Character Ignore Case in Java
- Character Ignore Case in Java
-
Use
toLowerCase
andtoUpperCase
to Ignore Character Case in Java -
Use
isLowerCase
andisUpperCase
to Ignore Character Case in Java
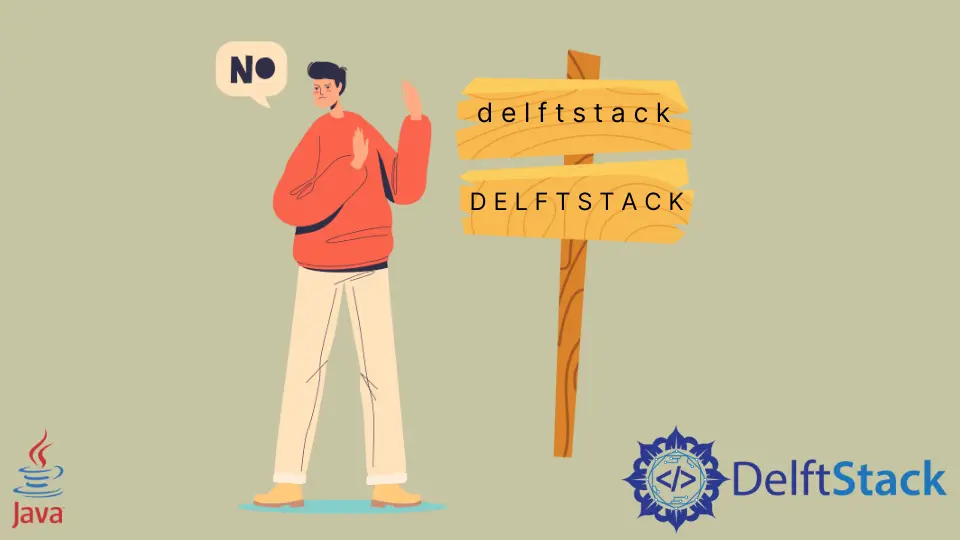
The strings are compared based on case sensitivity, but sometimes we need to ignore the case sensitivity for some characters.
This tutorial demonstrates how to ignore cases for characters in Java.
Character Ignore Case in Java
The Character
class of Java API can work with the case sensitivity of characters in Java. It has functions like toLowerCase
, toUpperCase
, isUppercase
, and isLowerCase
, which can compare characters in Java.
Use toLowerCase
and toUpperCase
to Ignore Character Case in Java
The toLowerCase
and toUpperCase
convert the characters from upper case to lower case and lower case to upper case. These two methods can be used to compare two characters while ignoring the case.
See example:
package delftstack;
public class Char_Ignore {
public static void main(String[] args) {
String Demo_String1 = "DELFTSTACK";
String Demo_String2 = "delftstack";
int count = Demo_String1.length();
for (int i = 0; i < count; i++) {
if (Character.toLowerCase(Demo_String1.charAt(i)) == Demo_String2.charAt(i)) {
System.out.print(Character.toLowerCase(Demo_String1.charAt(i)) + "\s");
}
}
System.out.print("\n");
for (int i = 0; i < count; i++) {
if (Demo_String1.charAt(i) == Character.toUpperCase(Demo_String2.charAt(i))) {
System.out.print(Character.toUpperCase(Demo_String2.charAt(i)) + "\s");
}
}
System.out.print("\n");
// Characters of first string are not equal to second string, to check:
System.out.print(Demo_String1.charAt(1) == Demo_String2.charAt(1)); // Returns False
}
}
The code above tries to compare two strings in two loops. In both loops, one string is the original, and the second is the case converted to make them equal so we can ignore the case.
It also shows that both strings are not equal by comparing the characters. See output:
d e l f t s t a c k
D E L F T S T A C K
false
Use isLowerCase
and isUpperCase
to Ignore Character Case in Java
The isLowerCase
will ignore all uppercase characters in the string, and the isUpperCase
will ignore all the lower case characters in the string. See example:
package delftstack;
public class Char_Ignore {
public static void main(String[] args) {
String Demo_String1 = "DELFTstack";
String Demo_String2 = "delftSTACK";
int count = Demo_String1.length();
for (int i = 0; i < count; i++) {
if (Character.isUpperCase(Demo_String1.charAt(i))) {
System.out.print(Character.toLowerCase(Demo_String1.charAt(i)) + "\s");
}
}
System.out.print("\n");
for (int i = 0; i < count; i++) {
if (Character.isLowerCase(Demo_String1.charAt(i))) {
System.out.print(Character.toUpperCase(Demo_String2.charAt(i)) + "\s");
}
}
}
}
The code above will print the two strings, first by ignoring the uppercase characters and second by ignoring the lower case letters. See output:
d e l f t
S T A C K
These methods can be used for both strings and single characters.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook