the signature of this class look like below.
Syntax of StringUtils
Class:
public class StringUtils extends Object
The constructor of the StringUtils
Class:
StringUtils() // no arg constructor
The following are some common operations that StringUtils
supports.
Operation | Description |
---|---|
IsEmpty /IsBlank |
It checks if a String contains text or not. |
Trim /Strip |
It removes the leading and trailing whitespace of the String. |
Equals /Compare |
It compares two strings in a null-safe manner. |
startsWith |
It checks if a String starts with a prefix in a null-safe manner. |
endsWith |
It checks if a String ends with a suffix in a null-safe manner. |
IndexOf /LastIndexOf /Contains |
It returns a null-safe index-of checks. |
IndexOfAny /LastIndexOfAny/IndexOfAnyBut /LastIndexOfAnyBut |
To find index-of any of a set of Strings. |
ContainsOnly /ContainsNone/ContainsAny |
It checks if String contains only/none/any of these characters |
Split /Join |
It splits a String into an array of substrings and vice versa. |
Remove /Delete |
It removes part of a String. |
StringUtils
Example in Java
In this example, we used some standard methods of the StringUtils
class to understand how the class works with the String. This class is similar to the String
class in Java except that it provides more utility methods to work with String.
See the example below.
import org.apache.commons.lang3.StringUtils;
public class SimpleTesting {
public static void main(String[] args) {
// Get abbreviation of string
String val = StringUtils.abbreviate("Delft", 4);
System.out.println(val);
// Capitalize string
val = StringUtils.capitalize("delft");
System.out.println(val);
// Chop string
val = StringUtils.chop("delft");
System.out.println(val);
// Compare two strings
int a = StringUtils.compare(val, val);
System.out.println(a);
// find substring in string
boolean b = StringUtils.contains("delft", "ft");
System.out.println(b);
// Find index of a char in string
int c = StringUtils.indexOf(val, 'f');
System.out.println(c);
// Find last index of a char in string
int d = StringUtils.lastIndexOf("delftstack", 't');
System.out.println(d);
// Lowercase string
val = StringUtils.lowerCase("DELFSTACK");
System.out.println(val);
// Repeat string
val = StringUtils.repeat("DELF", 2);
System.out.println(val);
// Reverse string
val = StringUtils.reverse("Delft");
System.out.println(val);
// Truncate string
val = StringUtils.truncate("Delft", 2);
System.out.println(val);
// Uppercase string
val = StringUtils.upperCase("Delft");
System.out.println(val);
}
}
Output:
D...
Delft
delf
0
true
3
6
delfstack
DELFDELF
tfleD
De
DELFT