Differences Between List and Arraylist in Java
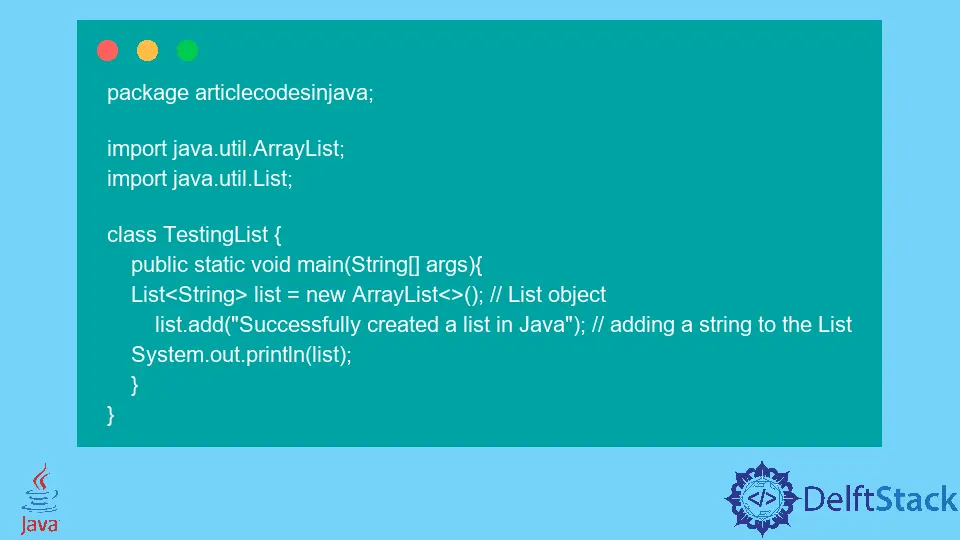
ArrayList
and List
are members of the Collection framework in Java, where ArrayList
is a class, and List
is an interface. We can perform different operations like deletion, insertion, sorting, manipulation, and searching on a group of objects with the help of the Collection framework.
Collection Framework
The Collection framework in Java has the following set of interfaces and classes:
Interface:
- Set
- Queue
- List
- Deque
Classes:
- ArrayList
- Vector
- LinkedList
- HashSet
- TreeSet
- LinkedHashSet
- PriorityQueue
The Collection framework has different classes and interfaces, but this article will focus only on List
and ArrayList
, so let’s discuss each with an example.
List in Java
In Java, List
belongs to the Collection framework. It is an ordered group of objects represented as a single unit.
We can store heterogeneous types of data in a list and access the data based on the indexes of the elements of the list. You can perform different operations like delete, insert, update and search on a list in Java.
The List
interface can be found in the java.util
package. It inherits the Collection interface.
It implements the LinkedList
, Stack
, ArrayList
, and Vector
classes. We can iterate over a list in both backward and forward directions.
package articlecodesinjava;
import java.util.ArrayList;
import java.util.List;
class TestingList {
public static void main(String[] args) {
List<String> list = new ArrayList<>(); // List object
list.add("Successfully created a list in Java"); // adding a string to the List
System.out.println(list);
}
}
Output:
[Successfully created a list in Java] //The output is in List form
Built-in List Methods in Java
In Java, a list has multiple methods specific to it. These are used for different purposes; some of the most popular and frequent are the following:
package articlecodesinjava;
import java.util.*;
class TestingList {
public static void main(String[] args) {
List<Object> list = new ArrayList<>();
list.add(1); // Adding values in list
list.add(2);
System.out.println("List after adding values " + list);
list.removeAll(list); // return and empty list, all values are erased
System.out.println("List after the removeAll method " + list);
list.add("3"); // Adding values in list
list.add("4");
System.out.println("New list " + list);
System.out.println("The size of the List " + list.size());
System.out.println("Return a value from the list a 1 position " + list.get(1));
System.out.println("Removing value at position 1 = " + list.remove(1));
System.out.println("Final list" + list);
}
}
Output:
List after adding values [1, 2]
List after the removeAll method []
New list [3, 4]
The size of the List 2
Return a value from the list a 1 position 4
Removing value at position 1 = 4
Final list[3]
First, we have imported the package java.util.*
to access List
and ArrayList
in the above program. Then we created a list and, with the object of the list, we populated some values first with the add()
, next we removed all the data from the list with removeAll()
it returned an empty list.
Further, we have again added some values in the list to perform some other operations on the list. We found the size of the list with the size()
and displayed a value at position/index number 1 with get(1)
, and at the end, we have removed a value at index number 1 with remove(1)
.
The list has tons of built-in methods. You can read more on the official site of Java.
ArrayList in Java
In Java, ArrayList
is an advanced version of the traditional array. The traditional array has a fixed size, so it wasn’t possible to grow and shrink the size of the array.
Hence, the Collection framework comes up with a dynamic array known as ArrayList
. The ArrayList
class is a dynamic array that can expand and shrink its size upon adding and removing elements from the list.
It inherits the AbstractList
class and implements the List
interface to use all the List
interface methods. The elements can be accessed through the indexes of the elements of the list.
ArrayList
does not support primitive data types like char, int, float, etc. But we use the wrapper class for such cases.
package articlecodesinjava;
import java.util.ArrayList;
class TestingArrayList {
public static void main(String[] args) {
ArrayList<String> arrayList = new ArrayList<>(); // ArrayList object
arrayList.add("Successfully created an arrayList in Java"); // adding a string to the ArrayList
System.out.println(arrayList);
}
}
Output:
[Successfully created an arrayList in Java]
Built-in ArrayList Methods in Java
ArrayList
extends AbstractList
, which implements List
, so we can use all the methods of List
in ArrayList
.
See the example below. We will use all the above programs with the same methods in ArrayList
.
Code example:
package articlecodesinjava;
import java.util.*;
class TestingArrayList {
public static void main(String[] args) {
ArrayList arrayList = new ArrayList<>();
arrayList.add(1); // Adding values in ArrayList
arrayList.add(2);
System.out.println("ArrayList after adding values " + arrayList);
arrayList.removeAll(arrayList); // return and empty ArrayList, all values are erased
System.out.println("ArrayList after the removeAll method " + arrayList);
arrayList.add("3"); // Adding values in ArrayList
arrayList.add("4");
arrayList.add(0, 5);
System.out.println("New ArrayList " + arrayList);
System.out.println("The size of the ArrayList " + arrayList.size());
System.out.println("Return a value from the ArrayList at 1 position " + arrayList.get(1));
System.out.println("Removing value at position 1 = " + arrayList.remove(1));
System.out.println("Final ArrayList" + arrayList);
}
}
Output:
ArrayList after adding values [1, 2]
ArrayList after the removeAll method []
New ArrayList [5, 3, 4]
The size of the ArrayList 3
Return a value from the ArrayList at 1 position 3
Removing value at position 1 = 3
Final ArrayList[5, 4]
Differences Between List and ArrayList in Java
Below are some of the key differences between the List
and ArrayList
in Java:
List
List
is an interface.- The
List
interface extends the Collection framework. - It can’t be instantiated.
- It creates a list of objects.
Lists
are faster in manipulating objects.
ArrayList
ArrayList
is a standard Collection class.ArrayList
extendsAbstractList
, which implementsList
interfaces.- It can be instantiated.
- it creates an array of objects.
ArrayLists
are not as fast in manipulating objects compared to List.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Java List
- How to Split a List Into Chunks in Java
- How to Get First Element From List in Java
- How to Find the Index of an Element in a List Using Java
- How to Filter List in Java
- List vs. Array in Java