How to Get Unicode Characters from its Number in Java
- Get Unicode Char Using Casting in Java
-
Get Unicode Char Using
String.valueOf()
Method in Java -
Get Unicode Char Using
Character.toChars()
Method in Java
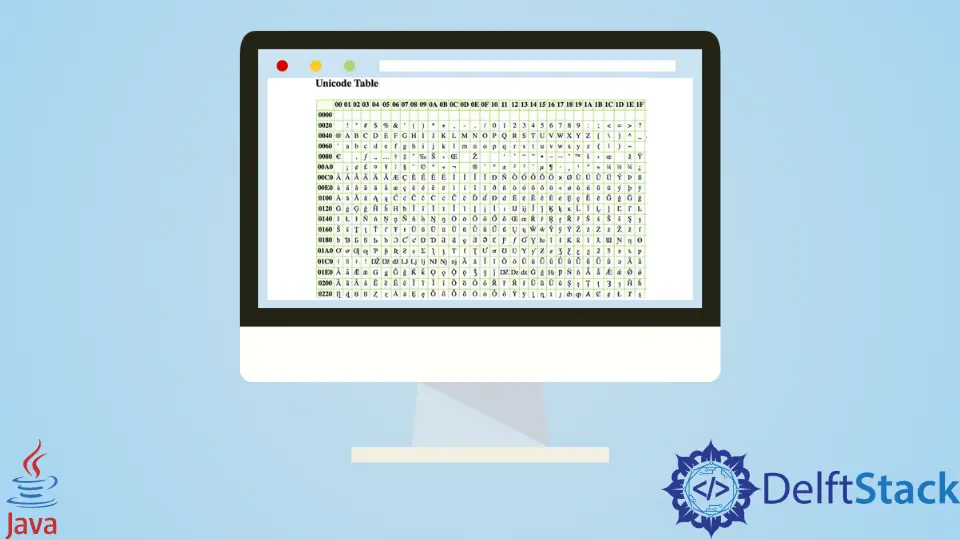
This tutorial introduces how to get the Unicode characters from their number in Java.
Unicode is a character encoding system that assigns a code to every character and symbol in a programming language. Because no other encoding standard covers all languages, Unicode is the only encoding method that ensures you can retrieve or combine data using any combination of languages.
Java strongly supports Unicode chars. This tutorial will discuss how to create a Unicode character from its number.
Get Unicode Char Using Casting in Java
Here, we get a Unicode value by casting to an int value to the char.
We can also get a string from an int representing a Unicode character into a string using the Character.toString()
method. But before we can apply this method to the code, we first need to convert the code into char explicitly.
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
int code = 0x2202;
System.out.println((char) code);
String code_str = Character.toString((char) code);
System.out.println(code_str);
}
}
Output:
∂
∂
The Character.toString()
method is an overloaded method, which takes the codepoint as the argument and returns the string representation of the specified codepoint. Look at the code below for another example.
public class SimpleTesting {
public static void main(String args[]) {
int code = 0x13434;
String code_str = Character.toString((char) code);
System.out.println(code_str);
}
}
Output:
㐴
\u
bits) are in HEX, we preceded the code with a 0x
extension.Get Unicode Char Using String.valueOf()
Method in Java
In this example, we used the String.valueOf()
method that takes a char type as an argument and returns a string. After casting, we first get a char and then pass it to the valueOf()
method.
See the example below.
public class SimpleTesting {
public static void main(String args[]) {
int code = 0x13434;
char ch_code = (char) code;
String code_str = String.valueOf(ch_code);
System.out.println(code_str);
}
}
Output:
㐴
Let’s see one more example to get Unicode char.
public class SimpleTesting {
public static void main(String args[]) {
int code = 0x2202;
char ch_code = (char) code;
String code_str = String.valueOf(ch_code);
System.out.println(code_str);
}
}
Output:
∂
Get Unicode Char Using Character.toChars()
Method in Java
We used the toChar()
method that returns a char in this example.
To convert code into Unicode, we first need to convert it into a hexadecimal integer using the parseInt()
and passing 16
as the radix. After this, we used the Character.toChars()
method to convert the integer into a char data type.
We finally call the String.valueOf()
method to produce a string. Look at the code example below:
public class SimpleTesting {
public static void main(String args[]) {
String code = "2202";
String code_str = String.valueOf(Character.toChars(Integer.parseInt(code, 16)));
System.out.println(code_str);
}
}
Output:
∂
Let’s see one more example to get Unicode char.
public class SimpleTesting {
public static void main(String args[]) {
String code = "1434";
String code_str = String.valueOf(Character.toChars(Integer.parseInt(code, 16)));
System.out.println(code_str);
}
}
Output:
ᐴ
This method is essentially like the previous one, except that we use the toChars()
method to convert the integer into characters instead of explicitly typecasting it.