List of Ints in Java
- Create a List of Ints in Java
-
Create List of Ints Using the
Arrays
Class in Java -
Create List of Ints Using the
MutableIntList
Class in Java
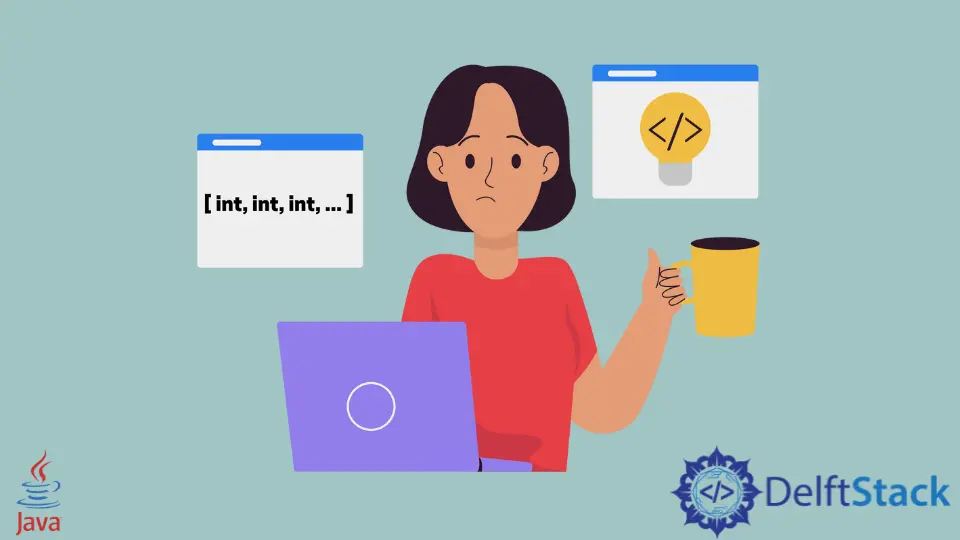
This tutorial introduces how to create a list of integer values in Java.
The List
is an interface in Java that is used to store data. It is dynamic in size. ArrayList is the implementation class of this List
and can be used to create a list since List
belongs to a collection framework that works on objects only. So, the list only stores objects.
This article will teach us how to create an integer list that will store primitive int data type values. In the Java collection framework, primitive values automatically get converted to their object by using wrapper classes. This process is called autoboxing.
Let’s understand by using some examples.
Create a List of Ints in Java
In this example, we used the ArrayList
class to create a list of ints. Here, we specified the ArrayList
type as Integer but passed only int values. Notice, Java takes care of this auto conversion and reduces code. See the example below.
import java.util.ArrayList;
import java.util.List;
public class SimpleTesting {
List<Integer> ScoreList = new ArrayList<Integer>();
public void AddScore(int score) {
ScoreList.add(score);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.AddScore(95);
simpleTesting.AddScore(85);
simpleTesting.AddScore(93);
simpleTesting.AddScore(91);
System.out.println("Students Scores: ");
System.out.println(simpleTesting.ScoreList);
}
}
Output:
Students Scores:
[95, 85, 93, 91]
Create List of Ints Using the Arrays
Class in Java
Here, we used the asList()
method of the Arrays
class to create a list of integers. If you have an array of integers and want to get a list, use the asList()
method. See the example below.
import java.util.Arrays;
import java.util.List;
public class SimpleTesting {
List<Integer> ScoreList = Arrays.asList(95, 85, 93, 91);
public void AddScore(int score) {
ScoreList.add(score);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
System.out.println("Students Scores: ");
System.out.println(simpleTesting.ScoreList);
}
}
Output:
Students Scores:
[95, 85, 93, 91]
Create List of Ints Using the MutableIntList
Class in Java
If you are working with the eclipse collection library, then use the MutableIntList
class to create a list of ints. Here, we used the empty()
method to create an empty list and then the add()
method to add elements. It creates a mutable list of integers that can be changed accordingly. See the example below.
import org.eclipse.collections.api.list.primitive.MutableIntList;
import org.eclipse.collections.impl.factory.primitive.IntLists;
public class SimpleTesting {
MutableIntList ScoreList = IntLists.mutable.empty();
public void AddScore(int score) {
ScoreList.add(score);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.AddScore(95);
simpleTesting.AddScore(85);
simpleTesting.AddScore(93);
simpleTesting.AddScore(91);
System.out.println("Students Scores: ");
System.out.println(simpleTesting.ScoreList);
}
}
Output:
Students Scores:
[95, 85, 93, 91]
Related Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- Differences Between List and Arraylist in Java
- List vs. Array in Java